Enhancing JSON Attribute Handling in Laravel with AsArrayObject and AsCollection Casts
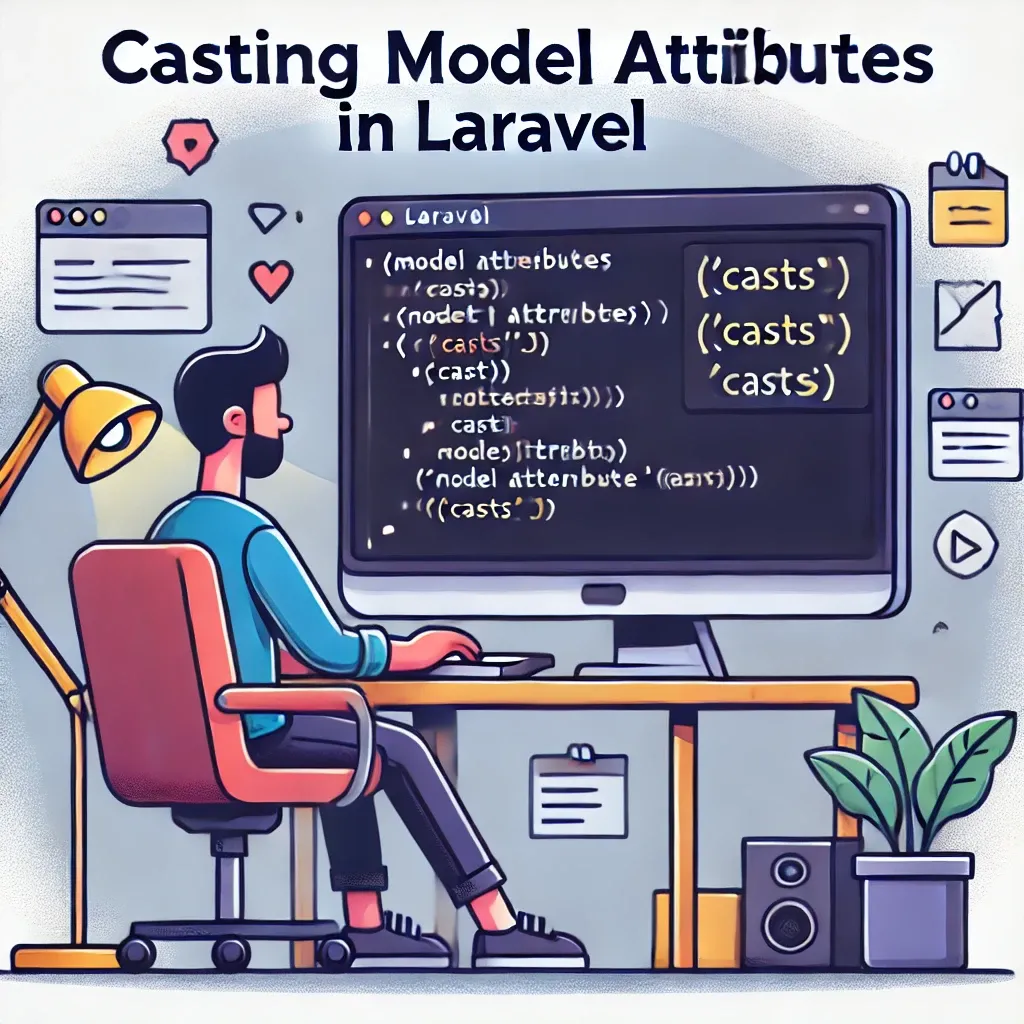
Laravel's Eloquent ORM is renowned for its powerful features, including its robust casting system. When working with JSON attributes, two casts stand out for their utility: AsArrayObject
and AsCollection
. Let's explore how these casts can elevate your Laravel development experience.
The Problem with Standard Array Casts
When dealing with JSON columns in your database, Laravel's default array cast is often sufficient. However, it falls short when you need to modify nested data. Consider this scenario:
$user = User::find(1);
$user->options['key'] = $value; // This triggers a PHP error
This limitation can be frustrating when working with complex, nested JSON structures.
Enter AsArrayObject and AsCollection
Laravel introduces two powerful casts to address this issue:
AsArrayObject Cast
The AsArrayObject
cast converts your JSON attribute to an ArrayObject
instance:
use Illuminate\Database\Eloquent\Casts\AsArrayObject;
class User extends Model
{
protected $casts = [
'options' => AsArrayObject::class,
];
}
Now, you can manipulate nested data without encountering PHP errors:
$user = User::find(1);
$user->options['key'] = $value;
$user->save();
AsCollection Cast
If you prefer working with Laravel's Collection class, the AsCollection
cast is your ally:
use Illuminate\Database\Eloquent\Casts\AsCollection;
class User extends Model
{
protected $casts = [
'items' => AsCollection::class,
];
}
This gives you access to all of Laravel's powerful Collection methods:
$user = User::find(1);
$user->items->push('new item');
$user->save();
Real-World Application
Imagine you're building an e-commerce platform where users have a 'preferences' JSON column storing various settings:
class User extends Model
{
protected $casts = [
'preferences' => AsArrayObject::class,
];
}
Updating user preferences becomes intuitive and straightforward:
$user->preferences['currency'] = 'EUR';
$user->preferences['notifications']['email'] = true;
$user->save();
Conclusion
By leveraging AsArrayObject
or AsCollection
casts, you can significantly streamline your code when working with complex JSON attributes in Laravel. These casts provide a more intuitive and error-resistant approach to handling nested data structures, enhancing the robustness and maintainability of your Laravel applications.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!