Enhancing Frontend Interactivity with Laravel Blade Fragments
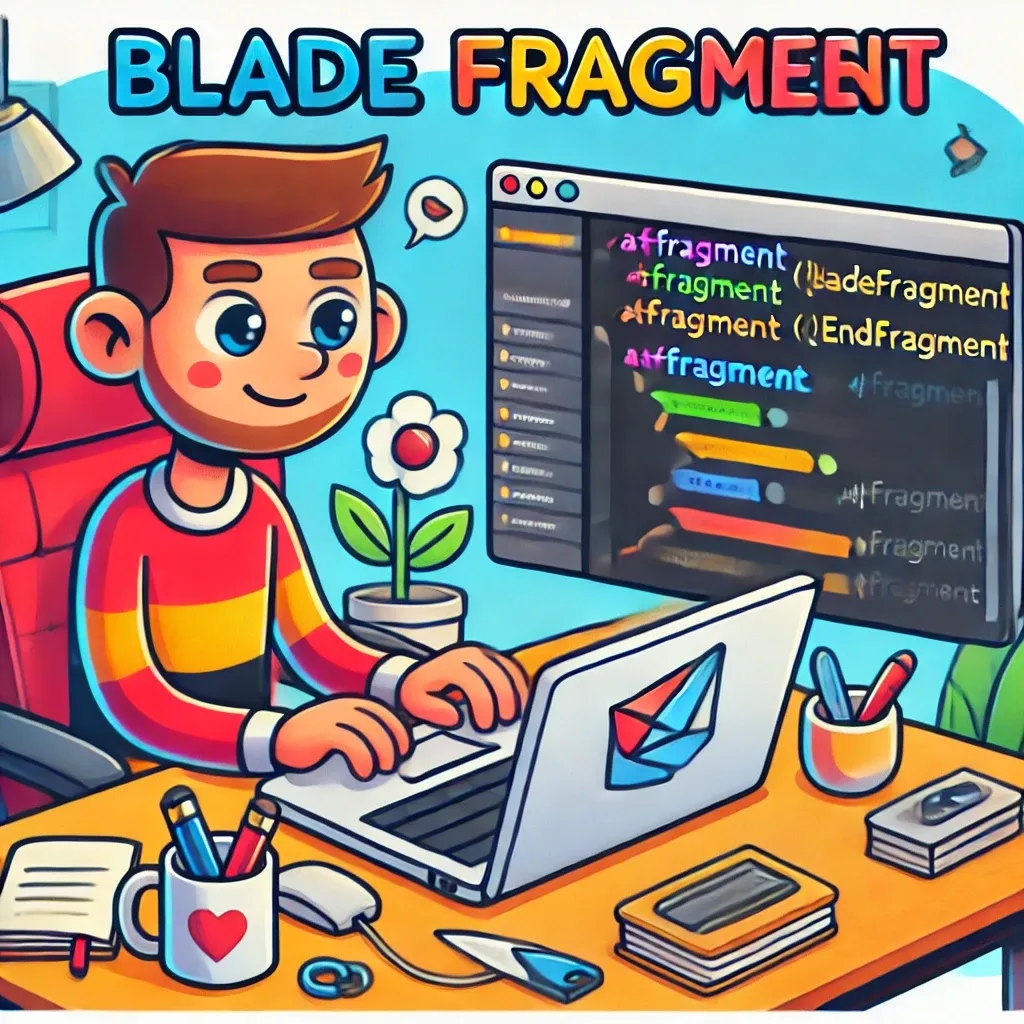
In modern web development, creating interactive user interfaces often requires updating only portions of a page. Laravel's Blade Fragments feature provides an elegant solution for this, allowing you to return specific sections of your Blade templates in response to requests. This is particularly useful when working with frontend frameworks like Turbo or htmx.
Understanding Blade Fragments
Blade Fragments allow you to define and return specific portions of a Blade template. This is incredibly useful for partial page updates or when you need to return only a specific part of a view in response to an AJAX request.
Basic Usage of Blade Fragments
To define a fragment in your Blade template, you use the @fragment
directive:
@fragment('user-list')
<ul>
@foreach ($users as $user)
<li>{{ $user->name }}</li>
@endforeach
</ul>
@endfragment
To return only this fragment from your controller, you can use the fragment
method:
return view('dashboard', ['users' => $users])->fragment('user-list');
This will return only the content within the specified fragment, rather than the entire view.
Conditional Fragments
Laravel also provides a fragmentIf
method, allowing you to conditionally return a fragment:
return view('dashboard', ['users' => $users])
->fragmentIf($request->hasHeader('HX-Request'), 'user-list');
This is particularly useful when working with htmx, as it allows you to return the full page for normal requests and only the fragment for htmx requests.
Multiple Fragments
You can also return multiple fragments in a single response:
return view('dashboard', ['users' => $users])
->fragments(['user-list', 'comment-list']);
Real-World Example: Dynamic Comment Section
Let's consider a blog post with a dynamically updating comment section:
// In your Blade template (show-post.blade.php)
<div id="post-content">
<h1>{{ $post->title }}</h1>
<p>{{ $post->content }}</p>
</div>
@fragment('comments')
<div id="comments">
@foreach ($post->comments as $comment)
<div class="comment">
<p>{{ $comment->content }}</p>
<small>By {{ $comment->user->name }}</small>
</div>
@endforeach
</div>
@endfragment
// In your controller
public function addComment(Request $request, Post $post)
{
$comment = $post->comments()->create([
'content' => $request->content,
'user_id' => auth()->id(),
]);
if ($request->hasHeader('HX-Request')) {
return view('show-post', ['post' => $post->fresh()])
->fragment('comments');
}
return back();
}
In this example, when a new comment is added via an htmx request, only the comments fragment is returned and updated on the page, providing a smooth, dynamic user experience.
Blade Fragments offer a powerful way to create more interactive, dynamic web applications with Laravel. By allowing you to return specific portions of your views, they enable seamless integration with modern frontend technologies while keeping your backend logic clean and Laravel-centric. Whether you're building a complex single-page application or simply want to add some dynamic elements to your Laravel app, Blade Fragments provide the flexibility and performance you need.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!