Enhancing Form Validation with Laravel's prohibited_if Rule
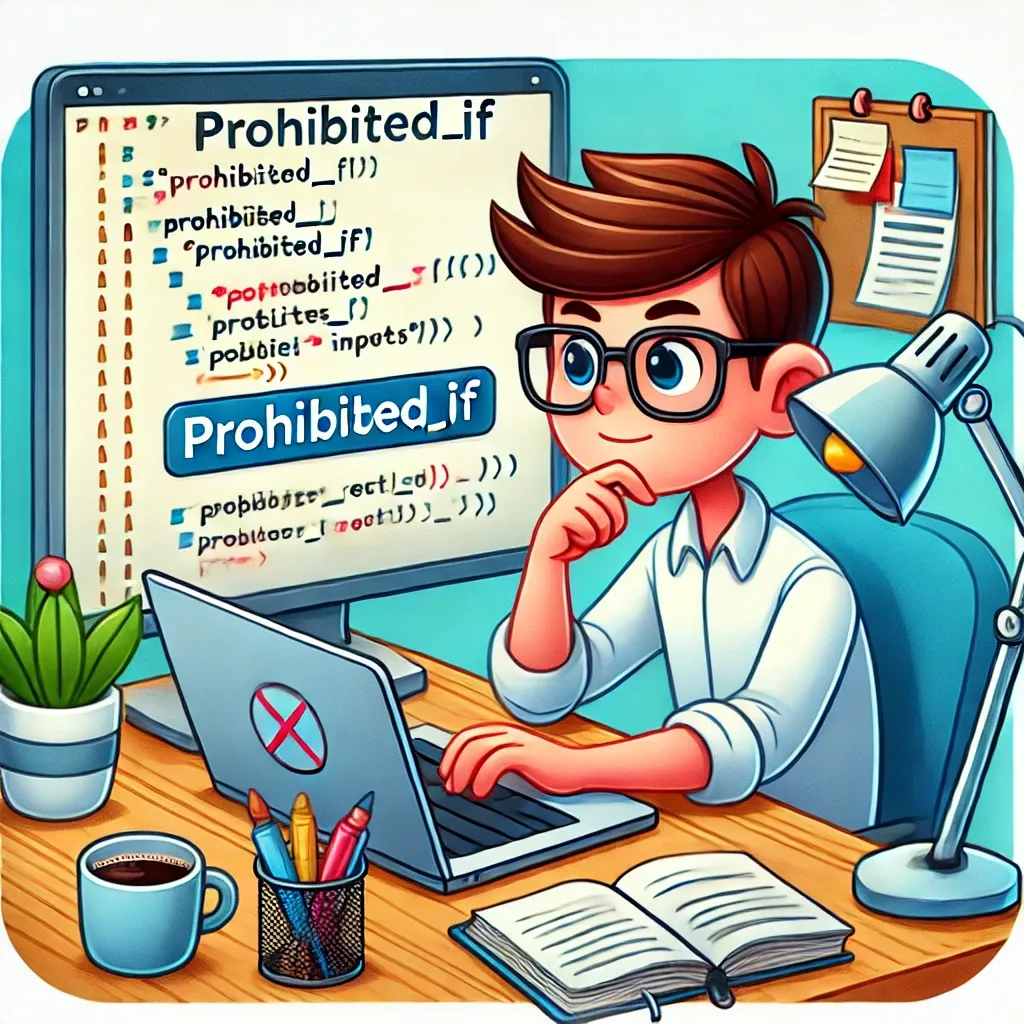
In the world of web development, form validation is crucial for maintaining data integrity and providing a smooth user experience. Laravel, known for its elegant solutions, offers a powerful validation rule called prohibited_if
. This rule allows you to conditionally prevent field input based on the values of other fields. Let's dive into how you can leverage this feature in your Laravel applications.
Understanding prohibited_if
The prohibited_if
rule in Laravel allows you to specify that a field must be empty or not present when certain conditions are met. This is particularly useful for creating dynamic forms where some fields should be empty based on the values of others.
Basic Usage
Here's a simple example of how to use prohibited_if
:
$validator = Validator::make($request->all(), [
'company_name' => 'prohibited_if:is_freelancer,true',
'is_freelancer' => 'boolean',
]);
In this example, the company_name
field must be empty or not present if is_freelancer
is true.
Advanced Usage with Rule Object
For more complex scenarios, you can use the Rule::prohibitedIf
method:
use Illuminate\Validation\Rule;
$validator = Validator::make($request->all(), [
'role_id' => Rule::prohibitedIf(fn() => $request->user()->is_admin),
]);
This allows you to use a closure for more dynamic condition checking.
Real-World Example
Let's consider a registration form for a job platform where users can sign up as either individuals or companies:
public function rules()
{
return [
'account_type' => 'required|in:individual,company',
'name' => 'required|string|max:255',
'company_name' => [
'prohibited_if:account_type,individual',
'required_if:account_type,company',
'string',
'max:255',
],
'company_size' => [
'prohibited_if:account_type,individual',
'required_if:account_type,company',
'integer',
'min:1',
],
];
}
In this example:
- If the user selects 'individual' as the account type,
company_name
andcompany_size
must be empty or not present. - If the user selects 'company', these fields become required.
Combining with Other Rules
You can combine prohibited_if
with other validation rules for more comprehensive validation:
'social_security_number' => [
'prohibited_if:country,not,US',
'required_if:country,US',
'string',
'size:9',
],
This ensures that a social security number is only provided (and required) for US-based users.
Error Messages
Laravel automatically generates error messages for prohibited_if
validations, but you can customize them:
$messages = [
'company_name.prohibited_if' => 'Company name should not be provided for individual accounts.',
];
$validator = Validator::make($request->all(), $rules, $messages);
The prohibited_if
validation rule in Laravel provides a powerful way to create dynamic, context-aware forms. By leveraging this feature, you can ensure that your forms adapt to user input, improving data integrity and user experience. Whether you're building complex registration forms, conditional surveys, or any interface with interdependent fields, prohibited_if
offers the flexibility you need to create robust, user-friendly forms in your Laravel applications.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!