Enhancing Concurrency Control with Laravel's Session Blocking
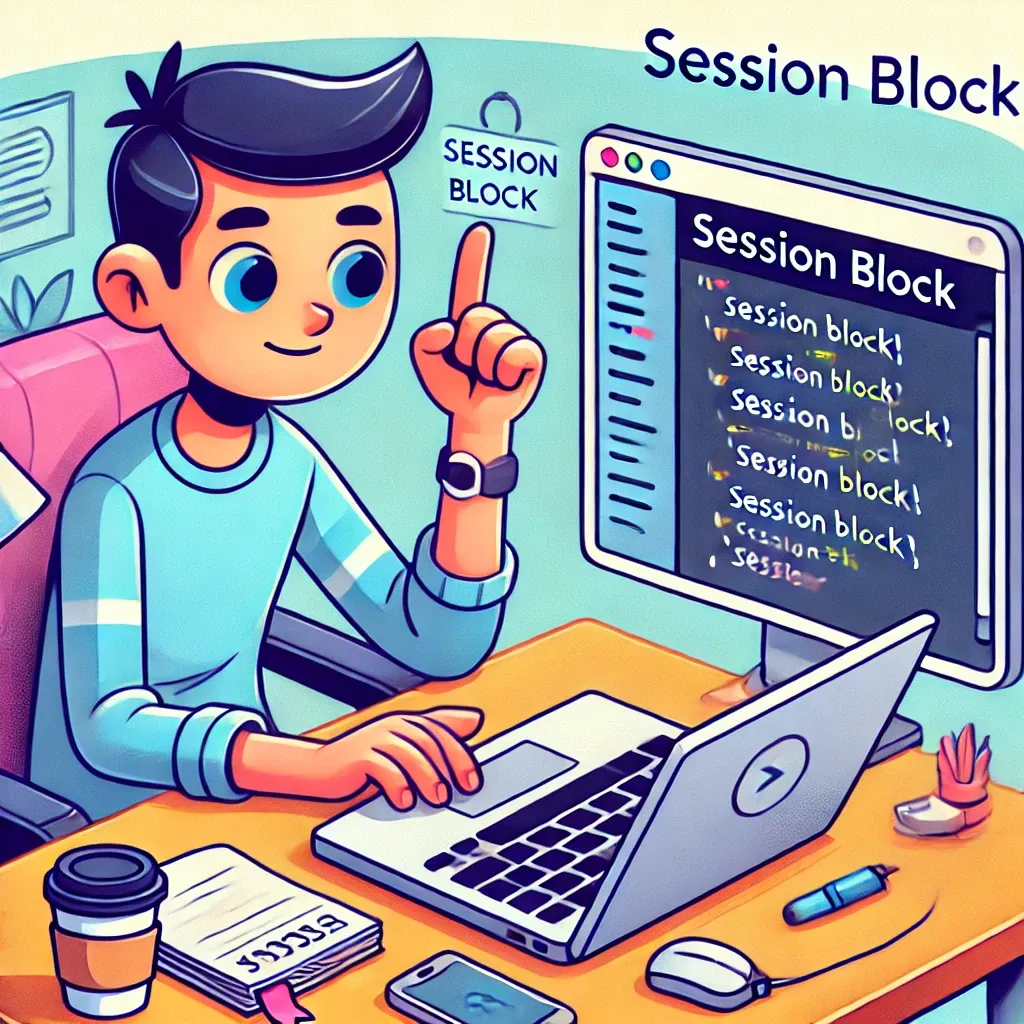
In the world of web development, managing concurrent requests can be challenging, especially when dealing with shared resources like session data. Laravel offers a powerful feature called session blocking to help developers handle these scenarios effectively. Let's dive into how you can leverage session blocking in your Laravel applications to prevent data loss and improve concurrency control.
Understanding Session Blocking
Session blocking in Laravel allows you to limit concurrent requests for a given session. This is particularly useful in scenarios where multiple requests from the same session could lead to data inconsistencies or race conditions.
Prerequisites
To use session blocking, your application must meet the following requirements:
- Use a cache driver that supports atomic locks (e.g., memcached, dynamodb, redis, database, file, or array).
- Not use the cookie session driver.
Implementing Session Blocking
To implement session blocking, you can use the block
method on your route definitions. Here's a basic example:
Route::post('/profile', function () {
// Profile update logic
})->block($lockSeconds = 10, $waitSeconds = 10);
Route::post('/order', function () {
// Order processing logic
})->block($lockSeconds = 10, $waitSeconds = 10);
In this example:
$lockSeconds
(10) is the maximum number of seconds the session lock should be held.$waitSeconds
(10) is the number of seconds a request should wait to obtain a session lock.
How It Works
When a request comes in, Laravel attempts to acquire a lock for the session. If successful, the request proceeds. If not, it waits up to $waitSeconds
to acquire the lock. If it can't acquire the lock within that time, Laravel throws an Illuminate\Contracts\Cache\LockTimeoutException
.
Real-World Example: Handling Concurrent Order Submissions
Let's consider a scenario where we want to prevent double-submission of orders:
Route::post('/submit-order', function (Request $request) {
return DB::transaction(function () use ($request) {
$order = Order::create($request->all());
// Process payment
$payment = Payment::process($order);
if ($payment->successful()) {
$order->markAsPaid();
return response()->json(['message' => 'Order placed successfully'], 201);
} else {
throw new PaymentFailedException('Payment processing failed');
}
});
})->block(5, 10);
In this example:
- We use a database transaction to ensure atomicity.
- Session blocking prevents concurrent order submissions from the same session.
- If a concurrent request occurs, it will wait up to 10 seconds before failing.
Laravel's session blocking feature provides a powerful tool for managing concurrency in your web applications. By implementing session blocking strategically, you can prevent data inconsistencies, improve the reliability of critical operations, and enhance the overall robustness of your Laravel applications. Remember to use this feature judiciously and always consider the impact on user experience when implementing concurrency controls.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!