Enhancing Component Communication in Laravel Livewire with $listeners
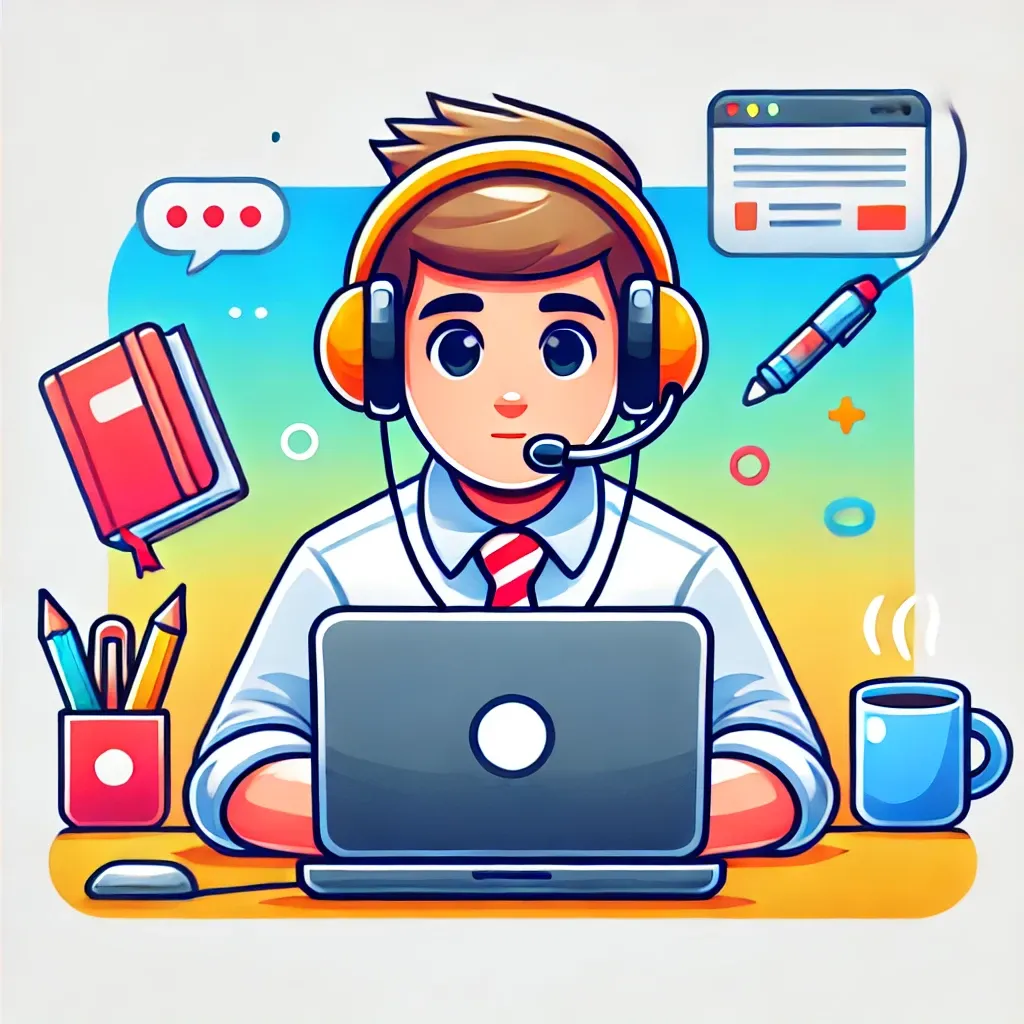
In the world of dynamic, reactive interfaces, efficient component communication is crucial. Laravel Livewire offers a powerful feature to facilitate this: the $listeners property. This blog post will explore how to use $listeners to create interactive, decoupled components that can react to events from other parts of your application.
Understanding $listeners
The $listeners property in Livewire allows a component to subscribe to events. When these events are dispatched from anywhere in your Livewire ecosystem, the component can react accordingly. This creates a flexible, event-driven architecture within your Livewire applications.
Basic Usage of $listeners
Let's look at a basic example of how to use $listeners:
class ShoppingCart extends Component
{
public $items = [];
protected $listeners = ['itemAdded' => 'addItem'];
public function addItem($item)
{
$this->items[] = $item;
}
public function render()
{
return view('livewire.shopping-cart');
}
}
In this example, the ShoppingCart component is listening for an 'itemAdded' event. When this event is dispatched, it will call the addItem method.
Dispatching Events
To make this work, we need another component to dispatch the event:
class ProductList extends Component
{
public function addToCart($product)
{
// Add to cart logic...
$this->dispatch('itemAdded', $product);
}
public function render()
{
return view('livewire.product-list');
}
}
The ProductList component dispatches the 'itemAdded' event when a product is added to the cart.
Advanced Usage
Multiple Listeners
You can listen for multiple events in a single component:
protected $listeners = [
'itemAdded' => 'addItem',
'itemRemoved' => 'removeItem',
'cartCleared' => 'clearCart'
];
Dynamic Listeners
Livewire also allows for dynamic listeners:
public function getListeners()
{
return [
"echo-private:orders.{$this->orderId},OrderShipped" => 'handleShippedOrder',
];
}
This is particularly useful when working with dynamic channels in Laravel Echo.
Real-World Example: Chat Application
Let's consider a more complex scenario of a real-time chat application:
class ChatRoom extends Component
{
public $messages = [];
public $roomId;
protected $listeners = ['messageReceived' => 'addMessage'];
public function mount($roomId)
{
$this->roomId = $roomId;
$this->messages = Message::where('room_id', $roomId)->latest()->take(50)->get()->reverse();
}
public function addMessage($message)
{
if ($message['room_id'] == $this->roomId) {
$this->messages[] = $message;
}
}
public function sendMessage($content)
{
$message = auth()->user()->messages()->create([
'content' => $content,
'room_id' => $this->roomId
]);
$this->dispatch('messageReceived', $message->toArray())->to('chat-room');
}
public function render()
{
return view('livewire.chat-room');
}
}
In this example:
- The ChatRoom component listens for 'messageReceived' events.
- When a new message is sent, it's broadcasted to all instances of the ChatRoom component.
- The addMessage method checks if the received message belongs to the current room before adding it to the messages array.
Best Practices
- Keep Components Decoupled: Use events for communication between components that don't have a direct parent-child relationship.
- Use Namespaced Events: For larger applications, consider namespacing your events (e.g., 'cart.item.added') to avoid conflicts.
- Be Mindful of Performance: While powerful, overuse of events can lead to performance issues. Use them judiciously.
- Combine with dispatch(): Always use the dispatch() method to emit events that listeners are waiting for.
- Testing: When testing components that use $listeners, make sure to test both the dispatching and receiving of events.
Conclusion
The $listeners property in Laravel Livewire provides a powerful mechanism for component communication. By leveraging this feature, you can create more interactive, responsive, and decoupled interfaces. Whether you're building a shopping cart, a chat application, or any other dynamic interface, $listeners offers the flexibility and reactivity you need to craft engaging user experiences. Remember, effective use of events can lead to cleaner, more maintainable code by reducing direct dependencies between components.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!