Enhancing Command Execution with Laravel's Isolatable Interface
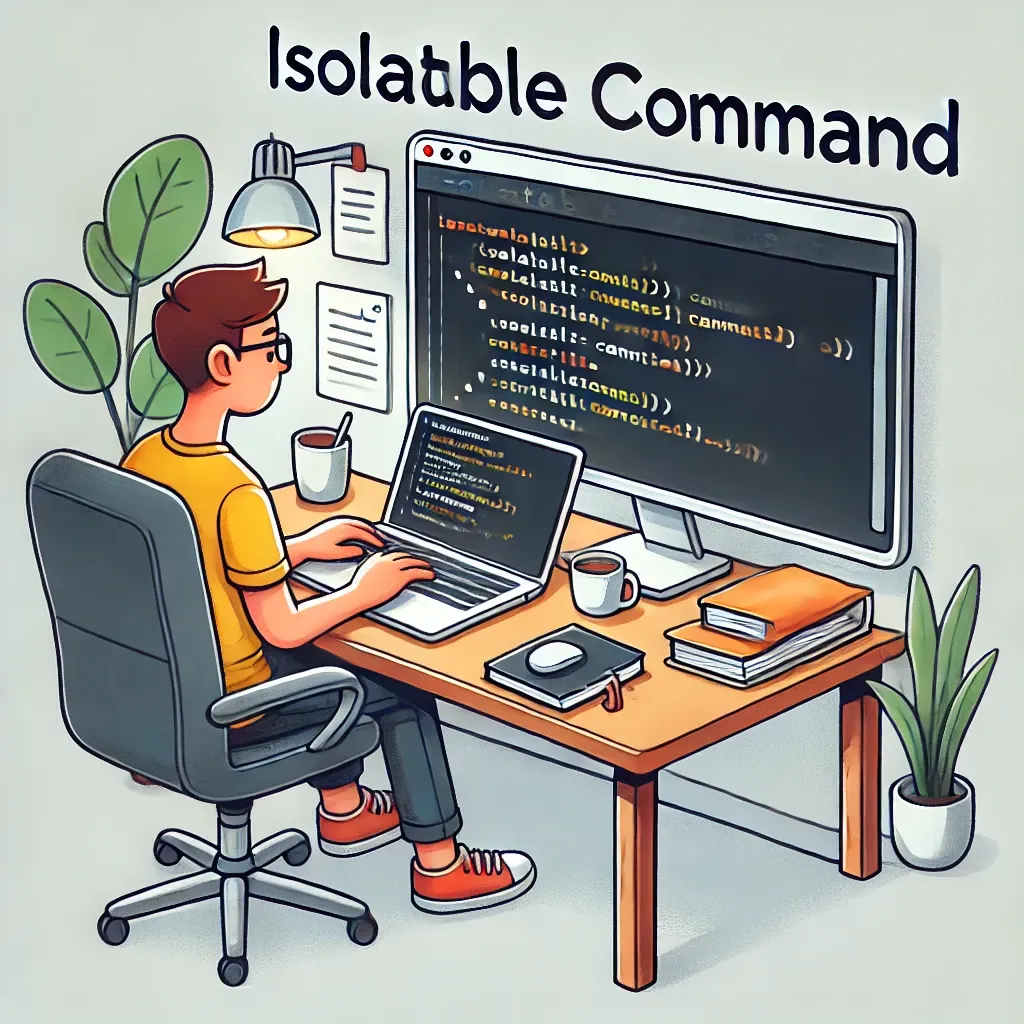
In complex Laravel applications, managing the execution of Artisan commands can sometimes be challenging, especially when dealing with long-running tasks or operations that should not overlap. Laravel's Isolatable interface provides a powerful solution to ensure that only one instance of a command can run at a time. Let's dive into how you can leverage this feature in your Laravel projects.
Understanding Isolatable Commands
The Isolatable interface in Laravel allows you to mark a command as isolatable, ensuring that only one instance of that command can run at any given time. This is particularly useful for tasks that could cause issues if run concurrently, such as data migrations, batch processing, or system-wide updates.
Implementing Isolatable Commands
To make a command isolatable, you need to implement the Illuminate\Contracts\Console\Isolatable
interface. Here's a basic example:
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
use Illuminate\Contracts\Console\Isolatable;
class ProcessDataCommand extends Command implements Isolatable
{
protected $signature = 'data:process';
protected $description = 'Process large amounts of data';
public function handle()
{
// Long-running data processing logic
}
}
By implementing the Isolatable interface, Laravel automatically adds an --isolated
option to your command.
Using Isolatable Commands
To run an isolatable command and ensure it's the only instance running:
php artisan data:process --isolated
If another instance of the command is already running, the new instance will not execute, but it will still exit with a successful status code.
Specifying Custom Exit Codes
You can specify a custom exit code for when the command can't run due to isolation:
php artisan data:process --isolated=12
This will return an exit code of 12 if the command can't run due to another instance already running.
Real-World Scenario: Batch Email Processing
Let's consider a more complex example where we need to process a large batch of emails, but we want to ensure only one instance of this process runs at a time:
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
use Illuminate\Contracts\Console\Isolatable;
use App\Services\EmailProcessingService;
class ProcessBatchEmailsCommand extends Command implements Isolatable
{
protected $signature = 'emails:process-batch';
protected $description = 'Process a batch of pending emails';
protected $emailService;
public function __construct(EmailProcessingService $emailService)
{
parent::__construct();
$this->emailService = $emailService;
}
public function handle()
{
$this->info('Starting batch email processing...');
$processedCount = $this->emailService->processPendingBatch();
$this->info("Processed {$processedCount} emails.");
}
}
To use this command with isolation:
php artisan emails:process-batch --isolated
This ensures that even if the command is triggered multiple times (e.g., by different cron jobs or users), only one instance will run, preventing duplicate email sends or resource conflicts.
Laravel's Isolatable interface provides a simple yet powerful way to manage command execution in scenarios where concurrent runs could cause issues. By implementing this interface, you can ensure the integrity of your data processing tasks, avoid resource conflicts, and create more robust, predictable command-line operations in your Laravel applications.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!