Enhancing Actions with Laravel's Fluent Class
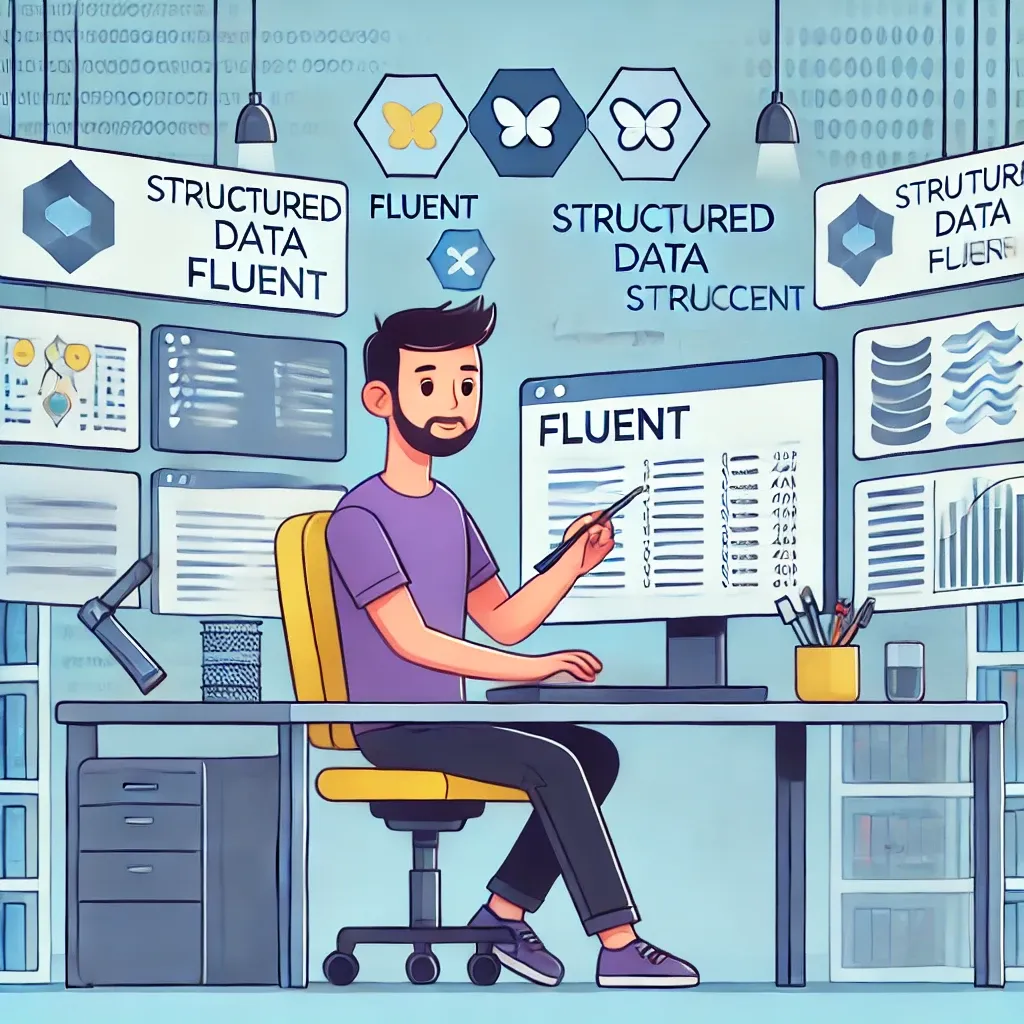
Laravel's Fluent class brings powerful data transformation to your action classes. When passing attributes to actions, consider upgrading from arrays to Fluent instances.
While arrays work fine for passing data to actions, Fluent instances offer built-in transformation methods and cleaner validation capabilities. This approach becomes especially valuable when dealing with complex data transformations or conditional validation.
Let's see how it works:
namespace App\Actions;
use App\Models\User;
use Illuminate\Support\Fluent;
class RegisterUser
{
// Instead of array $data
public function execute(Fluent $data): User
{
return User::create([
'name' => $data->name,
'email' => $data->email,
'preferences' => $data->array('preferences'),
'role' => $data->enum('role', UserRole::class),
'joined_at' => $data->date('joined_at')
]);
}
}
// In your test
it('can register a new user', function() {
$user = (new RegisterUser)->execute(new Fluent([
'name' => 'John Doe',
'email' => 'john@example.com',
'preferences' => ['theme' => 'dark'],
'role' => UserRole::Member,
'joined_at' => now()
]));
});
Real-World Example
Here's how you might use Fluent with conditional validation:
class SubscriptionValidator
{
public function validate(array $data)
{
$validator = Validator::make($data, [
'plan' => 'required|string',
'period' => 'required|in:monthly,yearly'
]);
$validator->sometimes(
['company_name', 'vat_number'],
'required',
function (Fluent $input) {
return $input->plan === 'business';
}
);
$validator->sometimes(
'student_id',
'required|string',
function (Fluent $input) {
return $input->get('plan') === 'student' &&
$input->get('period') === 'yearly';
}
);
return $validator;
}
}
The Fluent class provides a more elegant way to handle data in your actions and validations. Think of it as a Swiss Army knife for data transformation - it provides helpful methods while maintaining a clean interface.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!