Enhanced Filesystem Error Handling in Laravel
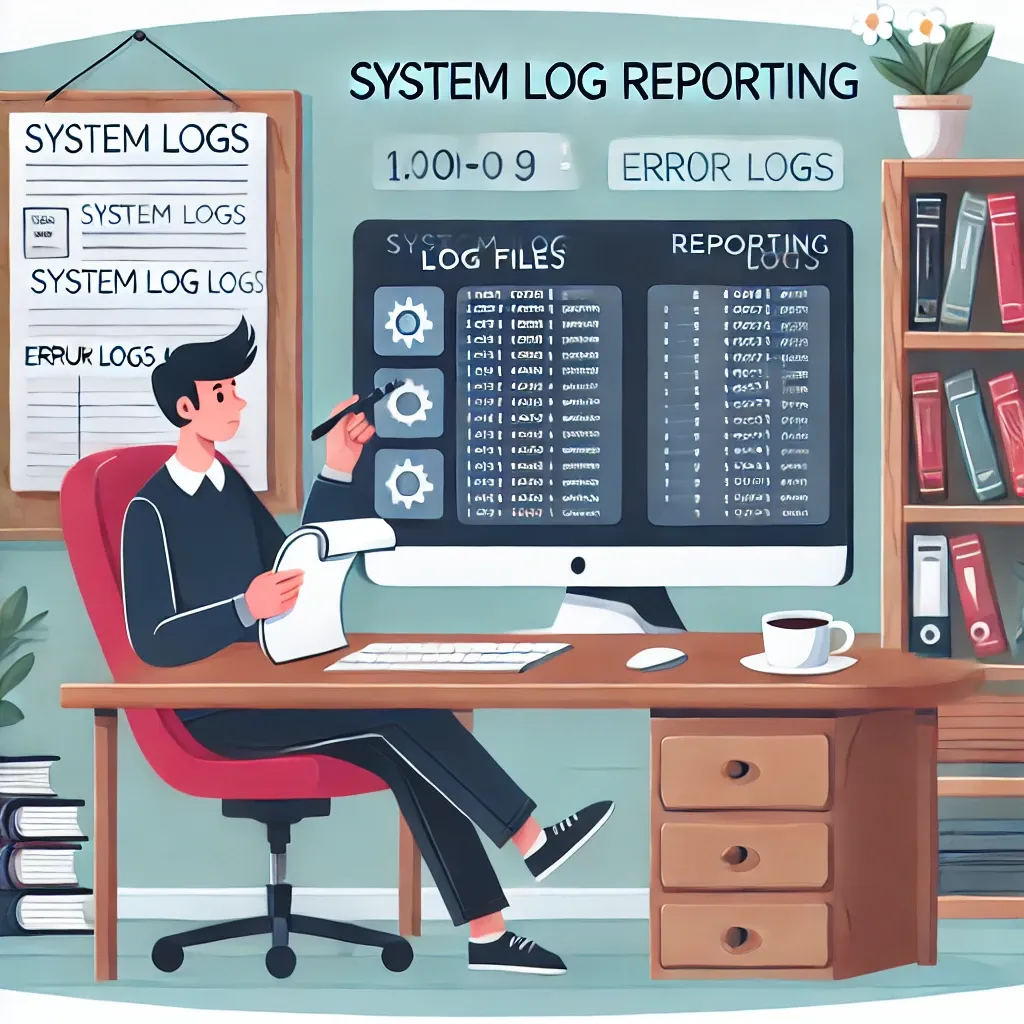
Laravel enhances filesystem error handling with new configuration options, allowing you to log filesystem errors without disrupting your application flow.
Managing filesystem errors traditionally meant choosing between catching exceptions or letting them disrupt your application. Laravel's new configuration options provide a middle ground, letting you log errors silently while keeping your application running smoothly.
Let's see how it works:
// config/filesystems.php
'disks' => [
'local' => [
'driver' => 'local',
'root' => storage_path('app'),
'throw' => false, // Suppress exceptions
'report' => true, // Enable error logging
],
]
Real-World Example
Here's how you might use it in a file management system:
class FileManager
{
public function handleUserUploads(array $files)
{
// Using disk configured to log errors without throwing
$disk = Storage::disk('user-uploads');
foreach ($files as $file) {
// Operation continues even if some files fail
if ($disk->put("uploads/{$file->hashName()}", $file)) {
$this->markFileAsUploaded($file);
}
}
}
public function syncBackups()
{
return [
// Critical backups - throw exceptions
'critical' => Storage::disk('critical-backups')
->files('daily'),
// Non-critical - just log errors
'optional' => Storage::disk('optional-backups')
->files('weekly')
];
}
}
// Disk configurations
'disks' => [
'user-uploads' => [
'driver' => 'local',
'throw' => false,
'report' => true, // Log errors silently
],
'critical-backups' => [
'driver' => 's3',
'throw' => true, // Throw exceptions
],
'optional-backups' => [
'driver' => 's3',
'throw' => false,
'report' => true, // Just log errors
],
]
The new configuration options provide granular control over error handling behavior. This is particularly valuable when dealing with different types of filesystem operations with varying levels of criticality.
Think of it as a configurable error handling strategy - you can choose between strict exception-based handling and silent logging based on each disk's requirements. This becomes especially useful when:
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!