Enhance Your Queue System with Laravel's New JobQueueing Event
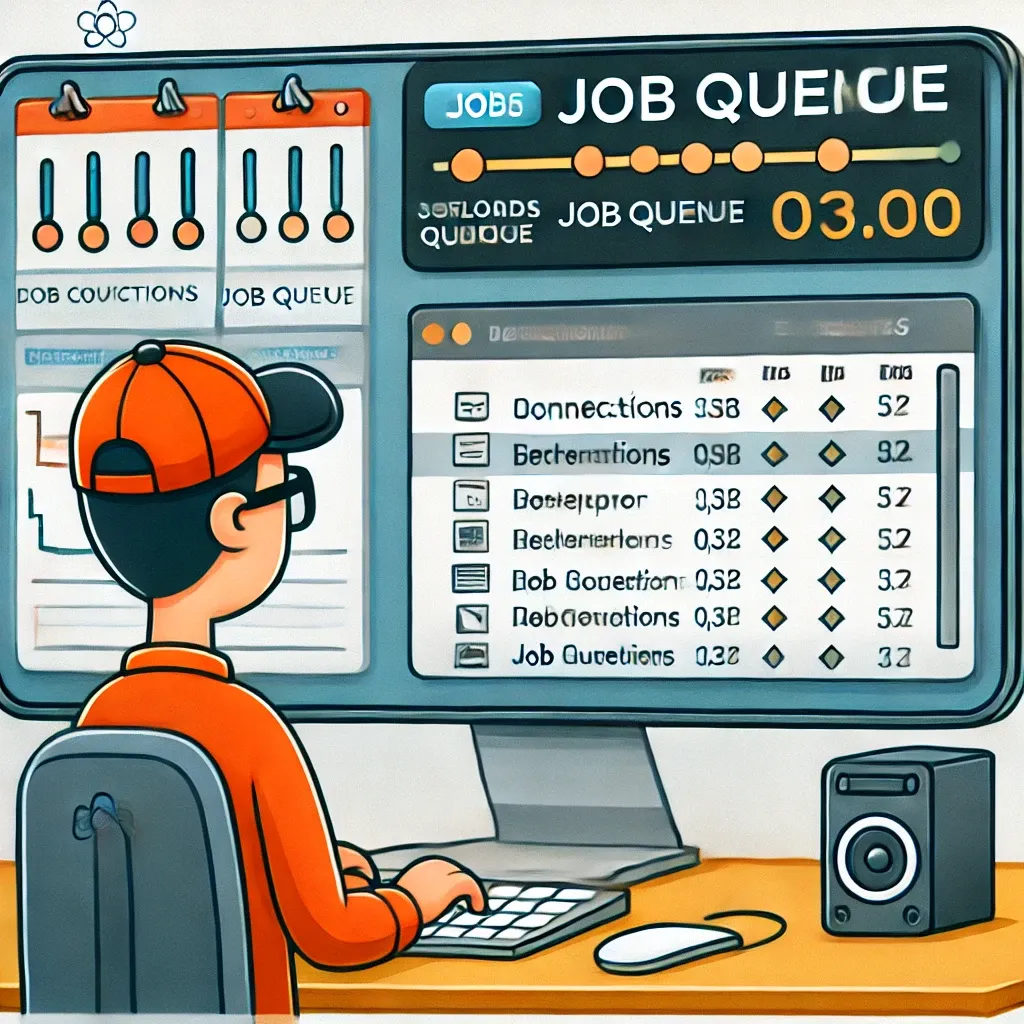
Laravel's queue system just got more flexible with the introduction of the JobQueueing event. This pre-queue hook opens up new possibilities for monitoring and manipulating jobs before they're dispatched.
Laravel's event-driven queue system already provides several hooks for tracking a job's lifecycle, such as JobProcessing and JobProcessed. Now, with the addition of the JobQueueing event, you can tap into an earlier stage - the moment just before a job is sent to your queue provider.
Let's see how this new event works:
use Illuminate\Queue\Events\JobQueueing;
use Illuminate\Support\Facades\Event;
// Listen for the JobQueueing event
Event::listen(function (JobQueueing $event) {
// Access the job, connection name, and payload
$job = $event->job;
$connectionName = $event->connectionName;
$payload = $event->payload;
// Perform actions before the job is queued
});
Real-World Example
This event unlocks several practical use cases. Here's how you might use it in a service provider:
<?php
namespace App\Providers;
use Illuminate\Queue\Events\JobQueueing;
use Illuminate\Support\Facades\Event;
use Illuminate\Support\ServiceProvider;
use Illuminate\Support\Facades\Log;
class AppServiceProvider extends ServiceProvider
{
public function boot(): void
{
// Log information about jobs being queued
Event::listen(function (JobQueueing $event) {
if (app()->environment('local')) {
Log::info('Queueing job', [
'job' => get_class($event->job),
'connection' => $event->connectionName,
'queue' => $event->payload['queue'] ?? 'default'
]);
}
});
}
}
By leveraging the JobQueueing event, you can build more sophisticated queue monitoring systems or implement custom logic that needs to run just before a job enters your queue provider.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter (https://x.com/harrisrafto), Bluesky (https://bsky.app/profile/harrisrafto.eu), and YouTube (https://www.youtube.com/@harrisrafto). It helps a lot!