Enhance your Eloquent models with casts in Laravel
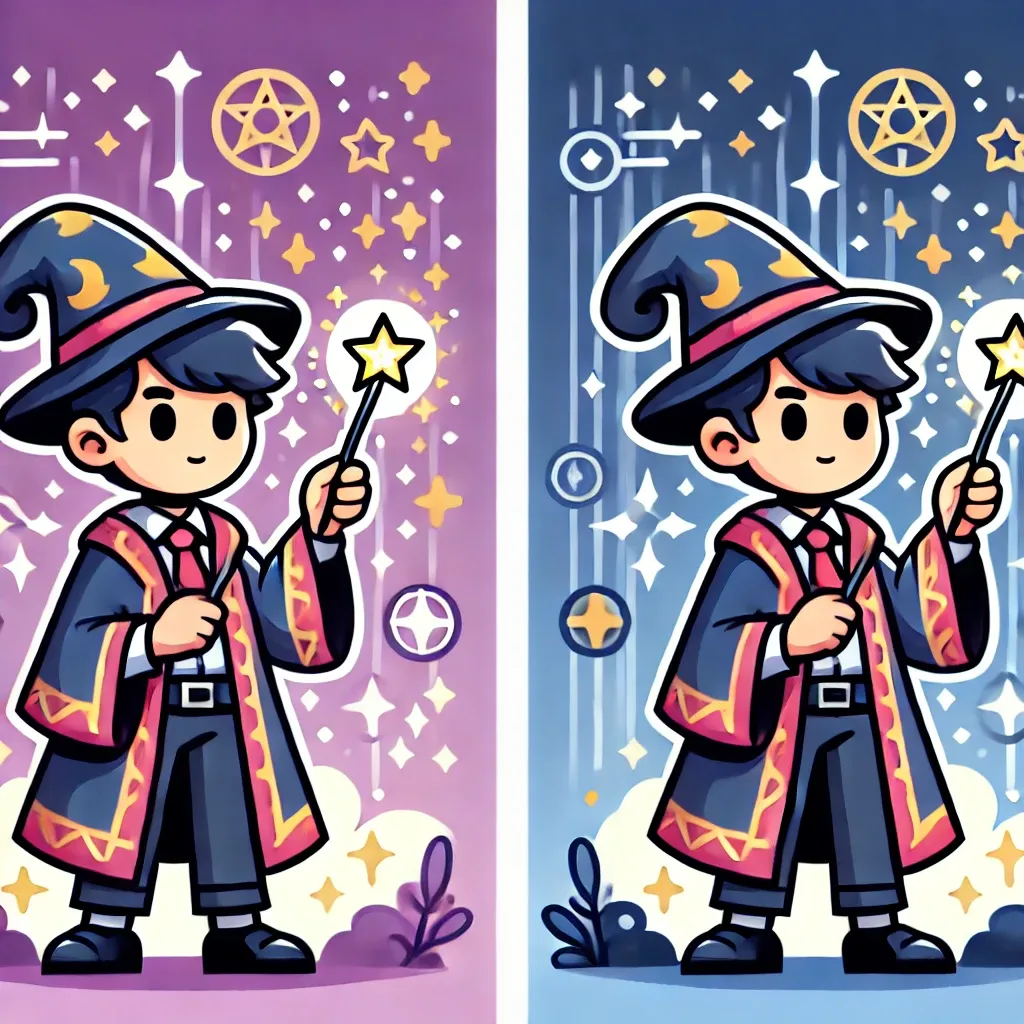
Managing data types and ensuring data consistency is a fundamental part of building robust applications. Laravel's Eloquent ORM provides a powerful feature called casts
that allows developers to automatically convert attributes to common data types, simplifying model logic and ensuring data integrity.
Understanding casts
The casts
property in an Eloquent model allows you to specify how attributes should be converted when they are accessed or set. This ensures that the data is always in the expected format, making it easier to work with different types of data without manually converting them each time.
Basic Usage
Here’s a basic example to illustrate how casts
work:
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
protected $casts = [
'is_admin' => 'boolean',
'created_at' => 'datetime',
];
}
In this example, the is_admin
attribute is automatically cast to a boolean, and the created_at
attribute is cast to a datetime
object. This ensures that whenever these attributes are accessed, they are in the expected format.
Real-Life Example
Consider a scenario where you have a blog application with a Post
model. You want to cast the published_at
attribute to a datetime
object and the tags
attribute to an array. Here’s how you can implement this:
- Defining the Model:
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
protected $casts = [
'published_at' => 'datetime',
'tags' => 'array',
];
}
- Using the Casted Attributes:
$post = Post::find(1);
// Accessing the 'published_at' attribute as a Carbon instance
echo $post->published_at->format('Y-m-d H:i:s');
// Accessing the 'tags' attribute as an array
foreach ($post->tags as $tag) {
echo $tag;
}
In this example, the published_at
attribute is cast to a Carbon
instance, allowing you to use all of Carbon’s date and time manipulation methods. The tags
attribute is cast to an array, making it easier to iterate over and manipulate.
Conclusion
The casts
property in Laravel Eloquent is a powerful tool for managing data types and ensuring consistency within your application. By leveraging this feature, you can simplify your model logic, reduce boilerplate code, and enhance the overall maintainability of your application.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!