Enhance your app’s performance with cache()->rememberForever() in Laravel
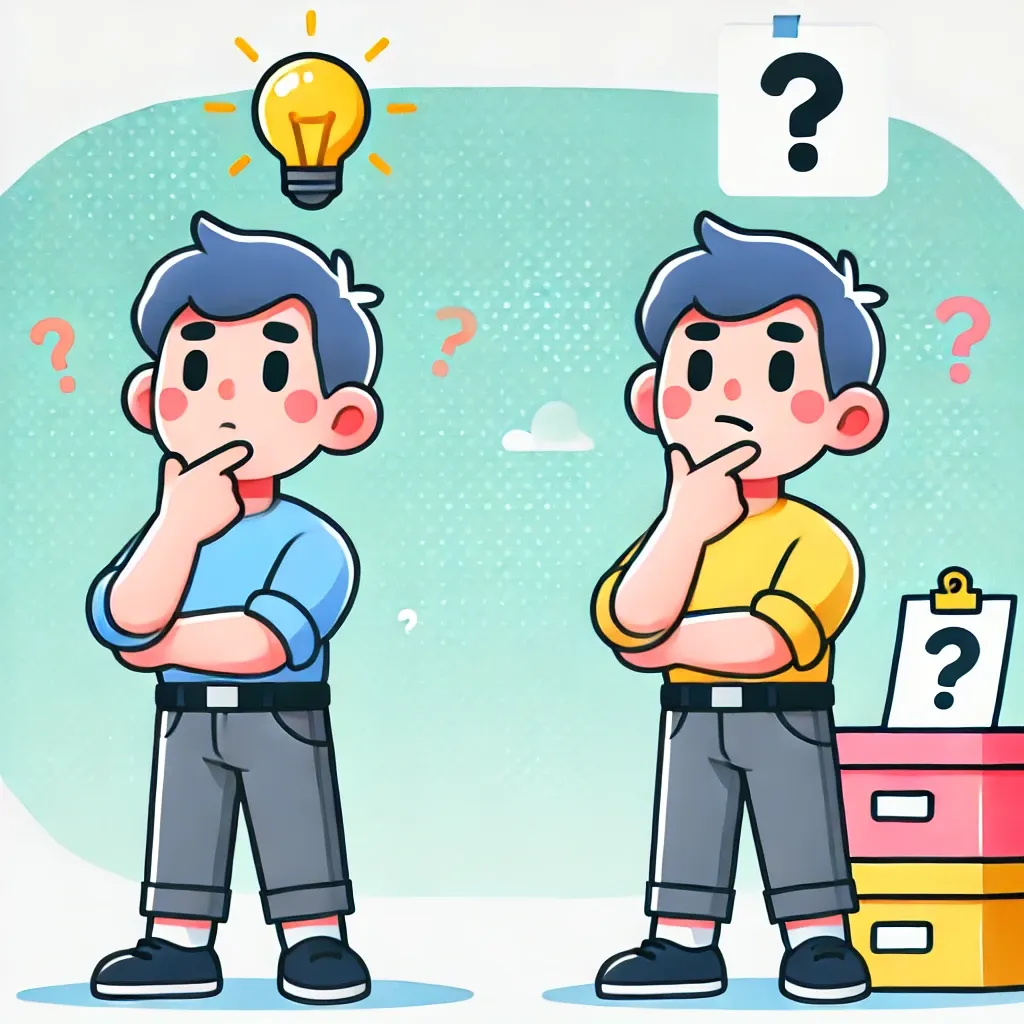
Efficiently managing and caching data is crucial for optimizing the performance of high-traffic applications. Laravel provides a robust caching system that allows developers to store data temporarily or indefinitely. One of the powerful methods available in Laravel's caching system is cache()->rememberForever()
. This method enables you to cache critical data indefinitely, ensuring that your application can retrieve it quickly without repeatedly querying the database.
Understanding cache()->rememberForever()
The cache()->rememberForever()
method allows you to store data in the cache permanently. Unlike temporary caching methods that require you to set an expiration time, this method ensures that the data remains in the cache until it is explicitly removed. This is particularly useful for data that does not change frequently but is accessed often, such as user roles, settings, or static pages.
Basic Usage
Here’s a basic example to illustrate how cache()->rememberForever()
works:
$users = Cache::rememberForever('users', function () {
return User::all();
});
In this example, the list of users is cached indefinitely. The rememberForever
method first checks if the data is already in the cache. If it is not, the closure provided will be executed, and the result will be stored in the cache. Subsequent requests will retrieve the data from the cache, significantly reducing database load and improving response times.
In this example, the list of users is cached indefinitely. The rememberForever
method first checks if the data is already in the cache. If it is not, the closure provided will be executed, and the result will be stored in the cache. Subsequent requests will retrieve the data from the cache, significantly reducing database load and improving response times.
Real-Life Example
Consider a scenario where you have a blog application, and you want to cache the list of categories since they do not change frequently. Here’s how you can implement this using cache()->rememberForever()
:
use Illuminate\Support\Facades\Cache;
use App\Models\Category;
class CategoryController extends Controller
{
public function index()
{
$categories = Cache::rememberForever('categories', function () {
return Category::all();
});
return view('categories.index', compact('categories'));
}
}
In this example, the CategoryController
retrieves the list of categories from the cache. If the categories are not already cached, the closure fetches them from the database and stores them in the cache. This approach ensures that the categories are only queried from the database once, and subsequent requests retrieve them from the cache, improving the application's performance.
Pro Tip
To ensure that your cache remains up-to-date, you can clear the cached data whenever a category is added, updated, or deleted. This can be done using the Cache::forget
method:
public function store(Request $request)
{
// Store the new category
$category = Category::create($request->all());
// Clear the categories cache
Cache::forget('categories');
return redirect()->route('categories.index');
}
In this example, after storing a new category, the Cache::forget
method is used to remove the cached categories. The next time the categories are requested, they will be retrieved from the database and cached again.
Conclusion
The cache()->rememberForever()
method in Laravel is a powerful tool for optimizing the performance of your applications. By caching frequently accessed but rarely changing data, you can significantly reduce database load and improve response times. Incorporate this method into your Laravel projects to ensure efficient data management and a smoother user experience.