Enhance loading performance with wire:loading.class in Livewire
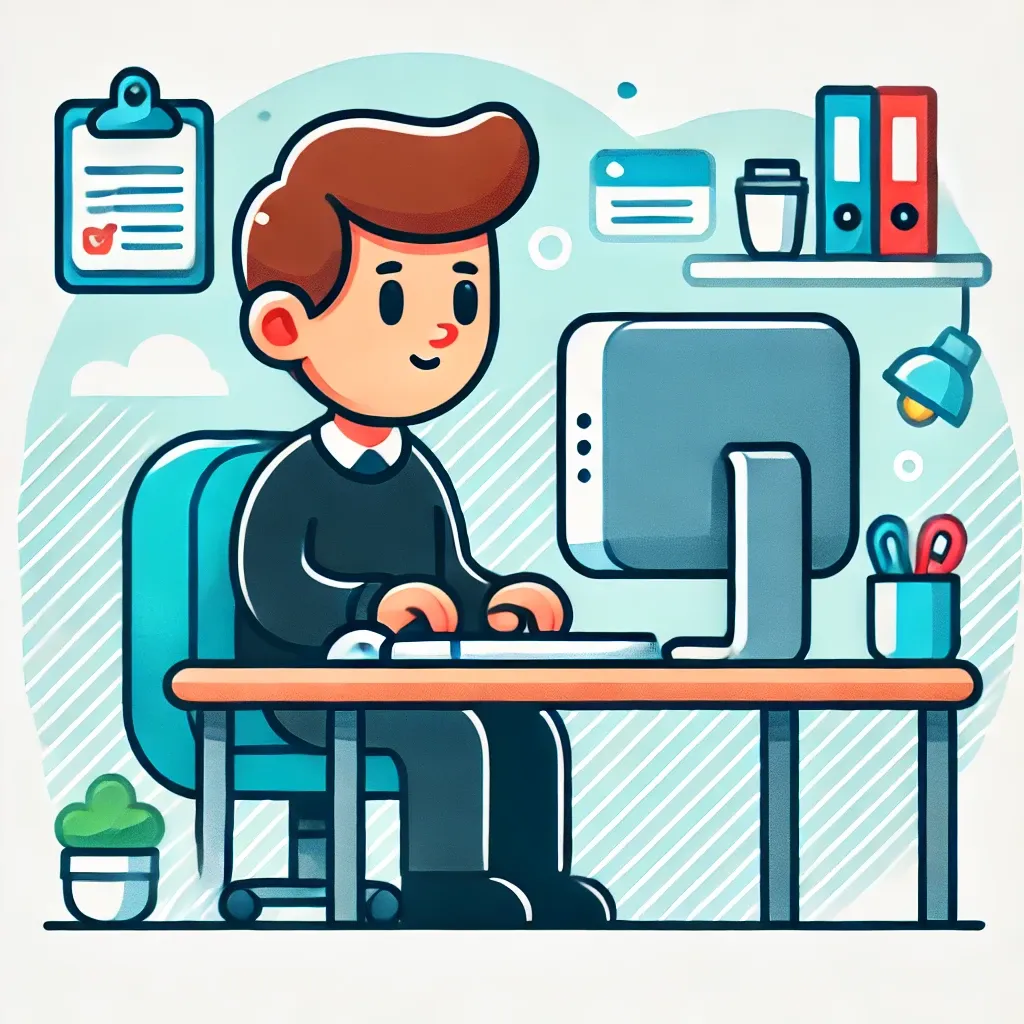
Improving the user experience by providing visual feedback during asynchronous operations is crucial in modern web applications. Laravel Livewire offers a powerful directive, wire:loading.class
, which allows you to dynamically add CSS classes to elements while waiting for actions to complete. This enhances the user experience by providing clear visual indicators during loading states.
Understanding wire:loading.class
The wire:loading.class
directive allows you to add a CSS class to an element while a Livewire request is being processed. This is particularly useful for displaying loading spinners, changing button styles, or indicating that a section of the page is updating.
Basic Usage
Here’s a basic example to illustrate how wire:loading.class
works:
<button wire:click="save" wire:loading.class="is-loading">
Save
</button>
In this example, the is-loading
class is added to the button while the save
method is being processed. This class can be used to style the button to indicate that a loading process is happening.
Real-Life Example
Consider a scenario where you have a form submission button, and you want to provide visual feedback to the user while the form is being submitted. Here’s how you can implement this using wire:loading.class
:
- Livewire Component:
use Livewire\Component;
class ContactForm extends Component
{
public $name;
public $email;
public $message;
public function submit()
{
// Simulate a delay
sleep(1);
// Handle the form submission
// For example: Contact::create(['name' => $this->name, 'email' => $this->email, 'message' => $this->message]);
session()->flash('message', 'Thank you for your message!');
}
public function render()
{
return view('livewire.contact-form');
}
}
- Blade Template:
<!-- Blade Template (livewire/contact-form.blade.php) -->
<form wire:submit.prevent="submit">
<input type="text" wire:model="name" placeholder="Name">
<input type="email" wire:model="email" placeholder="Email">
<textarea wire:model="message" placeholder="Message"></textarea>
<button type="submit" wire:loading.class="is-loading">
Send
</button>
<div wire:loading.class="loading-indicator">
<span>Sending...</span>
</div>
@if (session()->has('message'))
<div>{{ session('message') }}</div>
@endif
</form>
In this example, the is-loading
class is applied to the button while the form is being submitted, and the loading-indicator
class is applied to a div to display a loading message. This provides clear visual feedback to the user, enhancing the overall user experience.
Pro Tip
Combine wire:loading.class
with CSS animations to create more engaging loading indicators. For example, you can add a spinning animation to the button:
.is-loading {
position: relative;
pointer-events: none;
}
.is-loading::after {
content: '';
position: absolute;
right: 10px;
top: 50%;
transform: translateY(-50%);
border: 2px solid #fff;
border-top: 2px solid #3498db;
border-radius: 50%;
width: 14px;
height: 14px;
animation: spin 0.6s linear infinite;
}
@keyframes spin {
0% { transform: rotate(0deg); }
100% { transform: rotate(360deg); }
}
This CSS will create a spinner next to the button text while the form is being submitted, providing a clear and engaging loading indicator.
Conclusion
The wire:loading.class
directive in Livewire is a powerful tool for improving the user experience by providing visual feedback during asynchronous operations. By leveraging this directive, you can create more responsive and interactive interfaces, enhancing the overall usability of your application.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!