Elevating Request Handling with Enums in Laravel
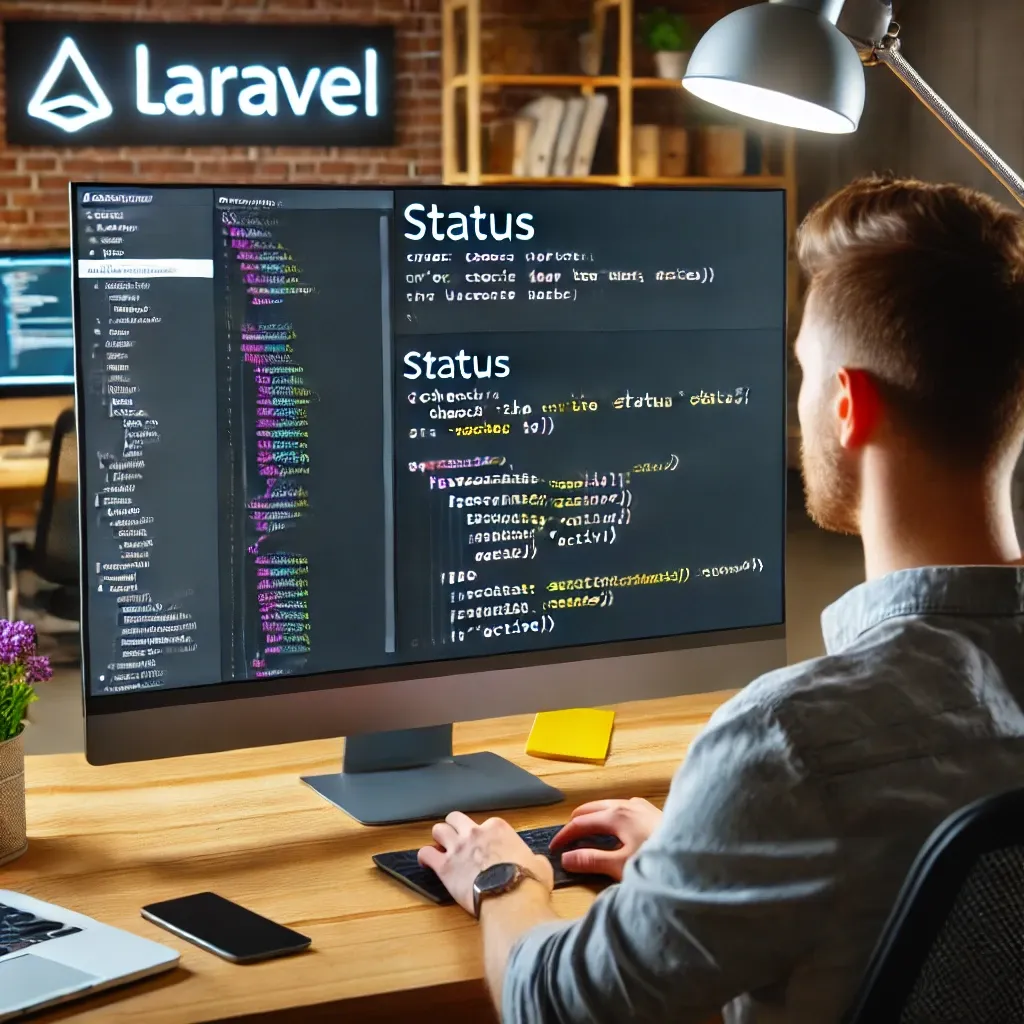
PHP 8.1 introduced enums, and Laravel has seamlessly integrated this powerful feature into its request handling capabilities. With the enum
method on the request object, you can now effortlessly retrieve and validate enum values from incoming requests. Let's explore how this feature can enhance your Laravel applications.
Understanding $request->enum()
The enum
method on the request object allows you to retrieve input data as an instance of a specified enum class. Here's the basic syntax:
$enumValue = $request->enum('input_name', EnumClass::class);
If the input value doesn't exist or doesn't match any backing value in the enum, null
is returned.
Real-Life Example
Let's consider a scenario where we're building an API to manage tasks. Each task has a status that can be 'pending', 'in_progress', or 'completed'. We'll use an enum to represent these statuses.
First, let's define our enum:
<?php
namespace App\Enums;
enum TaskStatus: string
{
case PENDING = 'pending';
case IN_PROGRESS = 'in_progress';
case COMPLETED = 'completed';
}
Now, let's create a controller method to update a task's status:
<?php
namespace App\Http\Controllers;
use App\Enums\TaskStatus;
use App\Models\Task;
use Illuminate\Http\Request;
class TaskController extends Controller
{
public function updateStatus(Request $request, Task $task)
{
$newStatus = $request->enum('status', TaskStatus::class);
if ($newStatus === null) {
return response()->json(['error' => 'Invalid status provided'], 400);
}
$task->status = $newStatus;
$task->save();
return response()->json($task);
}
}
In this example, we're using $request->enum()
to:
- Retrieve the 'status' input as a
TaskStatus
enum - Validate that the provided status is valid (not null)
- Update the task with the new status
Here's what the input and output might look like:
// PUT /api/tasks/1
// Input
{
"status": "in_progress"
}
// Output (success)
{
"id": 1,
"title": "Complete project proposal",
"status": "in_progress",
"updated_at": "2023-06-15T15:30:00.000000Z"
}
// Input with invalid status
{
"status": "started"
}
// Output (error)
{
"error": "Invalid status provided"
}
By leveraging $request->enum()
, you can create more robust, type-safe applications that align closely with your domain model. This feature bridges the gap between your application's internal representation of data and the incoming requests, providing a clean, efficient way to handle enumerated types in your Laravel applications.
If you found this tip helpful, don't forget to subscribe to my daily newsletter and follow me on X/Twitter for more Laravel gems!