Elegant Pipeline Cleanup with Laravel's finally Method
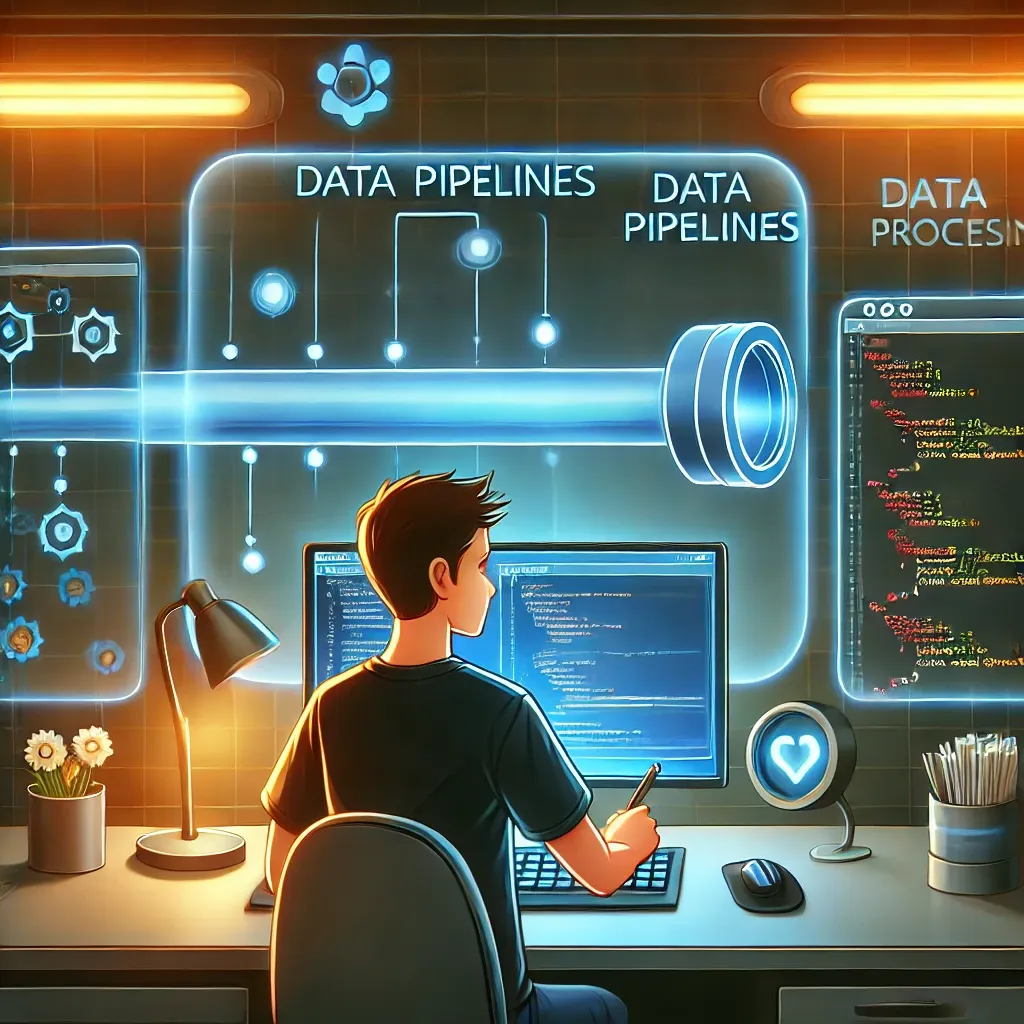
Pipeline cleanup gets more elegant in Laravel with the new finally method. This addition simplifies how you handle post-pipeline operations, whether your pipeline succeeds or fails.
Managing cleanup operations in pipelines traditionally required wrapping your pipeline in a try-finally block. Laravel's new finally method streamlines this process by integrating cleanup directly into the pipeline chain, making your code more readable and maintainable.
Let's see how it works:
$result = Pipeline::send($deployment)
->through([PipeOne::class, PipeTwo::class])
->finally(fn () => /** perform cleanup */)
->then(fn () => $deployment->deploy());
Real-World Example
Here's how you might use it in a deployment system:
class DeploymentManager
{
public function deploy(Deployment $deployment)
{
return Pipeline::send($deployment)
->through([
ValidateEnvironment::class,
BackupCurrentState::class,
UpdateDependencies::class,
RunMigrations::class
])
->finally(function () use ($deployment) {
// Always cleanup temporary files
Storage::delete("temp/{$deployment->id}");
// Release deployment lock
Cache::forget("deployment-lock:{$deployment->id}");
// Log completion timestamp
$deployment->update(['completed_at' => now()]);
})
->then(fn () => $this->finalizeDeployment($deployment));
}
protected function finalizeDeployment(Deployment $deployment)
{
return [
'status' => 'completed',
'deployment_id' => $deployment->id
];
}
}
The finally method brings several advantages over traditional try-finally blocks. It keeps your cleanup logic within the pipeline chain, making the code flow more natural and easier to follow. This is particularly valuable when dealing with complex operations that require proper resource cleanup.
Think of it as a built-in cleanup handler for your pipeline - it ensures your cleanup code runs regardless of whether the pipeline succeeds, fails, or gets interrupted. This becomes especially useful when:
- Managing temporary resources
- Releasing locks or semaphores
- Cleaning up temporary files
- Updating status records
- Logging completion states
For example, if your deployment process creates temporary files or acquires locks, the finally method ensures these are cleaned up even if an error occurs during the deployment process.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!