Elegant Factory Association with Laravel's UseFactory Attribute
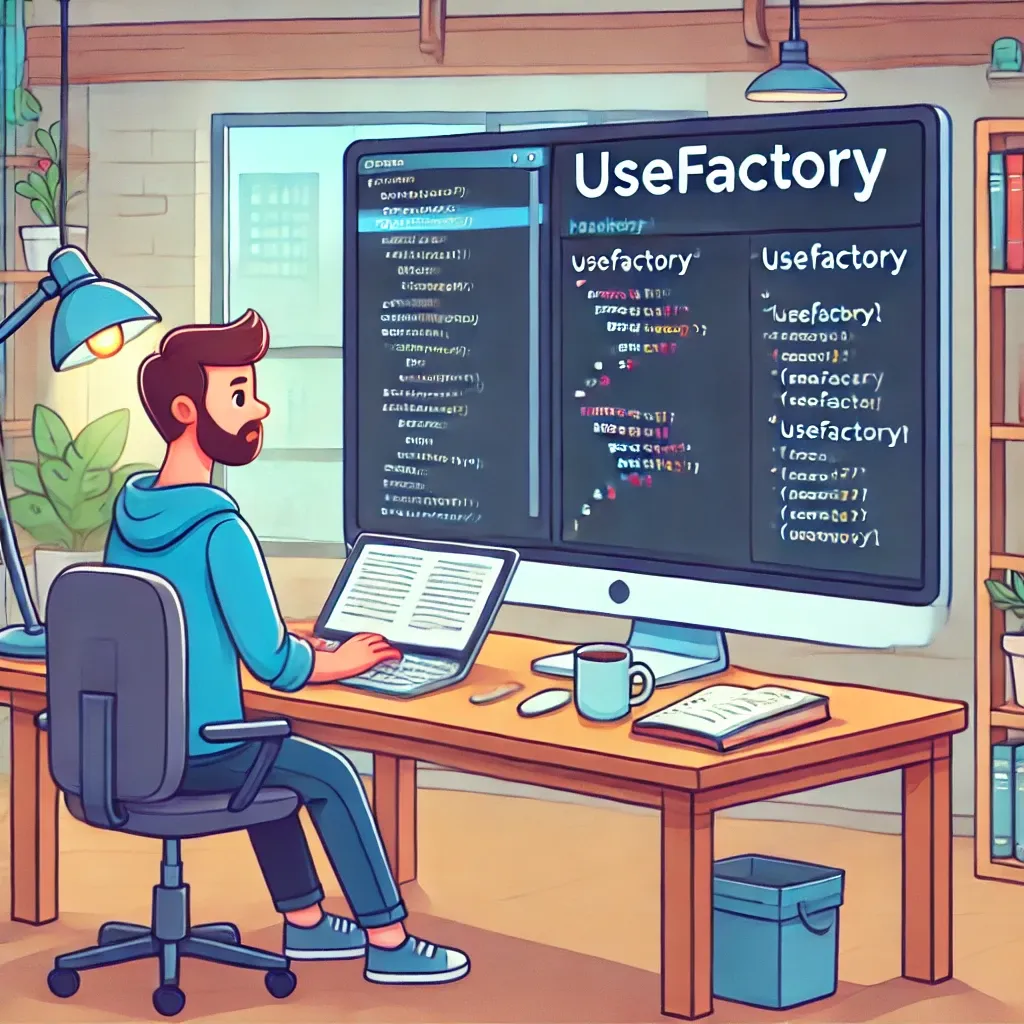
Need a cleaner way to associate factories with your Eloquent models? Laravel's new UseFactory attribute provides an elegant solution using PHP 8.0+ attributes, especially useful for models outside the standard namespace.
Factory registration in Laravel traditionally required either following strict naming conventions or manually defining factory methods. The new UseFactory attribute simplifies this process by providing a declarative way to specify factory associations directly on your models.
Let's see how it works:
use Database\Factories\CommentFactory;
use Illuminate\Database\Eloquent\Attributes\UseFactory;
#[UseFactory(CommentFactory::class)]
class Comment extends Model
{
use HasFactory;
}
Real-World Example
Here's how you might use it in a domain-driven structure:
// Post model
namespace App\Domains\Blog\Models;
use Database\Factories\PostFactory;
use Illuminate\Database\Eloquent\Attributes\UseFactory;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\Relations\HasMany;
#[UseFactory(PostFactory::class)]
class Post extends Model
{
use HasFactory;
protected $fillable = ['title', 'content', 'status'];
#[UseFactory(CommentFactory::class)]
public function comments(): HasMany
{
return $this->hasMany(Comment::class);
}
}
// Product model
namespace App\Domains\Shop\Models;
#[UseFactory(ProductFactory::class)]
class Product extends Model
{
use HasFactory;
// No need to define newFactory() or $model property
// The factory association is handled by the attribute
}
The UseFactory attribute brings several advantages over traditional factory registration methods. It provides a clear, visible association between models and their factories while maintaining backward compatibility with existing approaches. Laravel follows a priority order when resolving factories:
- Static $factory property if defined
- UseFactory attribute if present
- Convention-based factory resolution
Think of it as a decorator for your models - it clearly indicates which factory should be used without requiring additional method definitions or strict naming conventions.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!