Efficient File Downloads with Laravel Livewire's streamDownload
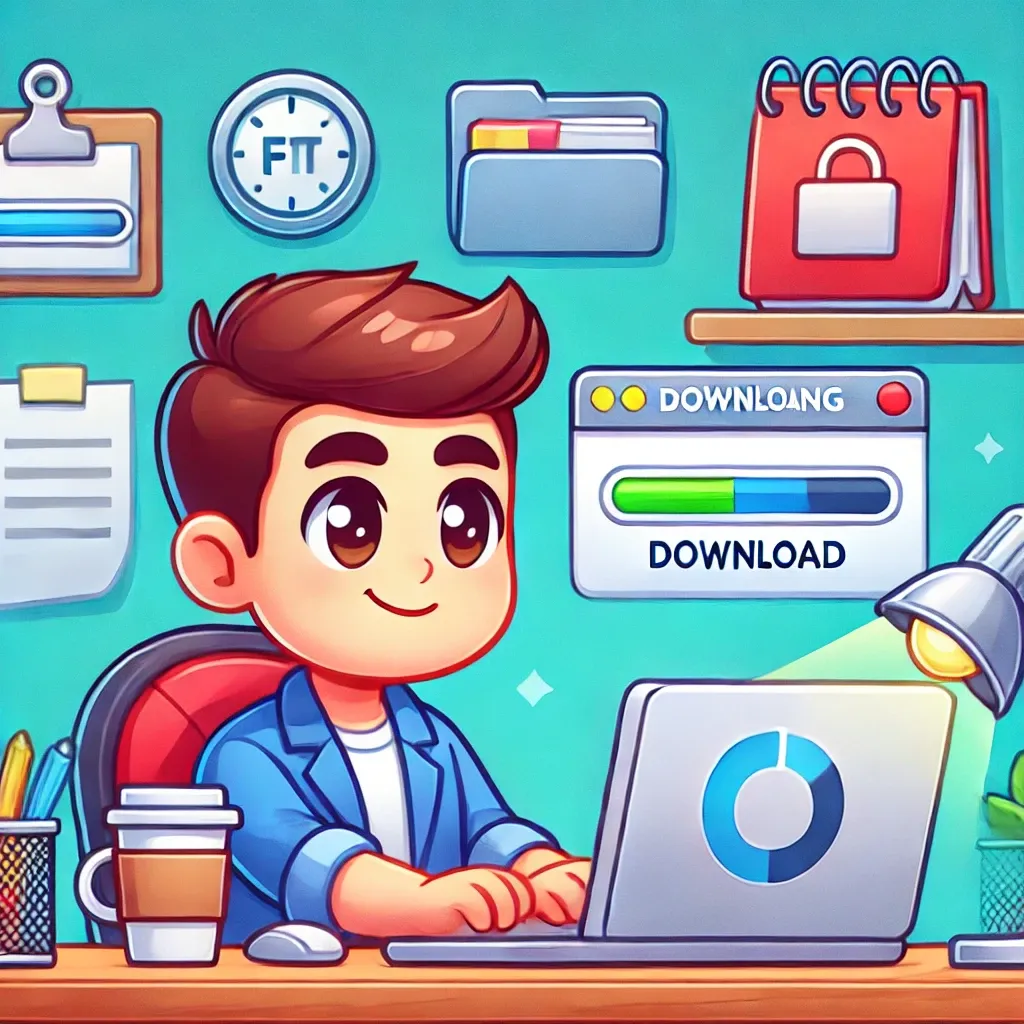
As web developers, we often face the challenge of serving large files to users efficiently. When working with Laravel Livewire, there's a powerful feature that can help us tackle this problem: streamDownload. In this post, we'll explore how to use streamDownload to serve large files without overwhelming your server's memory.
What is streamDownload?
streamDownload is a method provided by Livewire that allows you to serve file downloads to users by streaming the content directly to the browser. This means you can generate or process large files on the fly without having to store them on your server or load them entirely into memory.
How to Use streamDownload
Here's a basic example of how to use streamDownload:
use Livewire\Component;
use Livewire\WithFileDownloads;
class ReportComponent extends Component
{
use WithFileDownloads;
public function downloadReport()
{
return $this->streamDownload(function () {
$csv = fopen('php://output', 'w');
fputcsv($csv, ['Name', 'Email']);
foreach (User::all() as $user) {
fputcsv($csv, [$user->name, $user->email]);
}
fclose($csv);
}, 'users-report.csv');
}
}
Let's break this down:
- We use the WithFileDownloads trait to enable file download capabilities in our Livewire component.
- The downloadReport method uses streamDownload to generate and serve a CSV file.
- Inside the streamDownload callback, we generate the CSV content on the fly.
- The second parameter of streamDownload specifies the filename for the download.
Benefits of Using streamDownload
- Memory Efficiency: You can serve large files without loading them entirely into memory.
- On-the-fly Generation: Generate dynamic content right before serving it to the user.
- Improved User Experience: Users can start downloading immediately, even for large files.
- Server Resource Optimization: Reduce disk usage by not storing temporary files.
Real-World Example: Generating a Large Report
Let's consider a more complex real-world scenario where streamDownload can be incredibly useful: generating a large sales report in an e-commerce application.
use Livewire\Component;
use Livewire\WithFileDownloads;
class SalesReportComponent extends Component
{
use WithFileDownloads;
public $startDate;
public $endDate;
public function downloadSalesReport()
{
return $this->streamDownload(function () {
$csv = fopen('php://output', 'w');
fputcsv($csv, ['Date', 'Product', 'Quantity', 'Total']);
$sales = Sale::whereBetween('created_at', [$this->startDate, $this->endDate])
->with('product')
->lazy();
foreach ($sales as $sale) {
fputcsv($csv, [
$sale->created_at->toDateString(),
$sale->product->name,
$sale->quantity,
$sale->total
]);
}
fclose($csv);
}, "sales-report-{$this->startDate}-to-{$this->endDate}.csv");
}
public function render()
{
return view('livewire.sales-report');
}
}
In this example:
- We allow users to specify a date range for the report.
- We use Laravel's lazy loading to efficiently process a large number of sales records.
- We generate the CSV file on-the-fly, writing each row as we process it.
- The filename includes the date range, making it easy for users to identify different reports.
This approach allows us to generate potentially very large reports without putting undue stress on our server's memory. Even if the report contains millions of rows, we're processing and outputting them one at a time.
Conclusion
Livewire's streamDownload feature is a powerful tool for efficiently serving large files or dynamically generated content to your users. By allowing you to stream content directly to the browser, it helps you optimize your application's performance and provide a better user experience.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!