Download Files Easily with Laravel's HTTP sink Method
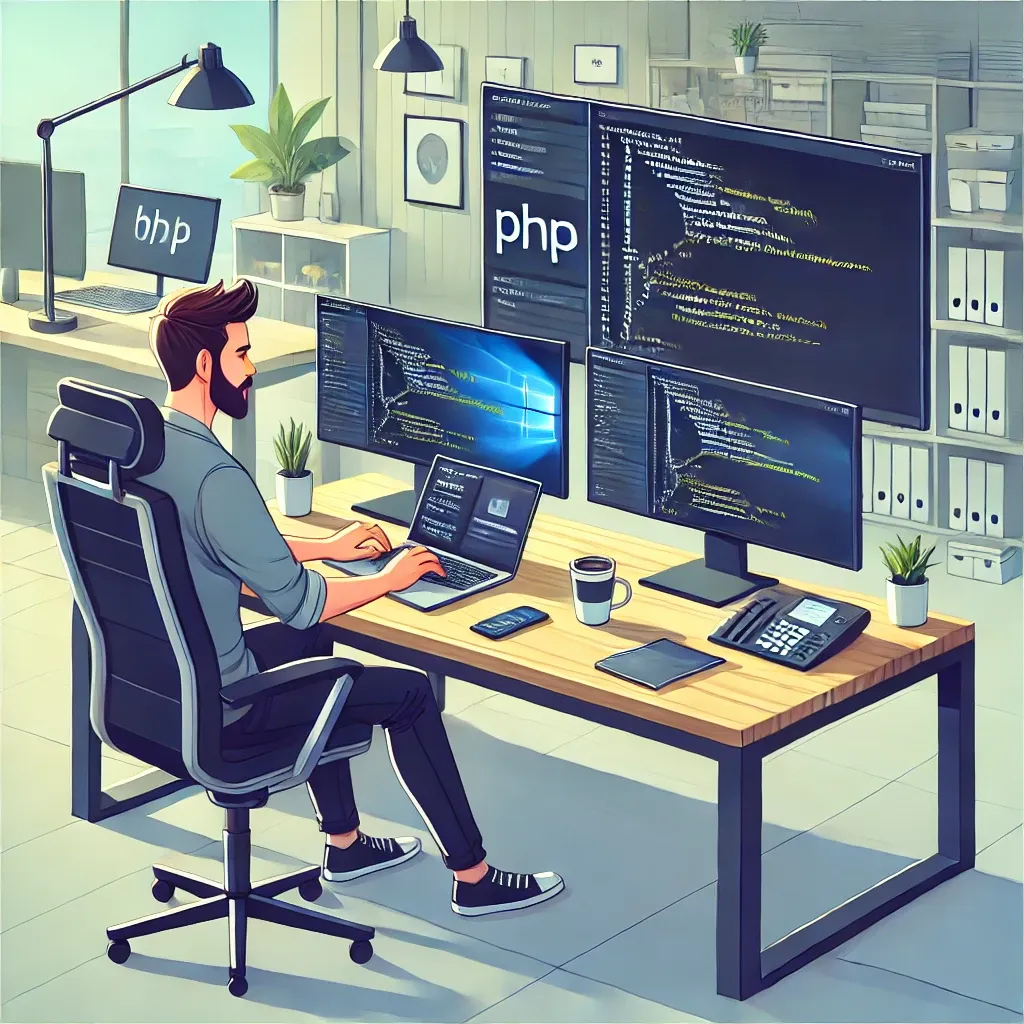
Need to download files with HTTP requests? Laravel's sink method provides a clean way to save HTTP responses directly to files with minimal code.
When downloading files in Laravel applications, you often need to handle the file writing process, manage memory efficiently, and deal with large file downloads. The sink method simplifies all of this by providing a direct pipeline from HTTP responses to your storage, handling the file writing process automatically.
Let's see how simple it is to use:
Http::sink(storage_path('download.zip'))
->get('https://example.com/example-file.zip');
Real-World Example
Here's how you might use it in a download manager:
class DownloadManager
{
public function downloadFile(string $url, string $filename)
{
return Http::sink(storage_path("downloads/{$filename}"))
->withHeaders([
'User-Agent' => 'MyApp/1.0',
'Accept' => '*/*'
])
->get($url);
}
public function downloadBackup(string $backupUrl, string $apiKey)
{
$timestamp = now()->format('Y-m-d-His');
return Http::sink(storage_path("backups/backup-{$timestamp}.zip"))
->withToken($apiKey)
->get($backupUrl);
}
public function downloadReportAsCsv(string $reportUrl)
{
return Http::sink(
storage_path('reports/latest.csv'),
withHeaders([
'Accept' => 'text/csv'
])
)->get($reportUrl);
}
}
The sink method simplifies file downloads by handling the file writing process for you. This means you don't need to manually manage file streams or worry about memory usage with large files, as the content is written directly to disk.
Think of it as a direct pipeline from the HTTP response to your storage - Laravel manages the complexities of file handling while you focus on building your application. This is especially useful when:
- Downloading large files that shouldn't be loaded entirely into memory
- Handling file downloads in background jobs
- Implementing automatic backup systems
- Creating file caching mechanisms
For example, if you're downloading a 1GB file, the sink method ensures it's written directly to disk instead of being loaded into your application's memory first. This makes it perfect for handling large files in production environments where memory constraints are important.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!