Discover the power of Str::replaceArray() in Laravel
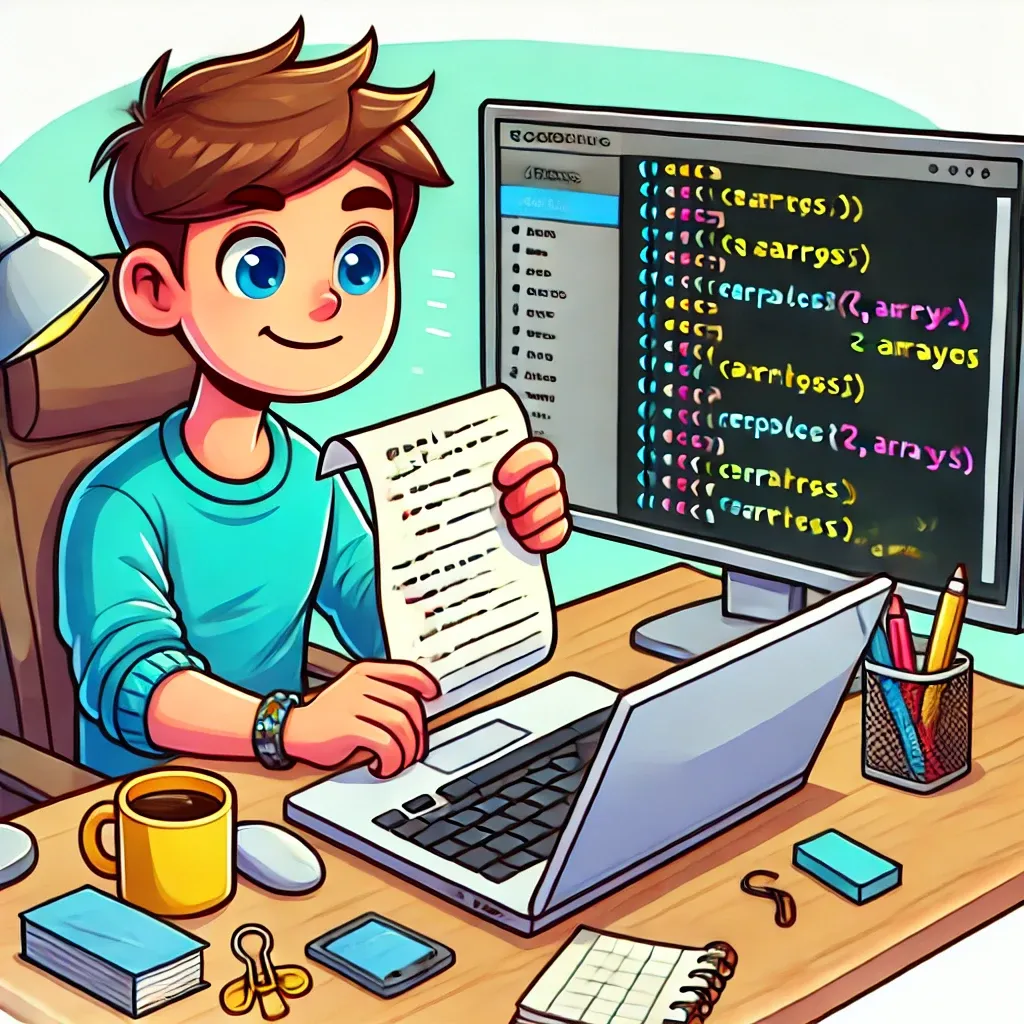
Working with dynamic strings often requires replacing multiple placeholders with specific values. Laravel’s Str::replaceArray()
function provides a powerful and efficient way to handle this. Let's dive into how you can use Str::replaceArray()
to simplify string manipulation in your Laravel projects.
Understanding Str::replaceArray()
The Str::replaceArray()
method is part of the Illuminate\Support\Str
class. It allows you to replace all occurrences of a given value within a string using an array of replacements. This is particularly useful for templating and dynamic content generation.
Basic Usage
Here’s a basic example to illustrate how Str::replaceArray()
works:
use Illuminate\Support\Str;
$template = 'The event will take place on ?, at ?';
$replaced = Str::replaceArray('?', ['Monday', '10 AM'], $template);
echo $replaced; // Output: The event will take place on Monday, at 10 AM
In this example, Str::replaceArray()
replaces each ?
in the template string with the corresponding value from the replacements array.
Real-Life Example
Imagine you are developing an application that sends out dynamic email notifications. You want to replace placeholders in your email templates with actual data. Here’s how Str::replaceArray()
can help:
use Illuminate\Support\Str;
class NotificationController extends Controller
{
public function sendEventNotification($event)
{
$template = 'Hello ?, your event ? is scheduled for ? at ?.';
$replacements = [
$event->user->name,
$event->name,
$event->date->format('l'),
$event->time->format('h:i A'),
];
$message = Str::replaceArray('?', $replacements, $template);
// Send the email using the generated message
Mail::to($event->user->email)->send(new EventNotification($message));
return response()->json(['status' => 'Notification sent successfully.']);
}
}
In this example, Str::replaceArray()
is used to replace placeholders in the email template with actual event details. This ensures that each notification is personalized and accurate.
Conclusion
The Str::replaceArray()
function in Laravel is an incredibly useful tool for replacing multiple placeholders within a string. Whether you’re generating dynamic content, creating templates, or managing user notifications, Str::replaceArray()
makes the task simple and efficient. Incorporate this function into your Laravel projects to enhance your string manipulation capabilities and streamline your workflow.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!