Detect Empty Values in Laravel with the blank Function
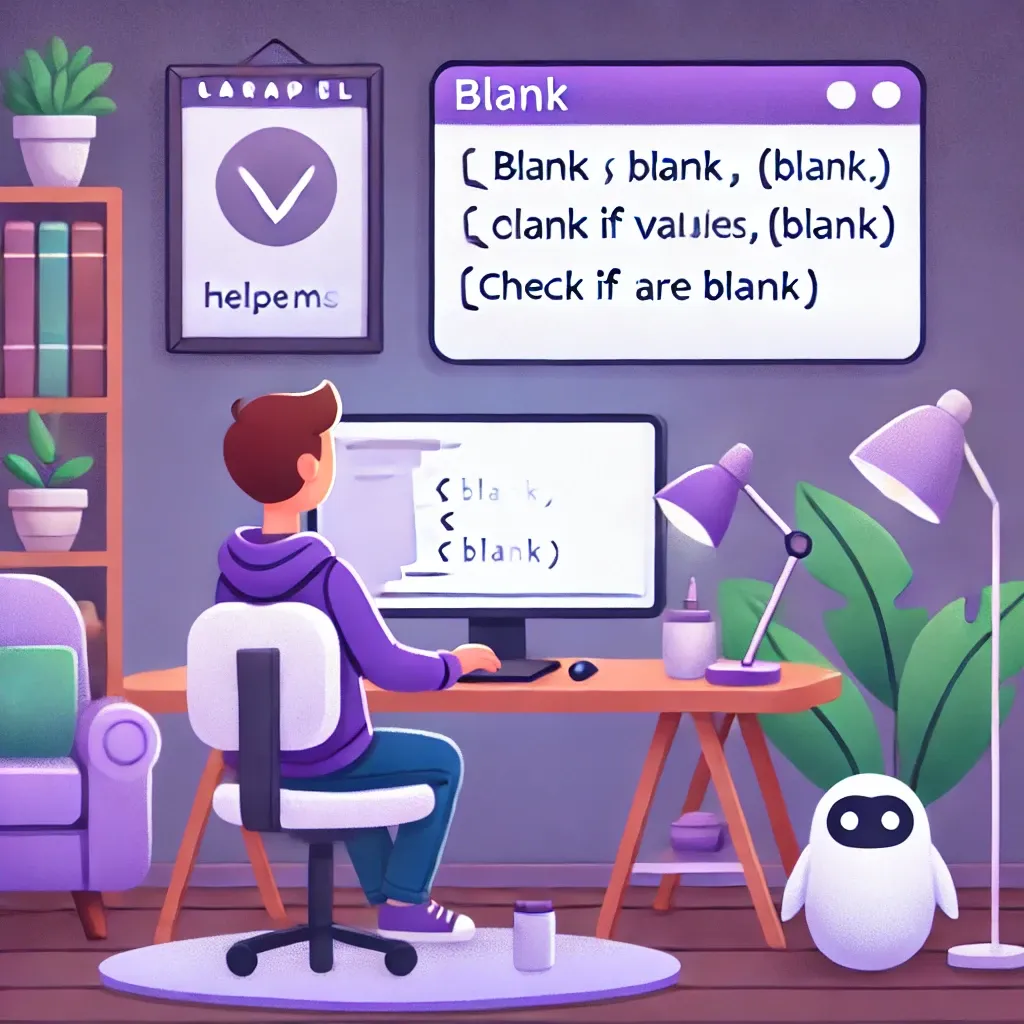
Validating empty values in PHP can be tricky. Laravel's blank function simplifies this process by providing a consistent way to check for 'blank' values.
Basic Usage
The blank
function works with various types:
// Returns true
blank('');
blank(' ');
blank(null);
blank(collect());
// Returns false
blank(0);
blank(true);
blank(false);
Real-World Example
Here's how you might use it in a form validation context:
class SubmissionValidator
{
public function validateUserInput(array $input)
{
$errors = [];
// Check required fields
foreach (['name', 'email', 'message'] as $field) {
if (blank($input[$field] ?? null)) {
$errors[$field] = "The {$field} field is required";
}
}
// Check optional fields with special conditions
if (!blank($input['phone'] ?? null) && !preg_match('/^[0-9-+\s()]*$/', $input['phone'])) {
$errors['phone'] = 'Invalid phone format';
}
// Validate array inputs
if (!blank($input['interests'] ?? null)) {
if (count($input['interests']) < 2) {
$errors['interests'] = 'Please select at least 2 interests';
}
}
return [
'valid' => empty($errors),
'errors' => $errors
];
}
public function processOrder($orderData)
{
// Skip empty notes
if (!blank($orderData['notes'])) {
$this->addOrderNotes($orderData['notes']);
}
// Check for empty shipping details
if (blank($orderData['shipping_address'])) {
throw new InvalidOrderException('Shipping address is required');
}
}
}
The blank
function provides a reliable way to check for empty values, making your validation logic cleaner and more consistent.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!