Deep Diving into Laravel's replaceRecursive Method for Collections
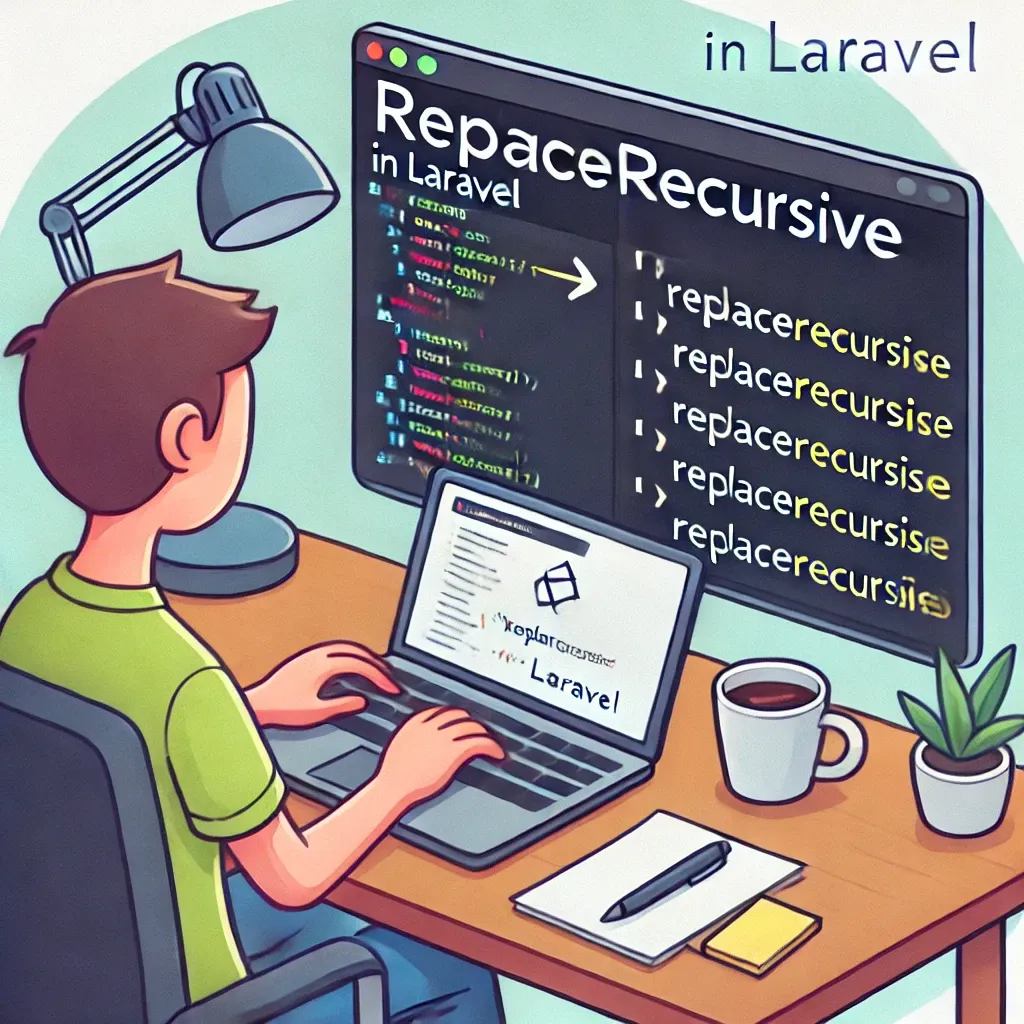
Laravel's Collection class offers a powerful suite of methods for manipulating arrays and data sets. One particularly useful method that often flies under the radar is replaceRecursive
. This method allows you to perform deep replacements in nested arrays or collections, providing a powerful tool for working with complex data structures.
Understanding replaceRecursive
The replaceRecursive
method works similarly to the replace
method, but it goes a step further by recursing into arrays and applying the same replacement process to nested values. This is particularly useful when working with multi-dimensional arrays or deeply nested data structures.
Basic Usage
Let's start with a simple example to illustrate how replaceRecursive
works:
$collection = collect([
'name' => 'John',
'profile' => [
'age' => 30,
'job' => 'Developer'
]
]);
$replaced = $collection->replaceRecursive([
'name' => 'Jane',
'profile' => [
'job' => 'Designer'
]
]);
// Result:
// [
// 'name' => 'Jane',
// 'profile' => [
// 'age' => 30,
// 'job' => 'Designer'
// ]
// ]
In this example, replaceRecursive
replaces the top-level 'name' key and the nested 'job' key, while leaving the 'age' key untouched.
Working with Nested Arrays
The real power of replaceRecursive
shines when working with deeply nested structures:
$collection = collect([
'settings' => [
'user' => [
'name' => 'John',
'preferences' => [
'theme' => 'dark',
'notifications' => true
]
]
]
]);
$replaced = $collection->replaceRecursive([
'settings' => [
'user' => [
'preferences' => [
'theme' => 'light'
]
]
]
]);
// Result:
// [
// 'settings' => [
// 'user' => [
// 'name' => 'John',
// 'preferences' => [
// 'theme' => 'light',
// 'notifications' => true
// ]
// ]
// ]
// ]
Here, replaceRecursive
dives deep into the nested structure to replace only the 'theme' value, leaving other values intact.
Real-World Scenario: Merging Configuration Files
Consider a scenario where you're building a plugin system for your Laravel application. Each plugin can have its own configuration, but you want to allow users to override these configurations partially. Here's how you might use replaceRecursive
to achieve this:
class PluginManager
{
public function mergeConfig($pluginName, $userConfig)
{
$defaultConfig = $this->getDefaultPluginConfig($pluginName);
return collect($defaultConfig)->replaceRecursive($userConfig)->all();
}
private function getDefaultPluginConfig($pluginName)
{
// Fetch default configuration for the plugin
return [
'enabled' => true,
'settings' => [
'cache' => [
'enabled' => true,
'duration' => 3600
],
'api' => [
'endpoint' => 'https://api.default.com',
'timeout' => 30
]
]
];
}
}
// Usage
$pluginManager = new PluginManager();
$mergedConfig = $pluginManager->mergeConfig('myPlugin', [
'settings' => [
'cache' => [
'duration' => 7200
],
'api' => [
'endpoint' => 'https://api.custom.com'
]
]
]);
// Result:
// [
// 'enabled' => true,
// 'settings' => [
// 'cache' => [
// 'enabled' => true,
// 'duration' => 7200
// ],
// 'api' => [
// 'endpoint' => 'https://api.custom.com',
// 'timeout' => 30
// ]
// ]
// ]
In this example, replaceRecursive
allows users to override specific configuration values without having to specify the entire configuration structure, maintaining default values for unspecified keys.
The replaceRecursive
method in Laravel's Collection class provides a powerful way to work with nested data structures. Whether you're merging configuration arrays, updating nested settings, or working with complex data from APIs, replaceRecursive
offers a clean and efficient solution for deep data manipulation in your Laravel applications.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!