Debug API Responses Faster with Laravel's New ddBody() Method
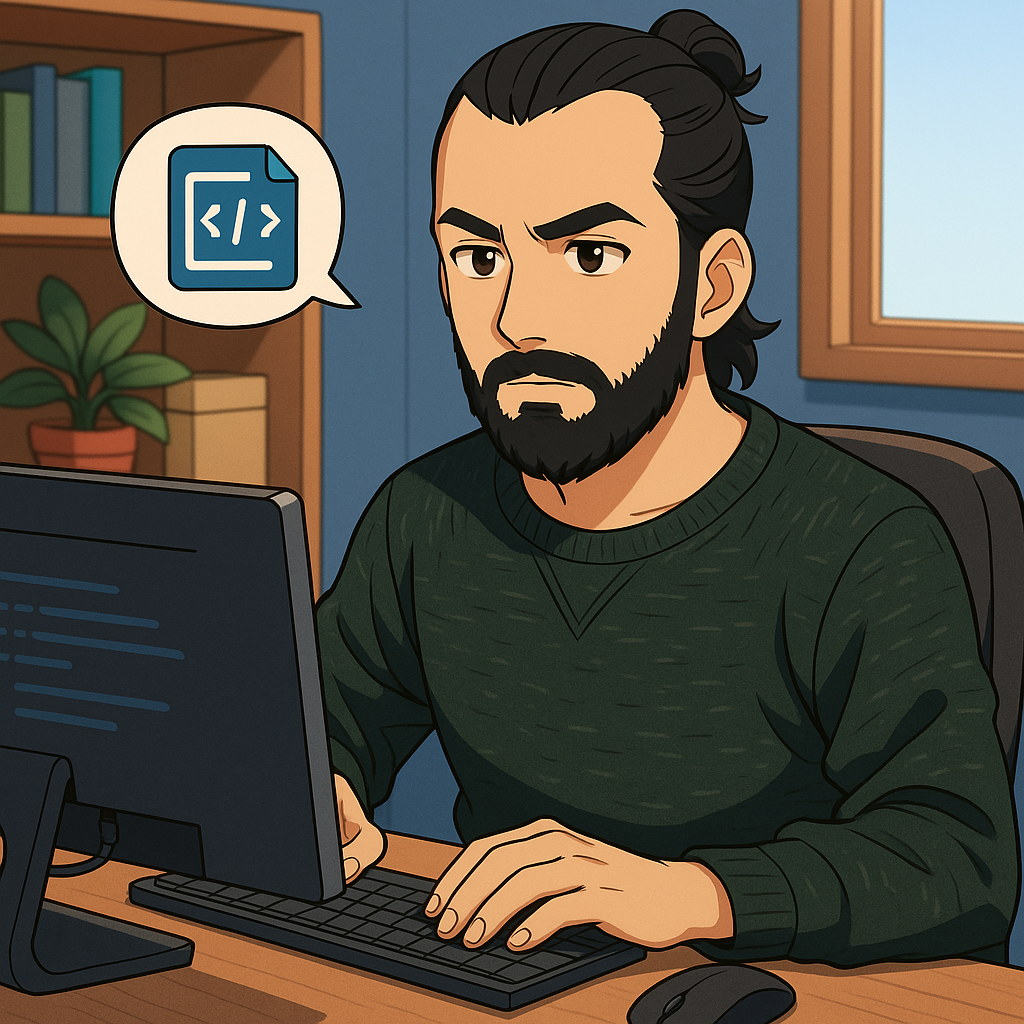
Debugging API responses in Laravel tests just got easier with the new ddBody() method. This convenient addition helps you quickly inspect response content without disrupting your testing workflow.
When writing feature tests for APIs, you often need to examine response content to understand why a test is failing. Previously, you might have used methods like dd() with json_decode or relied on assertJson to verify content. The new ddBody()
method streamlines this process by providing a direct way to dump the response body.
Let's see how it works:
public function test_api_returns_expected_data()
{
$response = $this->get('/api/products');
// Dump the entire response body
$response->ddBody();
// Continue with assertions...
$response->assertStatus(200);
}
Real-World Example
The ddBody() method becomes particularly useful when debugging complex API responses or when you need to examine specific parts of a JSON response:
public function test_product_creation()
{
$response = $this->postJson('/api/products', [
'name' => 'Test Product',
'price' => 19.99,
'category_id' => 1
]);
// Dump the entire response body
$response->ddBody();
// Or dump a specific JSON key
$response->ddBody('errors'); // Shows validation errors if any
// For JSON responses, this uses ddJson() under the hood
$response->ddBody('data.attributes.price'); // Show nested JSON values
}
The ddBody() method joins Laravel's family of debugging helpers like dd(), dump(), and ddd(), continuing the framework's focus on developer experience and productive debugging workflows.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter (https://x.com/harrisrafto), Bluesky (https://bsky.app/profile/harrisrafto.eu), and YouTube (https://www.youtube.com/@harrisrafto). It helps a lot!