Custom String IDs in Laravel Models: A New Approach
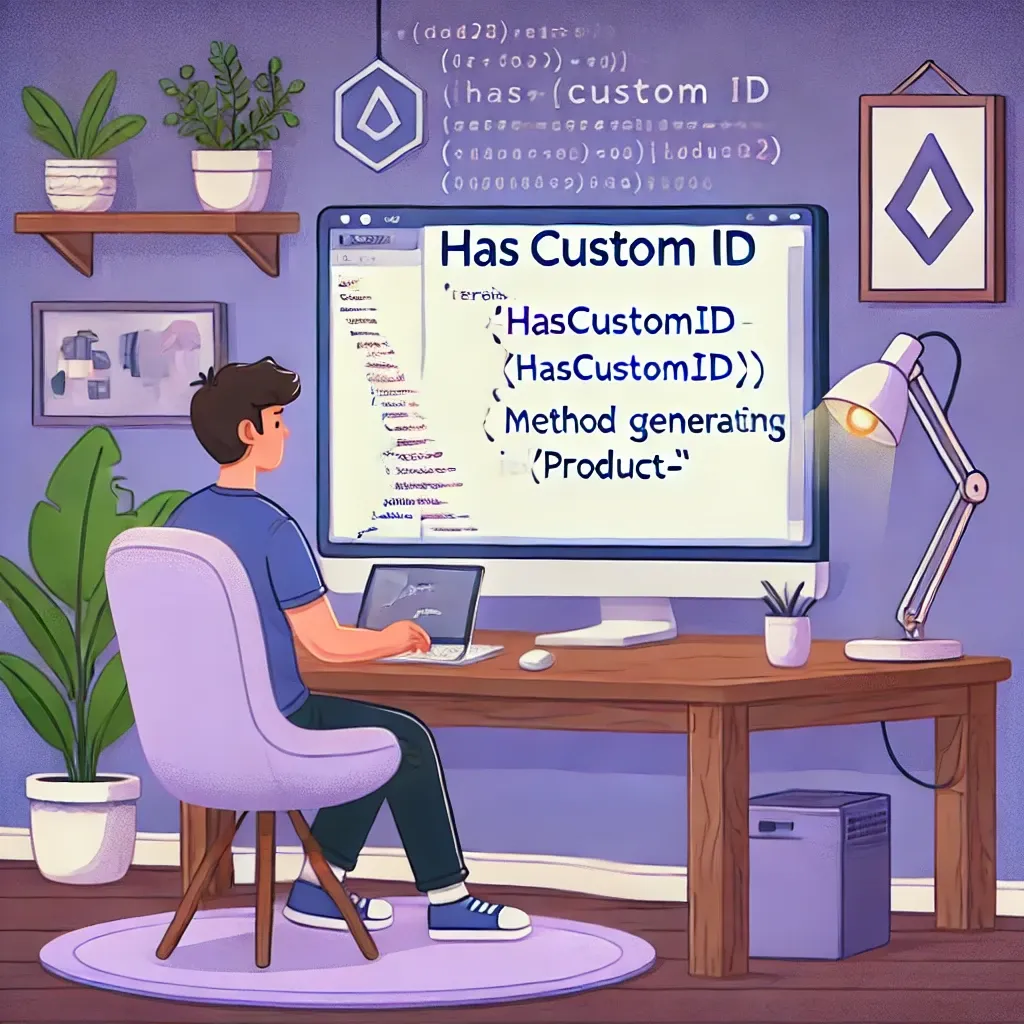
Need to use custom unique identifiers in your Laravel models? The refactored HasUniqueStringIds trait provides a clean way to implement custom string IDs without overriding route binding queries.
Basic Usage
Create your custom ID trait:
trait HasTwrnsTrait
{
use HasUniqueStringIds;
public function newUniqueId()
{
return (string) Twrn::new();
}
protected function isValidUniqueId($value): bool
{
return Twrn::isValid($value);
}
}
Real-World Example
Here's how to implement custom identifiers in your application:
class CustomIdentifierService
{
public static function generate(): string
{
return 'PRD-' . strtoupper(uniqid()) . '-' . rand(1000, 9999);
}
public static function isValid(string $value): bool
{
return preg_match('/^PRD-[A-Z0-9]+-\d{4}$/', $value);
}
}
trait HasCustomIdentifier
{
use HasUniqueStringIds;
public function newUniqueId(): string
{
return CustomIdentifierService::generate();
}
protected function isValidUniqueId($value): bool
{
return CustomIdentifierService::isValid($value);
}
}
class Product extends Model
{
use HasCustomIdentifier;
protected $keyType = 'string';
public $incrementing = false;
}
// Usage in routes
Route::get('/products/{product}', function (Product $product) {
return $product;
});
// Product URLs will look like:
// /products/PRD-5F9E2B3A-1234
The HasUniqueStringIds trait makes it straightforward to implement custom string identifiers while maintaining Laravel's elegant route model binding.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!