Cross-Process Lock Management in Laravel
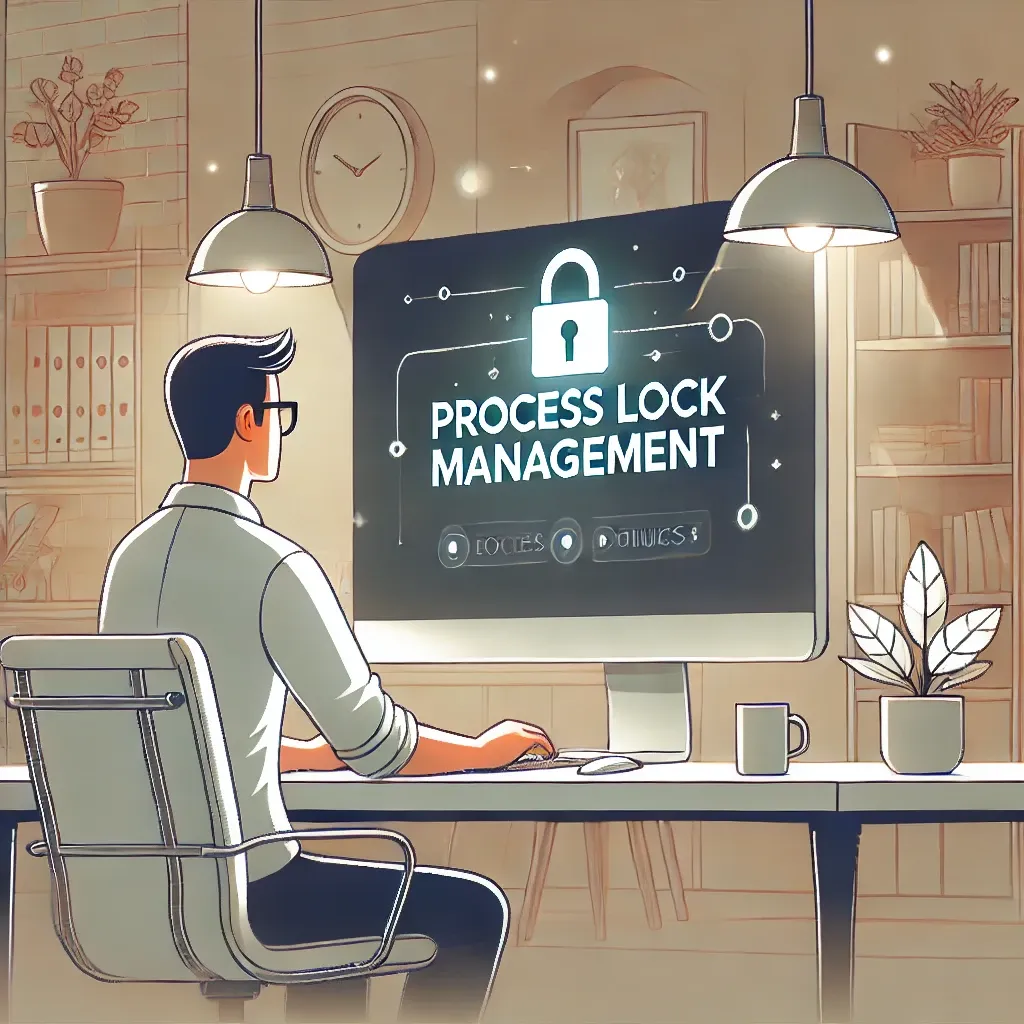
Working with locks across different processes? Laravel's Cache lock system lets you acquire, restore, and release locks seamlessly across requests and jobs! Let's explore this powerful feature.
Basic Lock Management
Here's how to handle locks between processes:
$lock = Cache::lock('processing', 120);
if ($lock->get()) {
ProcessPodcast::dispatch($podcast, $lock->owner());
}
Restoring Locks in Jobs
Restore and release the lock in your job:
Cache::restoreLock('processing', $this->owner)->release();
Real-World Example
Here's a practical implementation for processing large files:
class FileUploadController extends Controller
{
public function process(Request $request)
{
$file = $request->file('document');
$lockName = 'process-file-' . $file->hashName();
$lock = Cache::lock($lockName, 300); // 5 minutes
if ($lock->get()) {
// Initial quick validation
$this->validateFile($file);
// Dispatch processing job with lock owner
ProcessLargeFile::dispatch(
$file->store('temp'),
$lock->owner()
);
return response()->json([
'message' => 'File processing started',
'job_id' => $lockName
]);
}
return response()->json([
'message' => 'File is already being processed'
], 409);
}
}
class ProcessLargeFile implements ShouldQueue
{
public function __construct(
private string $filePath,
private string $lockOwner
) {}
public function handle()
{
try {
// Process the file
$this->processChunks();
} finally {
// Always release the lock
Cache::restoreLock('process-file', $this->lockOwner)
->release();
}
}
private function processChunks()
{
// Processing logic here
}
}
Cross-process lock management ensures your application handles concurrent operations safely and efficiently.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!