Creating Custom Casts in Laravel: Tailoring Data Transformations
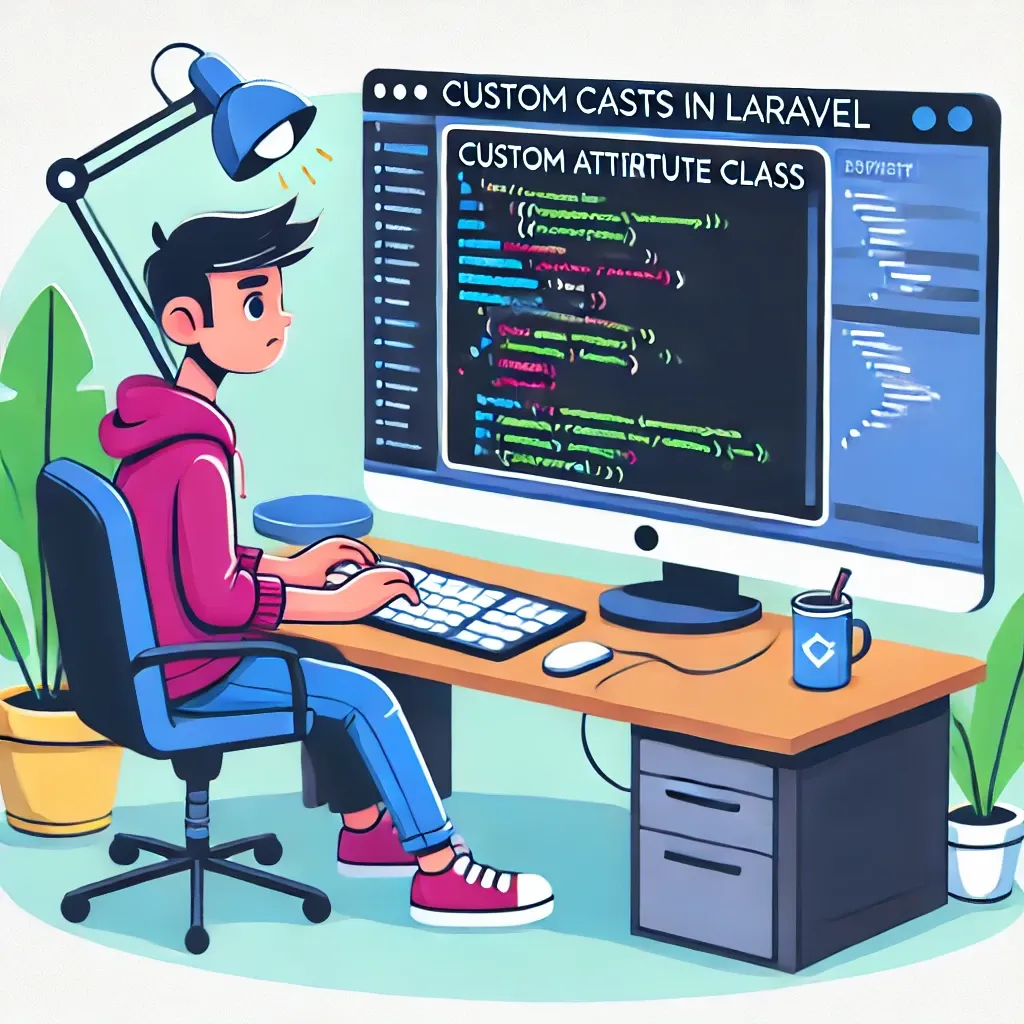
Laravel's Eloquent ORM provides a powerful casting system out of the box, but sometimes you need more specialized data transformations. This is where custom casts come into play, allowing you to define your own casting logic for model attributes.
Understanding Custom Casts
Custom casts in Laravel allow you to define how a model attribute should be transformed when it's retrieved from or stored in the database. This feature is particularly useful for complex data types or when you need to apply business-specific logic to your data transformations.
Creating a Custom Cast
To create a custom cast, you can use the Laravel Artisan command:
php artisan make:cast Json
This command generates a new cast class in your app/Casts
directory.
Implementing the Cast
Your custom cast class should implement the CastsAttributes
interface, which requires two methods: get
and set
. Here's an example of a custom JSON cast:
<?php
namespace App\Casts;
use Illuminate\Contracts\Database\Eloquent\CastsAttributes;
use Illuminate\Database\Eloquent\Model;
class Json implements CastsAttributes
{
public function get(Model $model, string $key, mixed $value, array $attributes): array
{
return json_decode($value, true);
}
public function set(Model $model, string $key, mixed $value, array $attributes): string
{
return json_encode($value);
}
}
- The
get
method transforms the raw database value into the desired format. - The
set
method prepares the value for storage in the database.
Using the Custom Cast
Once you've defined your custom cast, you can use it in your Eloquent models:
<?php
namespace App\Models;
use App\Casts\Json;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
protected $casts = [
'preferences' => Json::class,
];
}
Now, whenever you access or set the preferences
attribute, it will automatically be cast using your custom JSON cast.
Real-World Example
Let's consider a scenario where you need to store and retrieve encrypted data:
<?php
namespace App\Casts;
use Illuminate\Contracts\Database\Eloquent\CastsAttributes;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Support\Facades\Crypt;
class EncryptedAttribute implements CastsAttributes
{
public function get(Model $model, string $key, mixed $value, array $attributes): string
{
return Crypt::decryptString($value);
}
public function set(Model $model, string $key, mixed $value, array $attributes): string
{
return Crypt::encryptString($value);
}
}
You can then use this cast to automatically encrypt sensitive data:
class User extends Model
{
protected $casts = [
'secret_key' => EncryptedAttribute::class,
];
}
Conclusion
Custom casts in Laravel provide a powerful way to encapsulate complex attribute transformations. By defining your own casts, you can keep your models clean and apply consistent data transformations across your application. Whether you're dealing with JSON, encryption, or any other custom data format, custom casts offer a flexible solution for your data handling needs.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!