Create User-Friendly Password Requirement Indicators with Laravel's appliedRules() Method
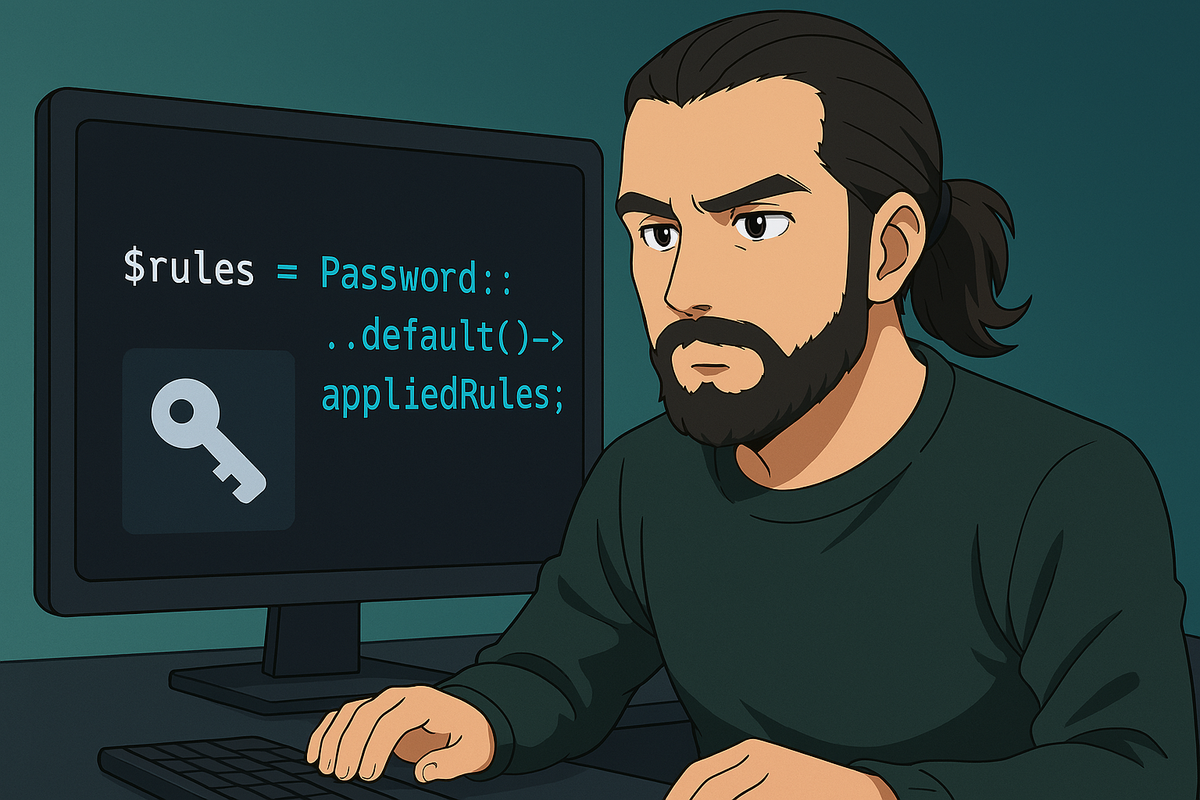
Creating user-friendly password requirement indicators just got easier with Laravel's new appliedRules() method, which provides direct access to your password validation rules for display in views.
When building registration or password reset forms, it's important to clearly communicate password requirements to users. Previously, you might have had to maintain these requirements in multiple places - once in your validation rules and again in your view. Laravel's new appliedRules() method for the Password validation builder solves this problem by providing direct access to your currently applied password rules.
Let's see how it works:
// In your controller
return view('auth.register', [
'appliedRules' => Password::default()->appliedRules(),
]);
The appliedRules() method returns an array with all available password rules and their current status (true if applied, false or a specific value if not).
Real-World Example
This method is particularly useful for creating dynamic password requirement lists that automatically stay in sync with your actual validation rules:
// In AppServiceProvider or another service provider
Password::defaults(function () {
return Password::min(8)
->mixedCase()
->numbers()
->uncompromised();
});
// In your controller
class RegisterController extends Controller
{
public function create()
{
return view('auth.register', [
'appliedRules' => Password::default()->appliedRules(),
]);
}
}
Then in your Blade view, you can dynamically display the requirements:
<div class="password-requirements">
<h4>Password must:</h4>
<ul>
<li class="{{ $appliedRules['min'] ? 'text-gray-900' : 'text-gray-400' }}">
Be at least {{ $appliedRules['min'] }} characters long
</li>
@if ($appliedRules['mixedCase'])
<li>Include both uppercase and lowercase letters</li>
@endif
@if ($appliedRules['numbers'])
<li>Include at least one number</li>
@endif
@if ($appliedRules['symbols'])
<li>Include at least one symbol</li>
@endif
@if ($appliedRules['uncompromised'])
<li>Not be found in any known data breaches</li>
@endif
</ul>
</div>
By leveraging the appliedRules() method, you can create a more coherent user experience where your interface accurately reflects your actual validation requirements.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter (https://x.com/harrisrafto), Bluesky (https://bsky.app/profile/harrisrafto.eu), and YouTube (https://www.youtube.com/@harrisrafto). It helps a lot!