Converting Values to Collections with Laravel's wrap Method
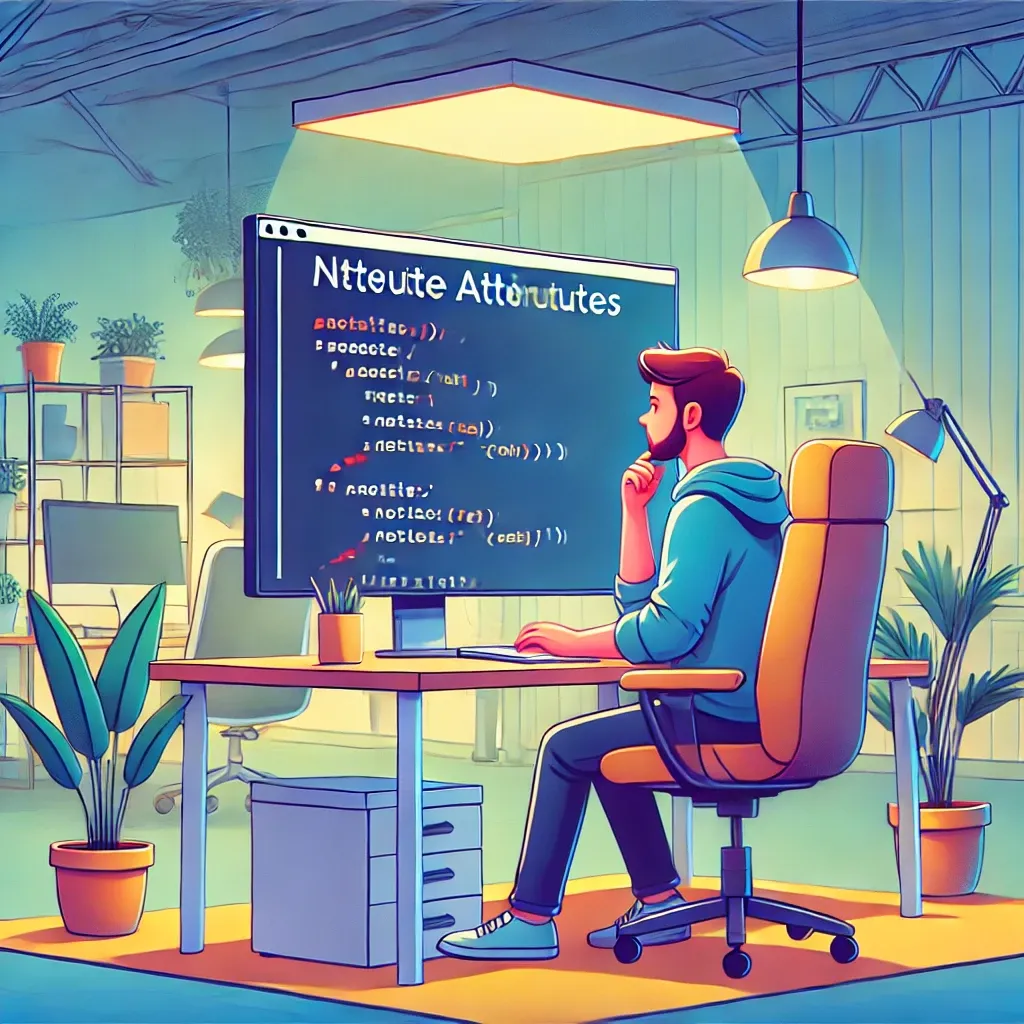
Converting values to collections becomes seamless with Laravel's Collection::wrap method. This versatile utility ensures you're always working with a collection, regardless of the input type.
Basic Usage
Wrap different types of values into collections:
use Illuminate\Support\Collection;
// Wrap a single value
$collection = Collection::wrap('John Doe');
// Result: ['John Doe']
// Wrap an array
$collection = Collection::wrap(['John Doe']);
// Result: ['John Doe']
// Wrap an existing collection
$collection = Collection::wrap(collect('John Doe'));
// Result: ['John Doe']
Real-World Example
Here's how you might use it in a data processing service:
class DataProcessor
{
public function processItems($items)
{
return Collection::wrap($items)
->map(fn($item) => $this->formatItem($item))
->filter()
->values();
}
public function addTags($entity, $tags)
{
$existingTags = $entity->tags;
$newTags = Collection::wrap($tags)
->unique()
->diff($existingTags);
$entity->tags()->attach($newTags);
return $entity;
}
public function processSearchResults($results)
{
return Collection::wrap($results)
->map(fn($result) => [
'id' => $result->id,
'title' => $result->title,
'url' => $result->url
])
->sortBy('title');
}
}
// Usage
class SearchController extends Controller
{
public function search(Request $request, DataProcessor $processor)
{
$results = SearchService::find($request->query);
return $processor->processSearchResults($results);
}
}
The wrap method ensures consistent collection handling regardless of input type, making your code more reliable and flexible.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!