Converting Special Characters with Laravel's transliterate Method
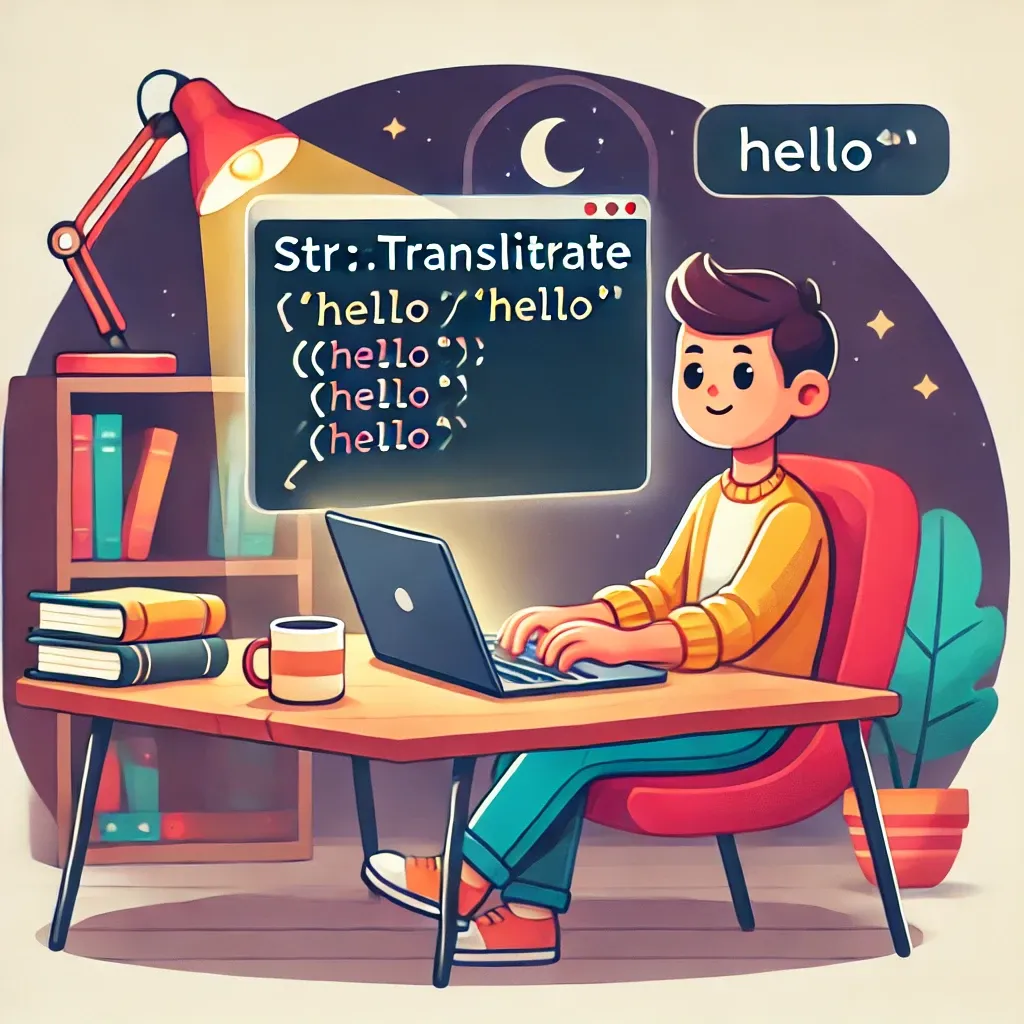
Need to convert special characters to their ASCII equivalents? Laravel's Str::transliterate method provides a simple solution for normalizing text content.
Basic Usage
Convert special characters to ASCII:
use Illuminate\Support\Str;
$email = Str::transliterate('ⓣⓔⓢⓣ@ⓛⓐⓡⓐⓥⓔⓛ.ⓒⓞⓜ');
// Result: 'test@laravel.com'
Real-World Example
Here's how you might use it in a text normalization service:
class TextNormalizer
{
public function normalizeUserInput(array $input)
{
return collect($input)->map(function ($value) {
return is_string($value)
? Str::transliterate($value)
: $value;
})->all();
}
public function sanitizeUsername(string $username)
{
return Str::transliterate($username);
}
public function cleanEmailAddress(string $email)
{
return strtolower(Str::transliterate($email));
}
public function normalizeTags(array $tags)
{
return array_map(function ($tag) {
return Str::transliterate($tag);
}, $tags);
}
}
// Usage
class UserController extends Controller
{
public function store(Request $request, TextNormalizer $normalizer)
{
$normalizedInput = $normalizer->normalizeUserInput([
'username' => $request->username,
'email' => $request->email,
'tags' => $request->tags
]);
// Create user with normalized data
User::create($normalizedInput);
}
}
The transliterate method makes it easy to ensure consistent text formatting across your application.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!