Convert Numbers to Ordinal Words in Laravel
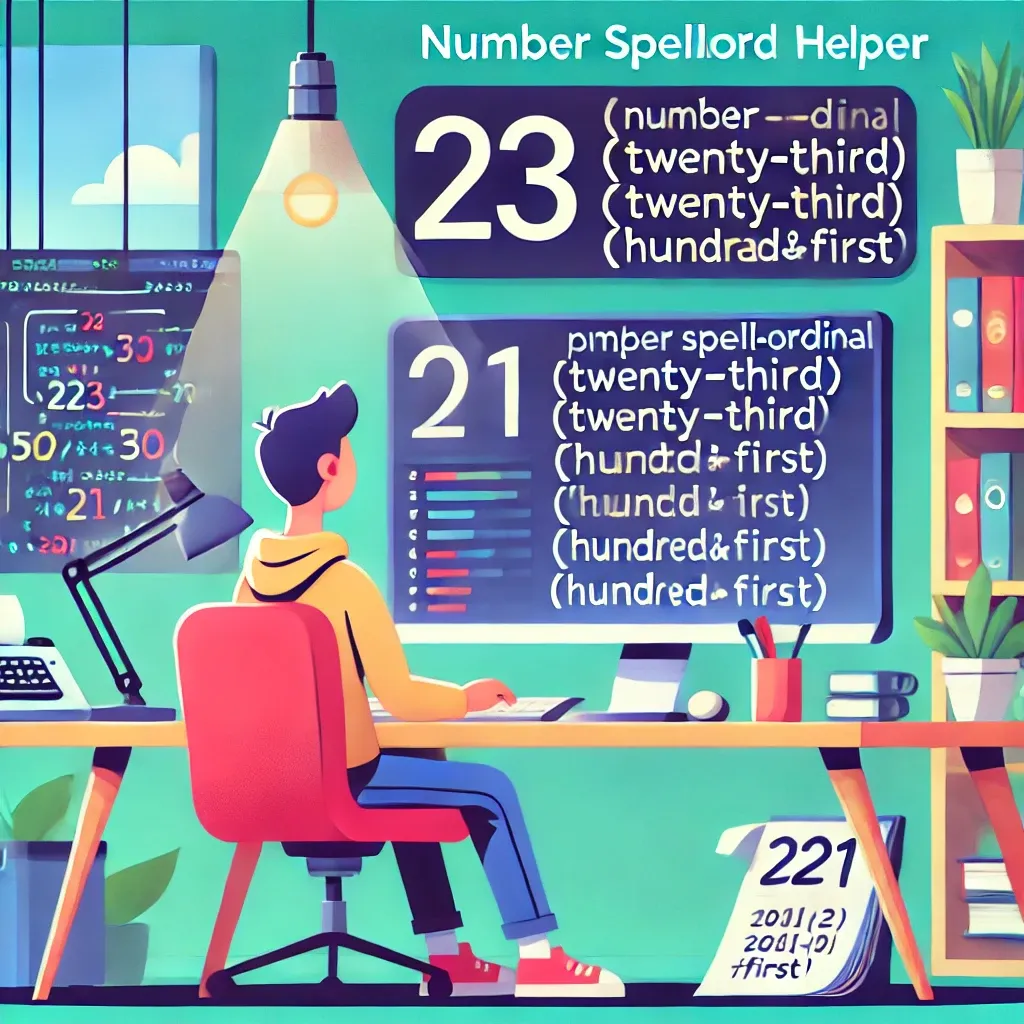
Need to spell out ordinal numbers in your Laravel app? The Number::spellOrdinal method provides a convenient way to convert numeric values into their ordinal word form.
Basic Usage
Convert numbers to ordinal words easily:
use Illuminate\Support\Number;
$title = 'The ' . Number::spellOrdinal(40) . ' president';
// Result: "The fortieth president"
Real-World Example
Here's how you might use it in a content management system:
class ChapterFormatter
{
public function formatChapterTitle(int $number, string $title): string
{
return sprintf(
'Chapter %s: %s',
Number::spellOrdinal($number),
$title
);
}
public function formatAnniversary(Carbon $date): string
{
$years = $date->diffInYears(now());
return sprintf(
'Celebrating our %s anniversary!',
Number::spellOrdinal($years)
);
}
}
class DocumentGenerator
{
public function generateBookIndex(array $chapters)
{
return collect($chapters)->map(function($chapter, $index) {
return sprintf(
'%s - %s',
Number::spellOrdinal($index + 1),
$chapter['title']
);
})->join("\n");
}
}
// Usage
$formatter = new ChapterFormatter();
echo $formatter->formatChapterTitle(3, 'The Journey Begins');
// Output: "Chapter third: The Journey Begins"
$doc = new DocumentGenerator();
$index = $doc->generateBookIndex([
['title' => 'Introduction'],
['title' => 'Getting Started'],
]);
// Output: "First - Introduction\nSecond - Getting Started"
The Number::spellOrdinal method makes it easy to create human-readable content with properly formatted ordinal numbers.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!