Convert Non-Decimal String Values with Laravel's Enhanced toInteger() Method
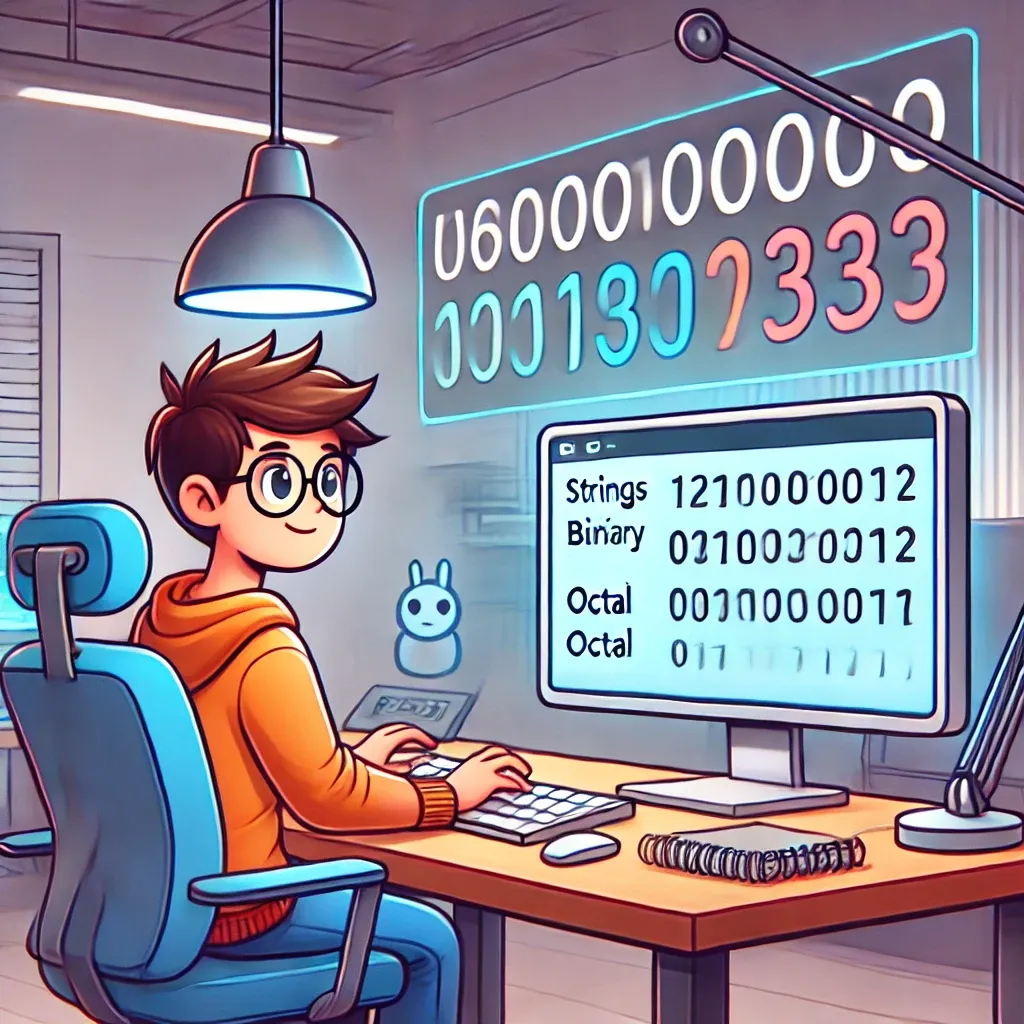
Working with hexadecimal, octal, or binary string values in Laravel? The Stringable toInteger() method now accepts a base parameter, streamlining how you convert non-decimal string values.
When manipulating strings that represent numbers in different bases, developers often need to switch between Laravel's fluent string operations and PHP's native functions. With the enhanced toInteger() method, you can now perform these conversions directly within your string manipulation chain.
Let's see how this works:
// Convert a hexadecimal string to an integer
$value = Str::of('1A')->toInteger(16); // Returns 26
// Convert a binary string to an integer
$value = Str::of('1010')->toInteger(2); // Returns 10
// Convert an octal string to an integer
$value = Str::of('777')->toInteger(8); // Returns 511
Real-World Example
This enhancement is particularly useful when processing data formats that include non-decimal numbers, such as color codes, binary data, or configuration values. Here's a practical example of processing a hexadecimal color code:
// Before: Breaking out of the fluent chain
$hexColor = '#1a2b3c';
$stringable = Str::of($hexColor)->after('#');
$colorValue = intval($stringable, 16);
// After: Staying in the fluent chain
$colorValue = Str::of($hexColor)
->after('#')
->toInteger(16);
You can also use this in more complex string manipulation scenarios:
// Extract and convert a hexadecimal value from a data string
$data = 'Config: temp=0x2A, mode=0x01';
$temperature = Str::of($data)
->between('temp=', ',') // Extract "0x2A"
->replaceFirst('0x', '') // Remove "0x" prefix
->toInteger(16); // Convert to decimal (42)
The base parameter accepts values from 2 to 36, following the same rules as PHP's native intval() function, giving you flexibility for a wide range of conversion needs.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter (https://x.com/harrisrafto), Bluesky (https://bsky.app/profile/harrisrafto.eu), and YouTube (https://www.youtube.com/@harrisrafto). It helps a lot!