Content Type Negotiation Made Easy: Laravel's prefers Method
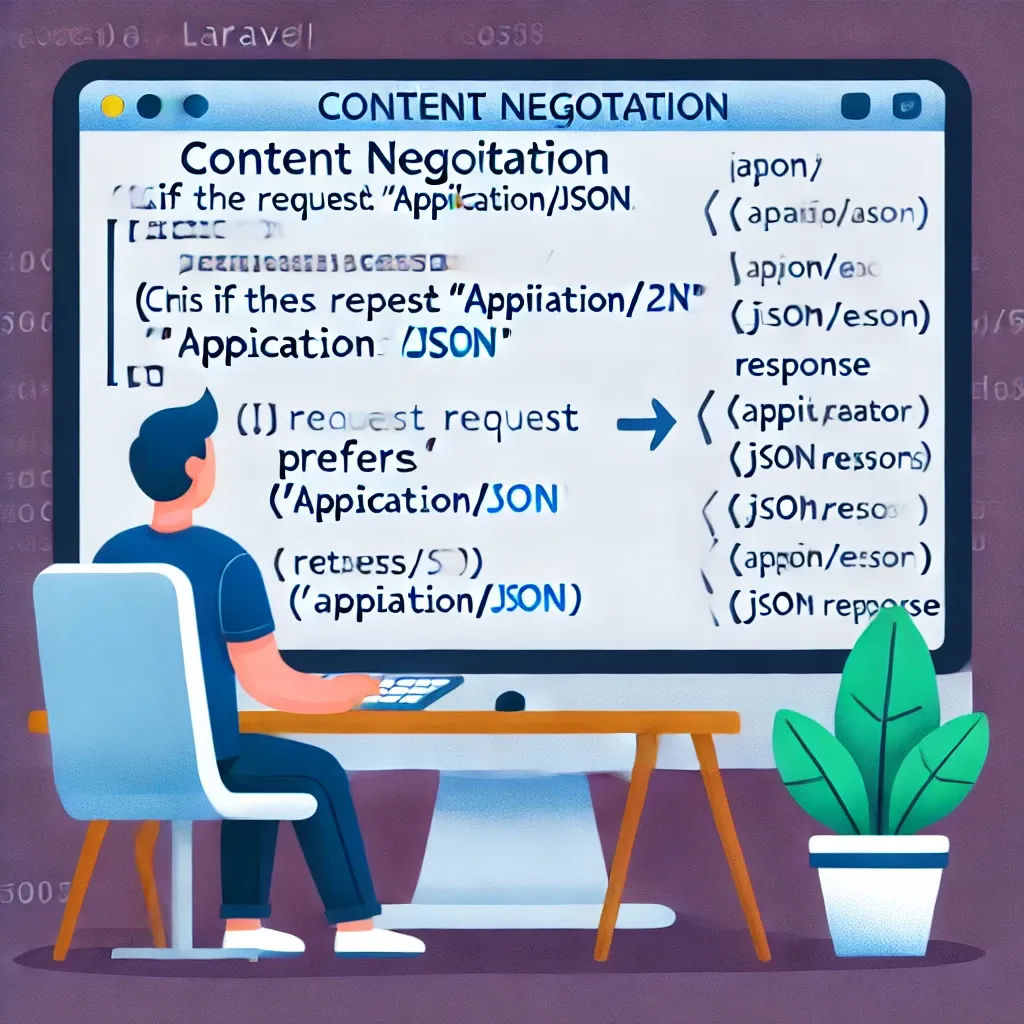
Ever needed to serve different content types based on what your client wants? Laravel's prefers
method makes content negotiation a breeze! Let's explore how this simple yet powerful feature can enhance your application's flexibility.
Understanding prefers
The prefers
method helps you determine which content type your client prefers from a given set of options:
$preferred = $request->prefers(['text/html', 'application/json']);
If the client accepts one of these types, you'll get that type back. If none are accepted, you'll get null
.
Basic Usage
Here's a simple example of how to use it:
public function show(Request $request, Post $post)
{
$preferred = $request->prefers(['text/html', 'application/json']);
if ($preferred === 'text/html') {
return view('posts.show', compact('post'));
}
if ($preferred === 'application/json') {
return response()->json($post);
}
return response('Not Acceptable', 406);
}
Real-World Example
Let's create a versatile API endpoint that can serve both web browsers and API clients:
class ArticleController extends Controller
{
public function show(Request $request, Article $article)
{
$preferred = $request->prefers([
'text/html',
'application/json',
'application/xml'
]);
return match($preferred) {
'text/html' => view('articles.show', compact('article')),
'application/json' => response()->json([
'data' => $article,
'meta' => [
'views' => $article->view_count,
'created' => $article->created_at->diffForHumans()
]
]),
'application/xml' => response()
->view('articles.xml', compact('article'))
->header('Content-Type', 'application/xml'),
default => response('Not Acceptable', 406)
};
}
}
Laravel's prefers
method simplifies content negotiation, making it easy to build flexible endpoints that can serve different types of clients effectively.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!