Consistent Model Creation with withAttributes in Laravel
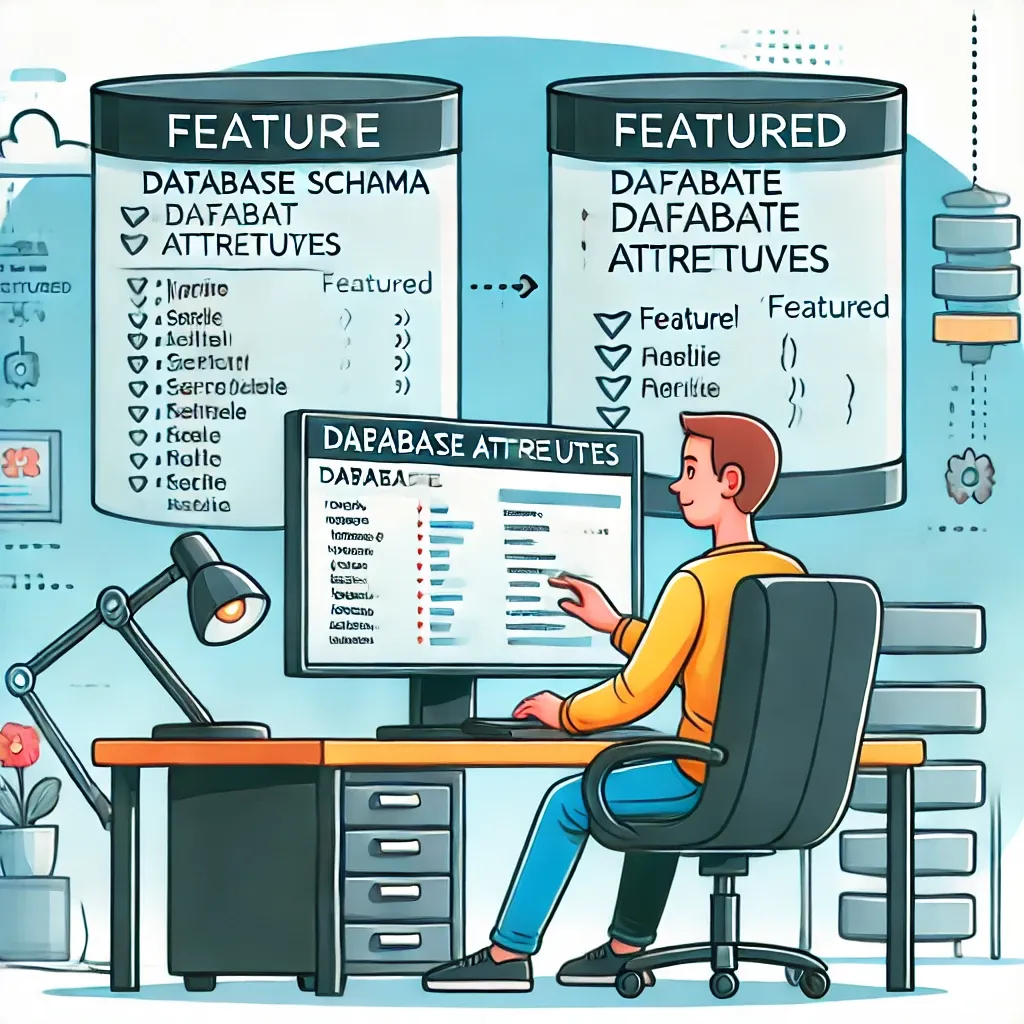
Need to ensure attributes are set when creating models through relationships? Laravel's withAttributes method provides an elegant solution for consistent model creation through relationship methods.
When working with constrained relationship methods, you often want newly created models to maintain the same constraints. Laravel's withAttributes method ensures that models created through these relationship methods automatically receive the expected attributes.
Let's see how it works:
class User extends Model
{
// Base relationship
public function posts(): HasMany
{
return $this->hasMany(Post::class);
}
// Constrained relationship with withAttributes
public function featuredPosts(): HasMany
{
return $this->posts()
->where('featured', true)
->withAttributes(['featured' => true]);
}
}
Real-World Example
Here's how you might use withAttributes in a content management system:
class Category extends Model
{
// Base products relationship
public function products(): HasMany
{
return $this->hasMany(Product::class);
}
// Published products with consistent creation
public function publishedProducts(): HasMany
{
return $this->products()
->where('status', 'published')
->withAttributes(['status' => 'published']);
}
// Featured products with consistent creation
public function featuredProducts(): HasMany
{
return $this->products()
->where('featured', true)
->withAttributes([
'featured' => true,
'featured_at' => now()
]);
}
}
Now when you create models through these constrained relationships, they'll automatically include the specified attributes:
// Create a published product
$product = $category->publishedProducts()->create([
'name' => 'New Product',
'price' => 99.99
]);
// The status will automatically be "published"
dd($product->status); // "published"
// Create a featured product
$featured = $category->featuredProducts()->create([
'name' => 'Featured Item'
]);
// Automatically gets featured attributes
dd($featured->featured); // true
dd($featured->featured_at); // current timestamp
The withAttributes method solves the common issue of attribute inconsistency when creating models through constrained relationships. It ensures that models created through filtered relationships maintain the same constraints that define the relationship.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!