Conditional Context Management Made Easy with Laravel's Context Facade
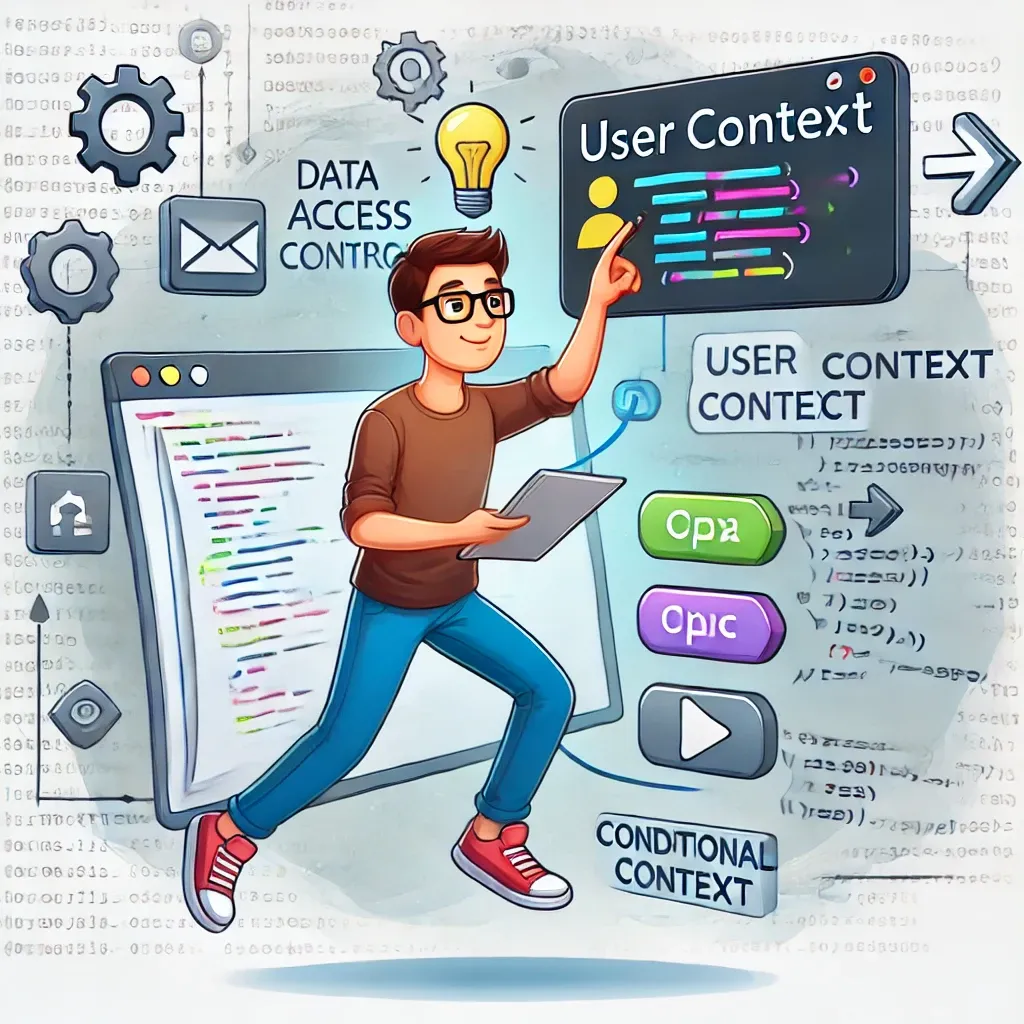
Need to handle contextual data differently based on user roles or other conditions? Laravel's Context Facade with the Conditionable trait provides an elegant solution for conditional context management.
Laravel's Context system allows you to share data across different parts of your application. With the addition of the Conditionable trait, you can now apply conditional logic to your context management, making your code more expressive and reducing unnecessary conditionals.
Let's see how it works:
Context::when(
auth()->user()->isAdmin(),
fn ($context) => $context->add('user', ['key' => 'other data', ...auth()->user()]),
fn ($context) => $context->add('user', auth()->user()),
);
This code checks if the current user is an admin. If they are, it adds the user to the context with additional data. If not, it simply adds the standard user data.
Real-World Example
The Conditionable trait makes your context management more readable with methods like when
and unless
. Here's a simpler example:
// Add different feature flags based on environment
Context::when(
app()->environment('production'),
fn ($context) => $context->add('features', ['beta' => false]),
fn ($context) => $context->add('features', ['beta' => true])
);
// Add user permissions based on role
Context::when(
auth()->user()->isAdmin(),
fn ($context) => $context->add('permissions', 'all'),
fn ($context) => $context->add('permissions', 'limited')
);
This feature is particularly useful for setting user-specific data based on roles, adding environment-specific configuration, or managing feature flags across your application.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter (https://x.com/harrisrafto), Bluesky (https://bsky.app/profile/harrisrafto.eu), and YouTube (https://www.youtube.com/@harrisrafto). It helps a lot!