Command Dependency Injection in Laravel: A Closer Look at Closure Commands
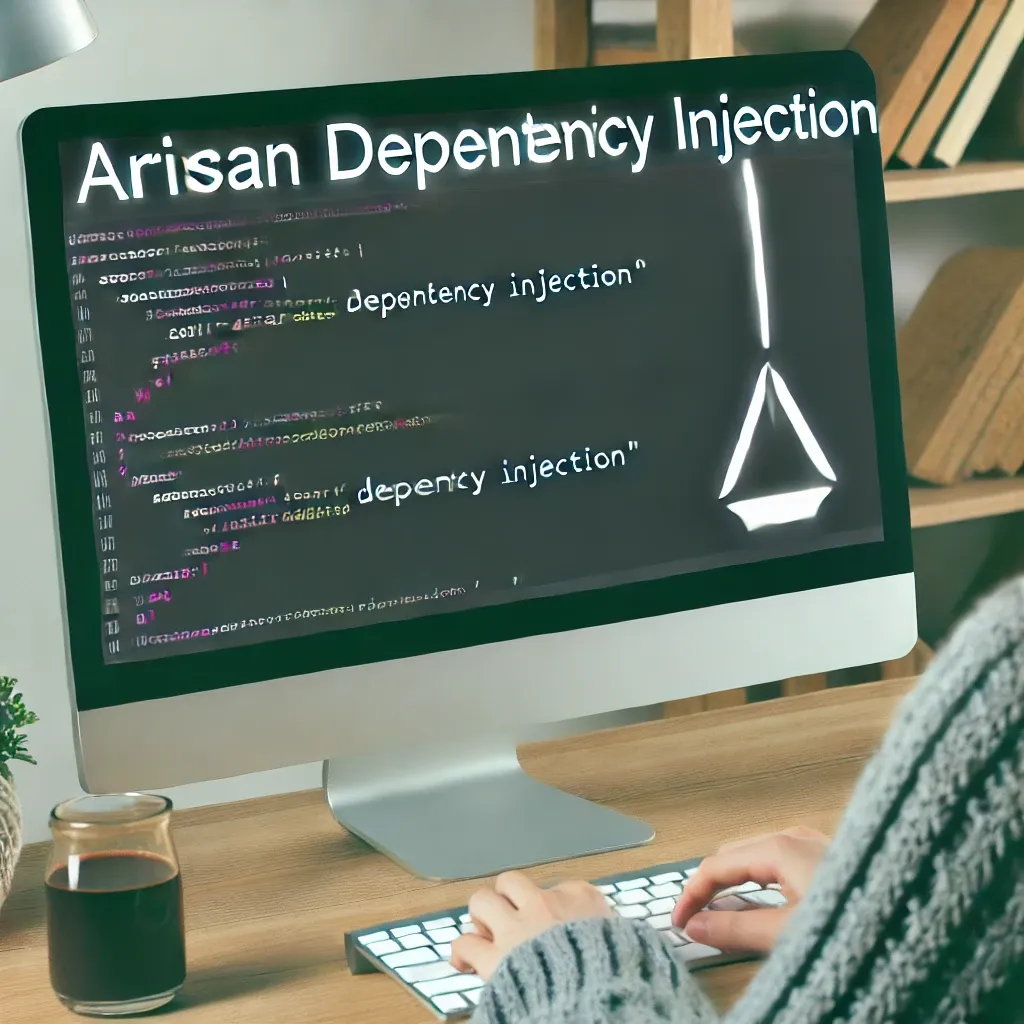
Need to inject dependencies into your Artisan command closures? Laravel makes it seamless! Let's explore how to leverage dependency injection in your command closures.
Basic Usage
Here's how you can inject dependencies along with your command arguments:
use App\Models\User;
use App\Support\DripEmailer;
Artisan::command('mail:send {user}', function (DripEmailer $drip, string $user) {
$drip->send(User::find($user));
});
Real-World Example
Here's how you might build a system notification command:
use App\Services\NotificationService;
use App\Models\User;
use App\Enums\Priority;
Artisan::command('notify:users {type} {--priority=normal}', function (
NotificationService $notifier,
string $type,
) {
$priority = Priority::from($this->option('priority'));
$users = User::whereNotNull('email')->get();
foreach ($users as $user) {
$notifier->send(
user: $user,
type: $type,
priority: $priority
);
$this->info("Notification sent to {$user->email}");
}
});
This approach lets you combine the simplicity of closure commands with the power of Laravel's dependency injection system, making your commands both concise and maintainable.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!