Cleaner Route Definitions with Laravel's Enum Support
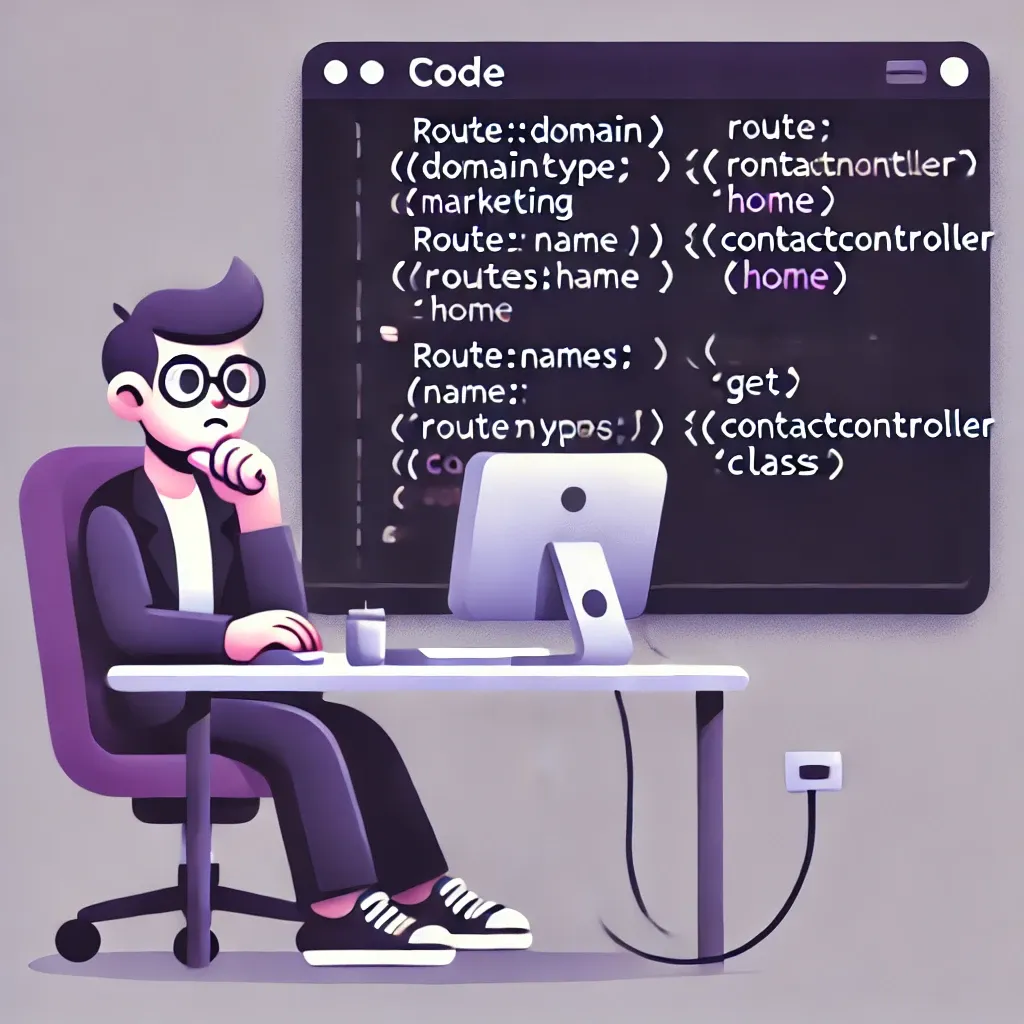
Laravel's route system just got even more elegant with direct enum support! No more calling ->value on your enums when defining routes. Let's explore this sleek improvement.
The New Way
Previously, you had to explicitly access the enum's value. Now you can pass enums directly:
// Old way
Route::domain(InterfaceDomain::Marketing->value)
->name(Routes::Home->value);
// New way
Route::domain(InterfaceDomain::Marketing)
->name(Routes::Home);
Real-World Example
Here's how this might look in a multi-tenant application:
enum DomainType: string
{
case Admin = 'admin.example.com';
case Customer = 'app.example.com';
case Marketing = 'www.example.com';
}
enum RouteNames: string
{
case Dashboard = 'dashboard';
case Profile = 'profile';
case Settings = 'settings';
}
Route::domain(DomainType::Admin)
->group(function () {
Route::get('/dashboard', AdminDashboardController::class)
->name(RouteNames::Dashboard);
Route::get('/settings', SettingsController::class)
->name(RouteNames::Settings);
});
This cleaner syntax makes your route definitions more readable and maintainable, while still leveraging the type safety of PHP's enums.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!