Case-Insensitive Pattern Matching with Laravel's Str::is Method
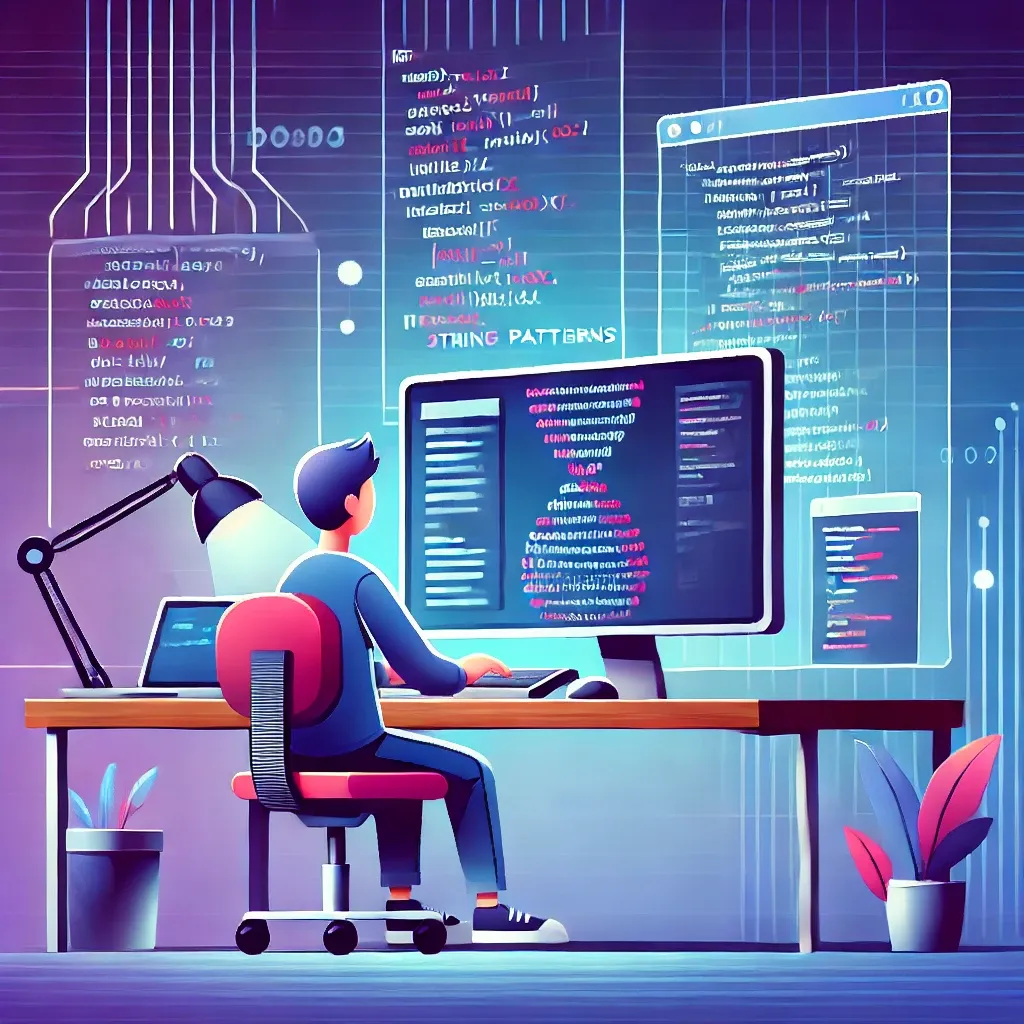
Need flexible pattern matching in your strings? Laravel's Str::is method now supports case-insensitive comparisons, making string pattern matching more versatile.
Basic Usage
Match patterns with case insensitivity:
use Illuminate\Support\Str;
// Simple string matching
Str::is('Admin', 'admin', true); // true
// Wildcard pattern matching
Str::is('*.jpg', 'photo.JPG', true); // true
// Prefix matching
Str::is('SKU123*', 'sku12345', true); // true
// Custom patterns
Str::is('prd-001*', 'PRD-001-XYZ', true); // true
// Email matching
Str::is('JOHN.DOE@example.com', 'john.doe@example.com', true); // true
Real-World Example
Here's how you might use it in a file processing system:
class FileProcessor
{
protected array $allowedExtensions = ['jpg', 'png', 'pdf'];
public function validateFile(string $filename)
{
foreach ($this->allowedExtensions as $ext) {
if (Str::is("*.{$ext}", $filename, true)) {
return true;
}
}
return false;
}
public function processUserUploads(array $files)
{
return collect($files)->filter(function ($file) {
// Match user-specific files (e.g., USER123-*.*)
return Str::is("USER*-*.*", $file, true);
});
}
public function categorizeDocument(string $filename)
{
$patterns = [
'invoice' => 'INV-*.*',
'report' => 'RPT-*.*',
'contract' => 'CTR-*.*'
];
foreach ($patterns as $type => $pattern) {
if (Str::is($pattern, $filename, true)) {
return $type;
}
}
return 'other';
}
}
// Usage
$processor = new FileProcessor();
$processor->validateFile('image.JPG'); // true
$processor->validateFile('document.PDF'); // true
$processor->categorizeDocument('INV-001.pdf'); // 'invoice'
$processor->categorizeDocument('inv-002.PDF'); // 'invoice'
The case-insensitive option in Str::is makes pattern matching more flexible and user-friendly.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!