Building Real-Time Applications with Laravel Broadcasting
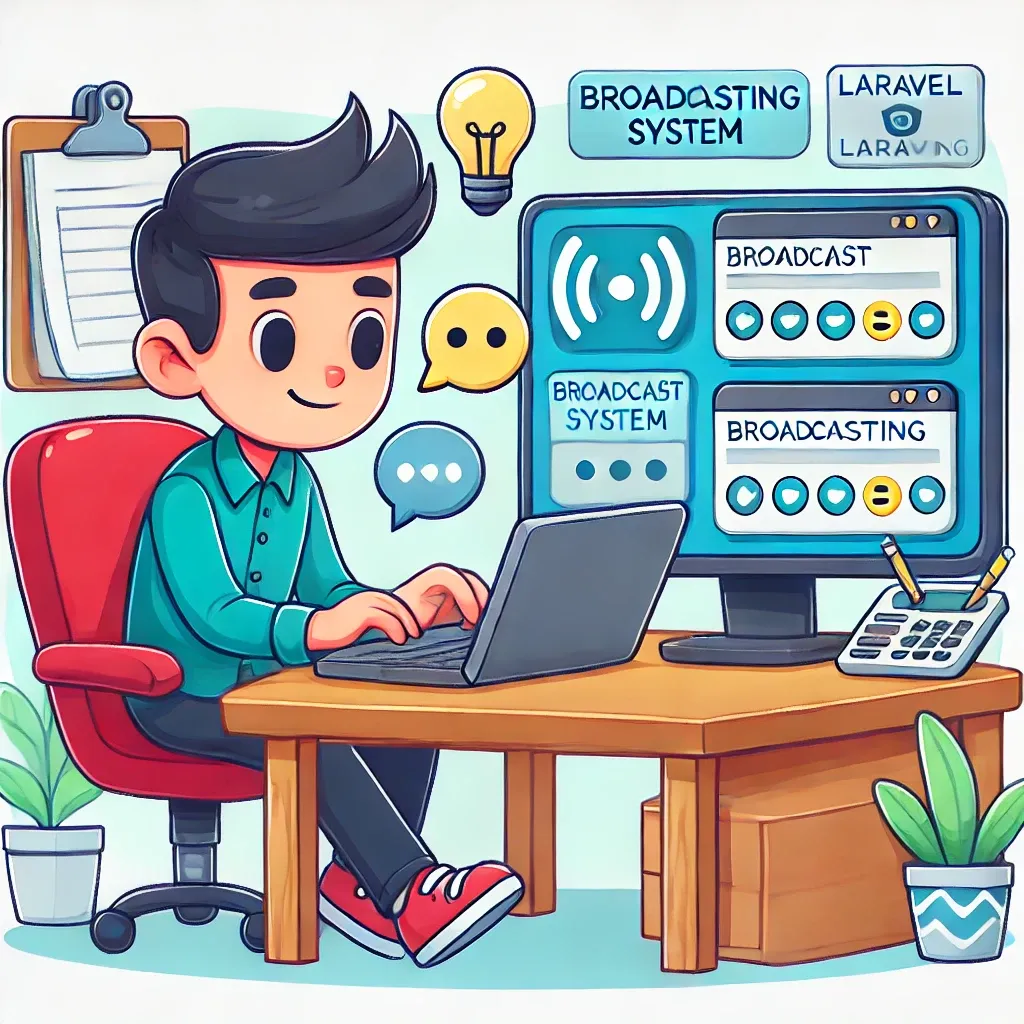
Laravel's broadcasting system provides a powerful way to add real-time features to your web applications. Whether you're building a chat application, live notifications, or real-time data updates, broadcasting can help you achieve seamless, live interactions. Let's explore how to leverage this feature in your Laravel projects.
Setting Up Broadcasting
First, configure your broadcasting driver in config/broadcasting.php
. Laravel supports several drivers like Pusher, Redis, and Socket.io.
For this example, we'll use Pusher:
'default' => env('BROADCAST_DRIVER', 'pusher'),
'connections' => [
'pusher' => [
'driver' => 'pusher',
'key' => env('PUSHER_APP_KEY'),
'secret' => env('PUSHER_APP_SECRET'),
'app_id' => env('PUSHER_APP_ID'),
'options' => [
'cluster' => env('PUSHER_APP_CLUSTER'),
'encrypted' => true,
],
],
],
Creating a Broadcastable Event
To create an event that can be broadcast:
php artisan make:event OrderShipped
Modify the event class:
use Illuminate\Broadcasting\Channel;
use Illuminate\Contracts\Broadcasting\ShouldBroadcast;
class OrderShipped implements ShouldBroadcast
{
use Dispatchable, InteractsWithSockets, SerializesModels;
public $order;
public function __construct(Order $order)
{
$this->order = $order;
}
public function broadcastOn()
{
return new PrivateChannel('orders.' . $this->order->user_id);
}
public function broadcastWith()
{
return [
'order_id' => $this->order->id,
'total' => $this->order->total,
];
}
}
Broadcasting the Event
To broadcast the event:
event(new OrderShipped($order));
Authorizing Channels
For private channels, you need to authorize access. In routes/channels.php
:
Broadcast::channel('orders.{userId}', function ($user, $userId) {
return $user->id === (int) $userId;
});
Listening for Events on the Client
Use Laravel Echo to listen for events on the client side:
import Echo from 'laravel-echo';
window.Pusher = require('pusher-js');
window.Echo = new Echo({
broadcaster: 'pusher',
key: process.env.MIX_PUSHER_APP_KEY,
cluster: process.env.MIX_PUSHER_APP_CLUSTER,
encrypted: true
});
Echo.private(`orders.${userId}`)
.listen('OrderShipped', (e) => {
console.log(e.order_id);
});
Presence Channels
For features like showing online users, use presence channels:
public function broadcastOn()
{
return new PresenceChannel('room.' . $this->roomId);
}
On the client:
Echo.join(`room.${roomId}`)
.here((users) => {
// Users currently in the channel
})
.joining((user) => {
console.log(user.name + ' joined the room');
})
.leaving((user) => {
console.log(user.name + ' left the room');
});
Broadcasting to Multiple Channels
You can broadcast to multiple channels:
public function broadcastOn()
{
return [
new PrivateChannel('orders.' . $this->order->user_id),
new Channel('orders'),
];
}
Customizing Event Broadcasting
You can customize the event name and socket ID:
public function broadcastAs()
{
return 'server.created';
}
public function broadcastWith()
{
return ['id' => $this->server->id];
}
Broadcasting and Queues
For better performance, you can queue your broadcast events:
class OrderShipped implements ShouldBroadcast, ShouldQueue
{
// ...
}
Testing Broadcasting
You can fake broadcasting in your tests:
use Illuminate\Support\Facades\Event;
public function testOrderShipping()
{
Event::fake();
// Perform order shipping...
Event::assertDispatched(OrderShipped::class);
}
Laravel's broadcasting system, combined with Laravel Echo, provides a powerful toolset for adding real-time features to your applications. Whether you're building a chat application, live notifications, or real-time dashboards, broadcasting can help you create dynamic, responsive user experiences.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!