Bootstrapping Your Laravel App with Database Seeding
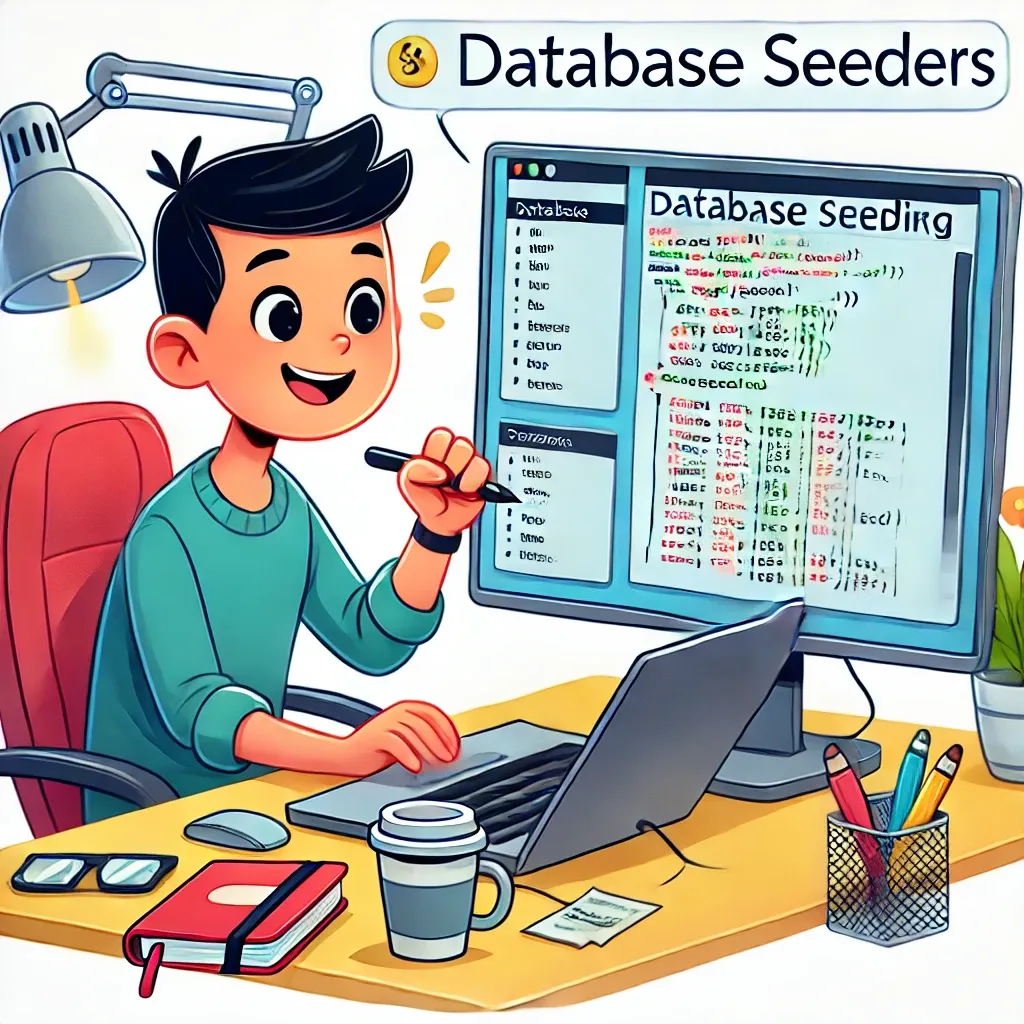
Database seeding is a crucial feature in Laravel that allows developers to populate their database with initial or test data. This process is essential for creating consistent development environments, setting up demo data, and facilitating robust testing. Let's dive into how you can leverage Laravel's seeding capabilities effectively.
Basic Seeder Structure
To create a seeder, use Laravel's Artisan command:
php artisan make:seeder UserSeeder
This generates a new seeder class:
use Illuminate\Database\Seeder;
class UserSeeder extends Seeder
{
public function run()
{
// Seeding logic here
}
}
Simple Data Insertion
For basic data insertion, you can use the DB facade:
use Illuminate\Support\Facades\DB;
public function run()
{
DB::table('users')->insert([
'name' => 'John Doe',
'email' => 'john@example.com',
'password' => bcrypt('password'),
]);
}
Using Model Factories
Model factories provide a more flexible and powerful way to generate test data:
use App\Models\User;
public function run()
{
User::factory()->count(50)->create();
}
Seeding Relationships
You can seed related data using factories:
use App\Models\User;
use App\Models\Post;
public function run()
{
User::factory(10)->create()->each(function ($user) {
$user->posts()->saveMany(
Post::factory(3)->make()
);
});
}
Calling Seeders from DatabaseSeeder
In your DatabaseSeeder
, you can call other seeders:
class DatabaseSeeder extends Seeder
{
public function run()
{
$this->call([
UserSeeder::class,
PostSeeder::class,
CommentSeeder::class,
]);
}
}
Conditional Seeding
You can add conditions to your seeders for different environments:
public function run()
{
if (app()->environment('local')) {
User::factory()->count(100)->create();
} else {
User::factory()->count(10)->create();
}
}
Using Faker for Realistic Data
Laravel's factories use Faker to generate realistic data:
$factory->define(User::class, function (Faker $faker) {
return [
'name' => $faker->name,
'email' => $faker->unique()->safeEmail,
'password' => bcrypt('password'),
];
});
Running Seeders
To run your seeders:
php artisan db:seed
# Or to run a specific seeder
php artisan db:seed --class=UserSeeder
Database seeding in Laravel provides a powerful way to set up your application with consistent, realistic data. By leveraging seeders and factories, you can create robust testing environments, demonstrate your application's capabilities, and ensure that all developers on your team are working with the same baseline data.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!