Boost your app's speed with Laravel Caching
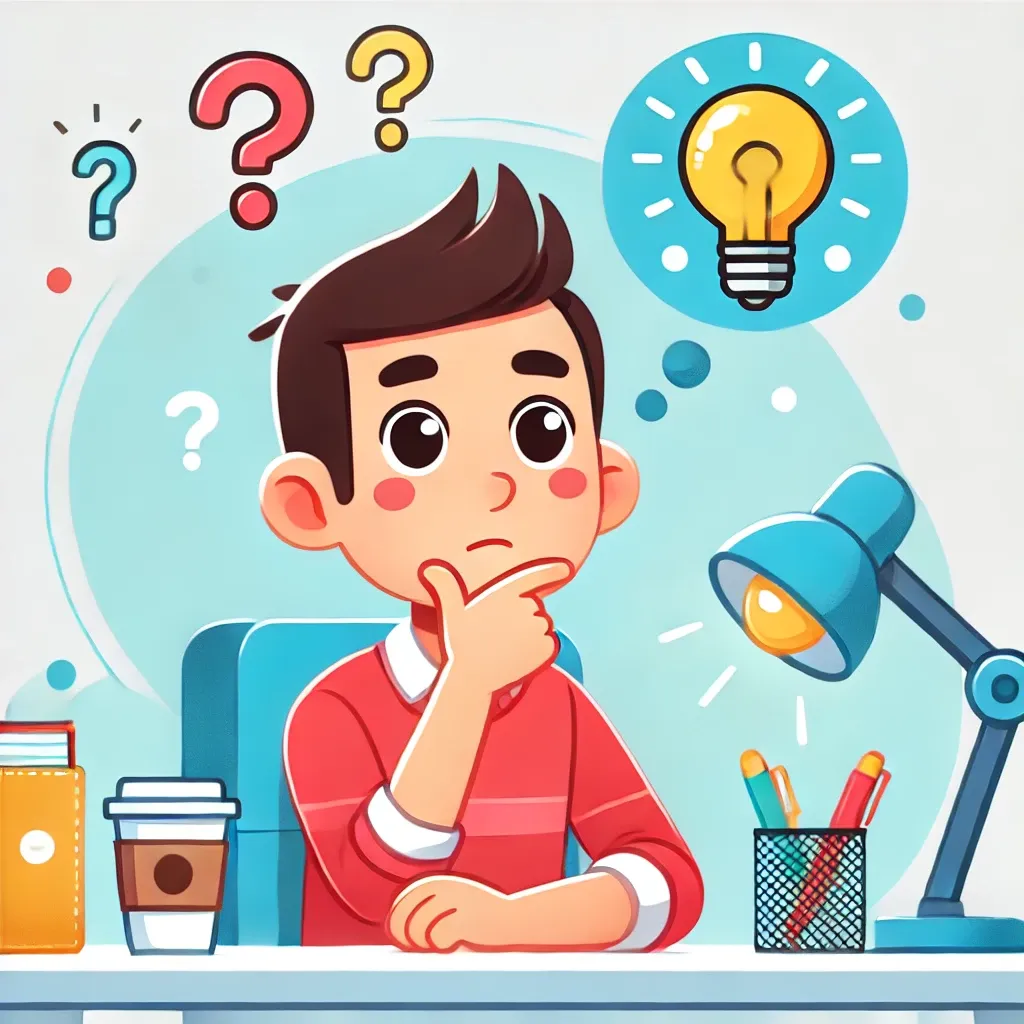
Hey Laravel devs, here is a gem for you! 💎
When it comes to web applications, speed is essential. Users expect fast and responsive experiences, and one way to achieve this is through efficient caching mechanisms. In this blog post, we'll explore how to use Laravel's Cache::remember()
method to store and retrieve data efficiently, helping you reduce database load and improve performance.
What is Caching?
Caching is the process of storing data in a temporary storage area (cache) so that it can be accessed quickly. Instead of querying the database every time data is needed, the application first checks if the data is available in the cache. If it is, the data is retrieved from the cache, which is much faster. If not, the data is fetched from the database and then stored in the cache for future requests.
Benefits of Caching
Using caching in your Laravel application offers several benefits:
- Improved Performance: By reducing the number of database queries, caching helps speed up response times, making your application more responsive.
- Reduced Database Load: Caching decreases the load on your database, which can be particularly beneficial for high-traffic applications.
- Cost Efficiency: Lowering database usage can lead to cost savings, especially if you're using cloud-based database services.
Using Cache::remember()
in Laravel
Laravel provides a simple and elegant way to implement caching using the Cache::remember()
method. This method attempts to retrieve an item from the cache. If the item does not exist, it executes the given closure and stores the result in the cache.
Here’s how you can use Cache::remember()
to cache a list of users for 60 minutes:
$users = Cache::remember('users', 60, function () {
return DB::table('users')->get();
});
In this example, the Cache::remember()
method checks if the users
key exists in the cache. If it does, the cached data is returned. If not, the closure is executed, fetching the data from the database and storing it in the cache for 60 minutes.
Real-Life Example
Let's consider a real-life example. Imagine you are building an e-commerce platform where the list of available products is frequently accessed by users. Fetching this list from the database every time can be resource-intensive and slow, especially as the number of products grows.
By caching the product list, you can significantly improve the performance of your application. Here’s how you might implement this:
$products = Cache::remember('products', 60, function () {
return DB::table('products')->where('available', 1)->get();
});
In this scenario, the products
key is used to store the list of available products. This list is cached for 60 minutes, meaning that subsequent requests within this period will retrieve the data from the cache rather than querying the database. This reduces the load on your database and speeds up the response time for your users.
Pro Tip
Choose an appropriate expiration time for your cached data to balance between performance and freshness. For example, caching user data for 60 minutes might be ideal in scenarios where user information does not change frequently.
Implementing caching in your Laravel application is a straightforward and effective way to enhance performance and reduce database load. By using the Cache::remember()
method, you can ensure that your app is quick and efficient, providing a better experience for your users.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!