Boost Real-Time Interactivity with wire:stream in Laravel Livewire
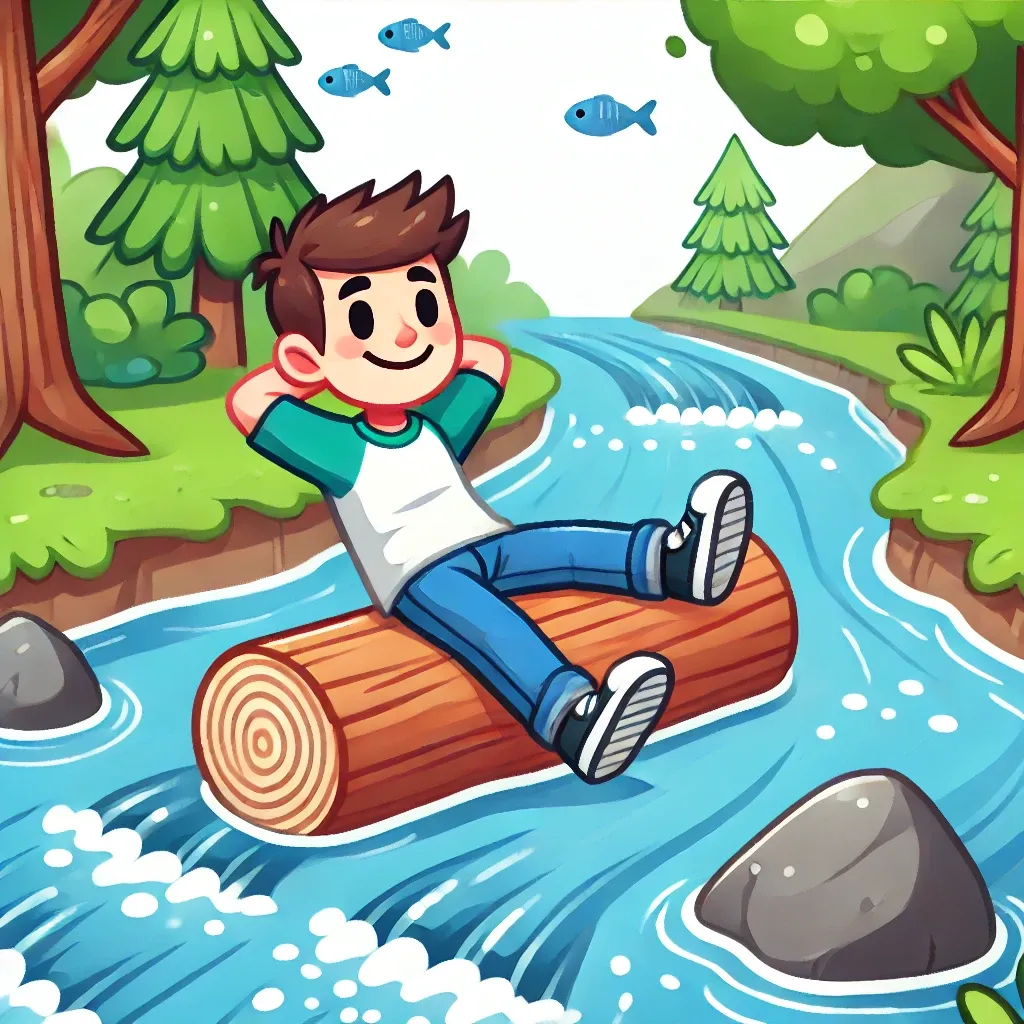
Real-time interactivity is crucial for modern web applications, enhancing user engagement and providing up-to-date information without requiring full page reloads. Laravel Livewire v3 introduces wire:stream
, a powerful feature that allows you to handle real-time updates and push data to your components efficiently. This feature is perfect for applications that require live notifications, chat functionalities, or any form of real-time data streaming.
Understanding wire:stream
The wire:stream
directive in Livewire enables components to receive real-time updates directly from the server. This allows for more dynamic and interactive user interfaces, as changes can be pushed to the client side instantly without needing full re-renders of the component.
Basic Usage
Here’s a basic example to illustrate how wire:stream
works:
<!-- Blade Template -->
<div wire:stream>
@foreach ($notifications as $notification)
<div>{{ $notification }}</div>
@endforeach
</div>
In this example, the wire:stream
directive listens for updates and automatically pushes new notifications to the component in real-time.
Real-Life Example
Consider a scenario where you have a live notifications component. Using wire:stream
, you can push new notifications to users as they arrive, ensuring they receive updates instantly.
- Livewire Component:
namespace App\Http\Livewire;
use Livewire\Component;
class CountDown extends Component
{
public $start = 3;
public function begin()
{
while ($this->start >= 0) {
// Stream the current count to the browser...
$this->stream(
to: 'count',
content: $this->start,
replace: true,
);
// Pause for 1 second between numbers...
sleep(1);
// Decrement the counter...
$this->start = $this->start - 1;
}
}
public function render()
{
return <<<'HTML'
<div>
<button wire:click="begin">Start count-down</button>
<h1>Count: <span wire:stream="count">{{ $start }}</span></h1>
</div>
HTML;
}
}
- Blade Template:
<!-- Blade Template (livewire/count-down.blade.php) -->
<div>
<button wire:click="begin">Start count-down</button>
<h1>Count: <span wire:stream="count">{{ $start }}</span></h1>
</div>
In this example, the CountDown
component updates the count value in real-time and streams it to the browser using the wire:stream
directive. The count is displayed within an h1
tag and updated every second.
Pro Tip
For an even more responsive experience, combine wire:stream
with other Livewire features like wire:poll
to handle scenarios where real-time updates might be intermittent or require regular polling to ensure consistency.
<!-- Blade Template with Polling -->
<div wire:stream wire:poll.5s>
<h1>Count: <span wire:stream="count">{{ $start }}</span></h1>
</div>
In this setup, the component will also poll the server every 5 seconds, ensuring that any missed updates are fetched and displayed.
Conclusion
The wire:stream
directive in Laravel Livewire is a powerful tool for enhancing real-time interactivity in your web applications. By leveraging this feature, you can provide a more dynamic and engaging user experience, keeping your users updated with the latest information without the need for full page reloads.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!