Boost performance with database query caching in Laravel
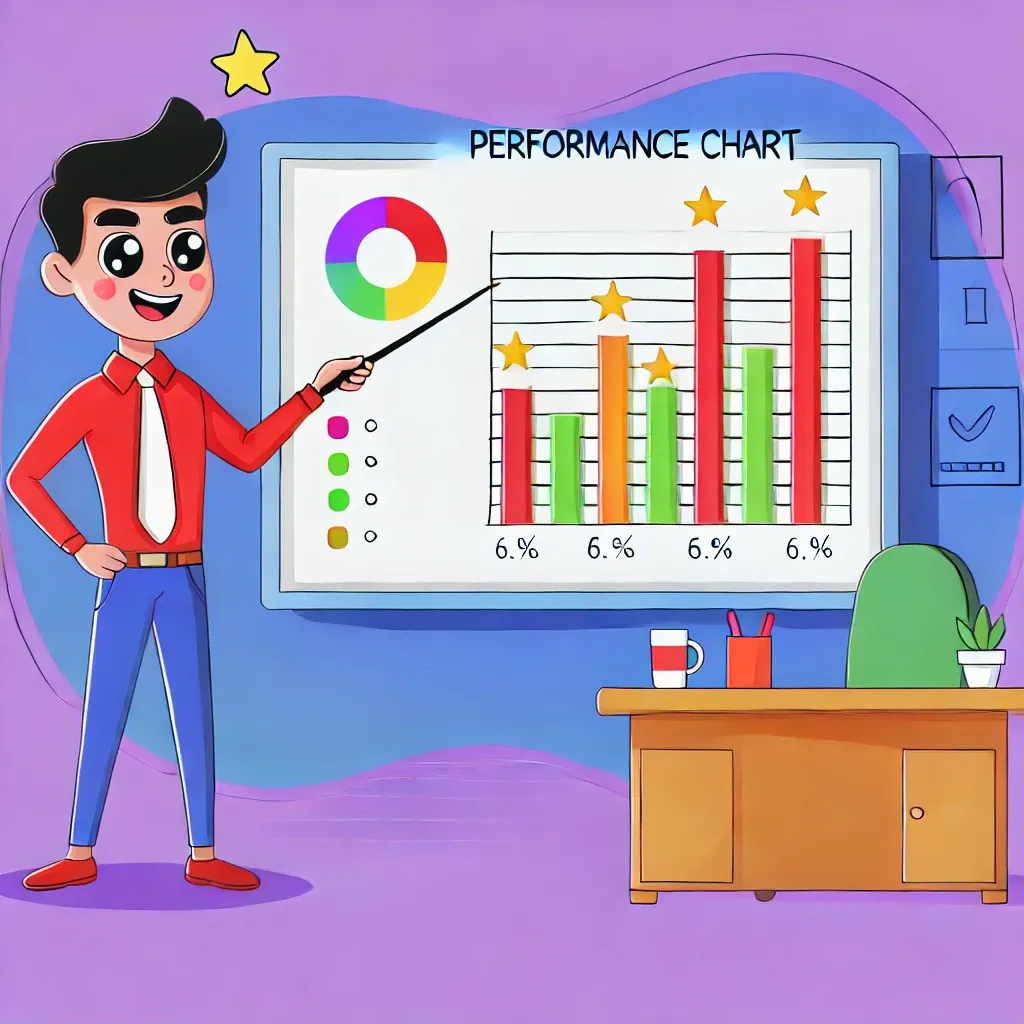
Hey Laravel devs, here is a gem for you! 💎
Database query caching is a powerful technique to optimize the performance of your Laravel applications. By caching frequently accessed data, you can significantly reduce the load on your database and speed up your application. In this blog post, we’ll explore how to use Laravel's Cache::tags()
method to implement fine-grained control over your caching strategy.
Why Use Database Query Caching?
Database queries can be expensive, especially when dealing with complex joins, aggregations, or large datasets. Caching the results of these queries can save valuable processing time and resources.
Benefits of Query Caching
Using query caching in your Laravel application offers several advantages:
- Improved Performance: Reduce the time it takes to retrieve data by serving it from the cache.
- Reduced Database Load: Decrease the number of queries hitting your database, which can improve its overall performance and stability.
- Fine-Grained Control: With
Cache::tags()
, you can cache related data sets and manage them efficiently.
How to Use Cache::tags()
in Laravel
Laravel provides a flexible and easy-to-use caching system. Here’s how you can use Cache::tags()
to cache database queries:
Caching with Tags
The Cache::tags()
method allows you to group related cache items together and manage them as a unit. This is particularly useful when you want to clear multiple related cache items at once.
Here’s an example of how to cache a list of users and their roles:
$users = Cache::tags(['users', 'roles'])->remember('users_list', 60, function () {
return DB::table('users')->get();
});
In this example, the users
and roles
tags are used to group the cached users_list
. The query result is cached for 60 minutes. If the data is not found in the cache, the closure is executed to fetch the data from the database and store it in the cache.
Real-Life Example
Imagine you are building an admin dashboard where you frequently need to display a list of users along with their roles. Fetching this data from the database every time can be slow and resource-intensive.
By using query caching, you can improve the performance of your dashboard. Here’s how you might implement this:
- Cache the Data: Use the
Cache::tags()
method to cache the list of users and their roles. - Display the Data: Retrieve the cached data when needed, ensuring that the dashboard loads quickly.
- Clear the Cache: If you update the users or roles, clear the relevant cache tags to ensure that the cached data is updated.
Example:
// Caching the users and roles
$users = Cache::tags(['users', 'roles'])->remember('users_list', 60, function () {
return DB::table('users')->get();
});
// Clearing the cache when data changes
Cache::tags(['users', 'roles'])->flush();
Pro Tip
When using cache tags, remember to clear the cache whenever you make changes to the related data. This ensures that your application always serves the most up-to-date information.
Using database query caching in Laravel can significantly enhance the performance of your application. By caching frequently accessed data and using cache tags for fine-grained control, you can reduce database load and improve response times. Start leveraging Laravel's caching capabilities today to optimize your applications!
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!