Automating Report Generation and Distribution with Laravel's Task Scheduler
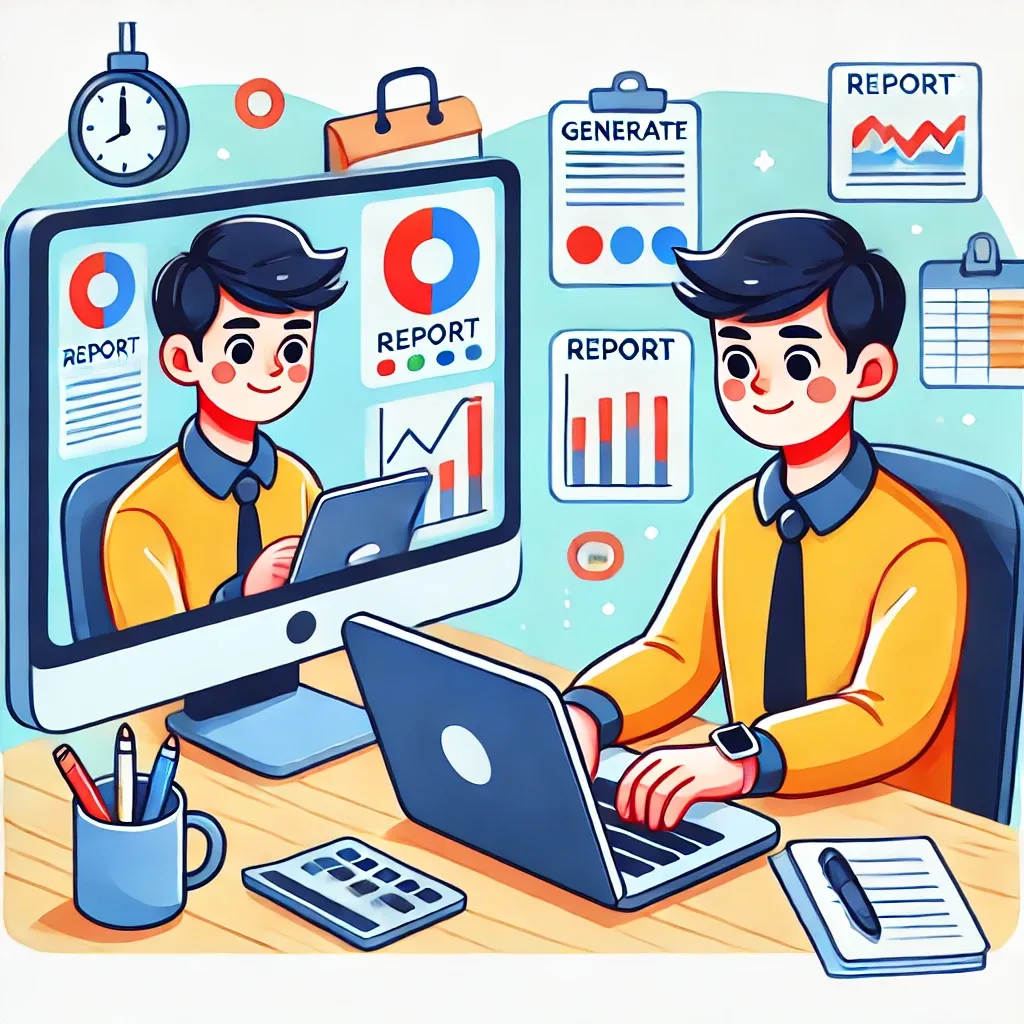
Laravel's Task Scheduler provides a powerful way to automate recurring tasks, including the generation and distribution of reports. Let's explore how to set up automated reporting in your Laravel application.
Basic Setup
First, let's set up a basic scheduled task for report generation in your app/Console/Kernel.php
file:
protected function schedule(Schedule $schedule)
{
$schedule->call(function () {
$report = Report::generate();
Mail::to('team@example.com')->send(new WeeklyReportMail($report));
})->weekly()->mondays()->at('9:00');
}
This schedule generates a report and emails it every Monday at 9:00 AM.
Creating a Dedicated Command
For more complex reports, it's better to create a dedicated Artisan command:
php artisan make:command GenerateWeeklyReport
In the new command file:
class GenerateWeeklyReport extends Command
{
protected $signature = 'report:weekly';
protected $description = 'Generate and send weekly report';
public function handle()
{
$report = Report::generateWeekly();
Mail::to('team@example.com')->send(new WeeklyReportMail($report));
$this->info('Weekly report sent successfully.');
}
}
Then, schedule this command:
$schedule->command('report:weekly')->weekly()->mondays()->at('9:00');
Flexible Report Generation
You can make your report generation more flexible:
class GenerateReport extends Command
{
protected $signature = 'report:generate {type : The type of report} {--email= : Email to send the report to}';
public function handle()
{
$type = $this->argument('type');
$email = $this->option('email') ?? 'default@example.com';
$report = Report::generate($type);
Mail::to($email)->send(new ReportMail($report, $type));
$this->info("$type report sent to $email");
}
}
Schedule multiple report types:
$schedule->command('report:generate daily --email=daily@example.com')
->dailyAt('23:00');
$schedule->command('report:generate weekly --email=weekly@example.com')
->weekly()->fridays()->at('18:00');
$schedule->command('report:generate monthly --email=monthly@example.com')
->monthlyOn(1, '9:00');
Handling Long-Running Reports
For reports that take a long time to generate:
$schedule->command('report:generate quarterly')
->quarterly()
->runInBackground()
->withoutOverlapping();
Conditional Report Generation
Generate reports based on conditions:
$schedule->command('report:generate sales')
->dailyAt('23:55')
->when(function () {
return Sales::where('created_at', '>=', now()->startOfDay())->count() > 0;
});
Report Output Handling
Save report output for auditing:
$schedule->command('report:generate audit')
->monthly()
->appendOutputTo(storage_path('logs/audit-reports.log'));
Notifications on Completion
Notify your team when reports are ready:
$schedule->command('report:generate monthly')
->monthlyOn(1, '9:00')
->thenPing('https://beats.envoyer.io/heartbeat/xyz');
By leveraging Laravel's Task Scheduler, you can create a robust, automated reporting system that keeps your team informed with minimal manual intervention. This approach ensures consistent, timely reports and frees up your team to focus on analyzing the data rather than generating it.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!