Automating Database Backups with Laravel's Task Scheduler and Cloud Storage
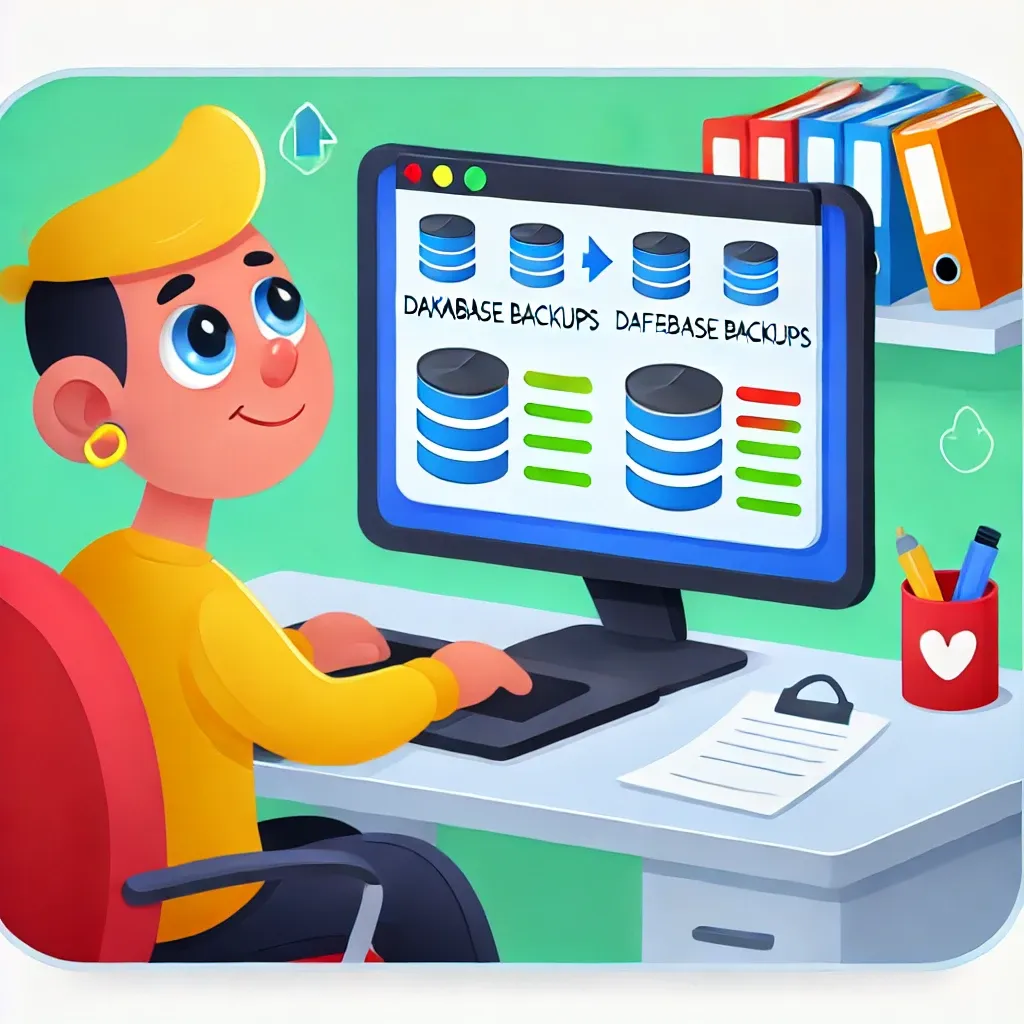
Ensuring regular database backups is crucial for any application. Laravel's Task Scheduler combined with cloud storage provides an efficient way to automate this process. Let's explore how to implement automated database backups using Laravel and store them in the cloud.
Setting Up the Backup Command
First, create a custom Artisan command for the backup process:
php artisan make:command DatabaseBackup
In the newly created command file:
namespace App\Console\Commands;
use Illuminate\Console\Command;
use Illuminate\Support\Facades\Storage;
class DatabaseBackup extends Command
{
protected $signature = 'db:backup';
protected $description = 'Backup the database to cloud storage';
public function handle()
{
$filename = "backup-" . now()->format('Y-m-d') . ".sql";
$path = storage_path("app/backup/{$filename}");
// Create the backup
$command = "mysqldump --user=" . env('DB_USERNAME') ." --password=" . env('DB_PASSWORD')
. " --host=" . env('DB_HOST') . " " . env('DB_DATABASE')
. " > " . $path;
exec($command);
// Upload to cloud storage (e.g., AWS S3)
Storage::disk('s3')->put("backups/{$filename}", file_get_contents($path));
unlink($path); // Remove local copy
$this->info('Database backup uploaded to S3.');
}
}
Scheduling the Backup
Schedule the backup command in app/Console/Kernel.php
:
protected function schedule(Schedule $schedule)
{
$schedule->command('db:backup')->daily()->at('01:00');
}
This schedules the backup to run daily at 1:00 AM.
Cloud Storage Configuration
Ensure your cloud storage is configured in config/filesystems.php
:
's3' => [
'driver' => 's3',
'key' => env('AWS_ACCESS_KEY_ID'),
'secret' => env('AWS_SECRET_ACCESS_KEY'),
'region' => env('AWS_DEFAULT_REGION'),
'bucket' => env('AWS_BUCKET'),
'url' => env('AWS_URL'),
'endpoint' => env('AWS_ENDPOINT'),
],
Don't forget to set the corresponding environment variables in your .env
file.
By implementing this setup, you ensure that your database is regularly backed up and securely stored in the cloud. This approach provides an extra layer of data protection and makes disaster recovery more manageable.
Remember to test your backup process thoroughly and periodically verify the integrity of your backups to ensure they're valid and can be restored if needed.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!