Accessing Command Arguments and Options in Laravel
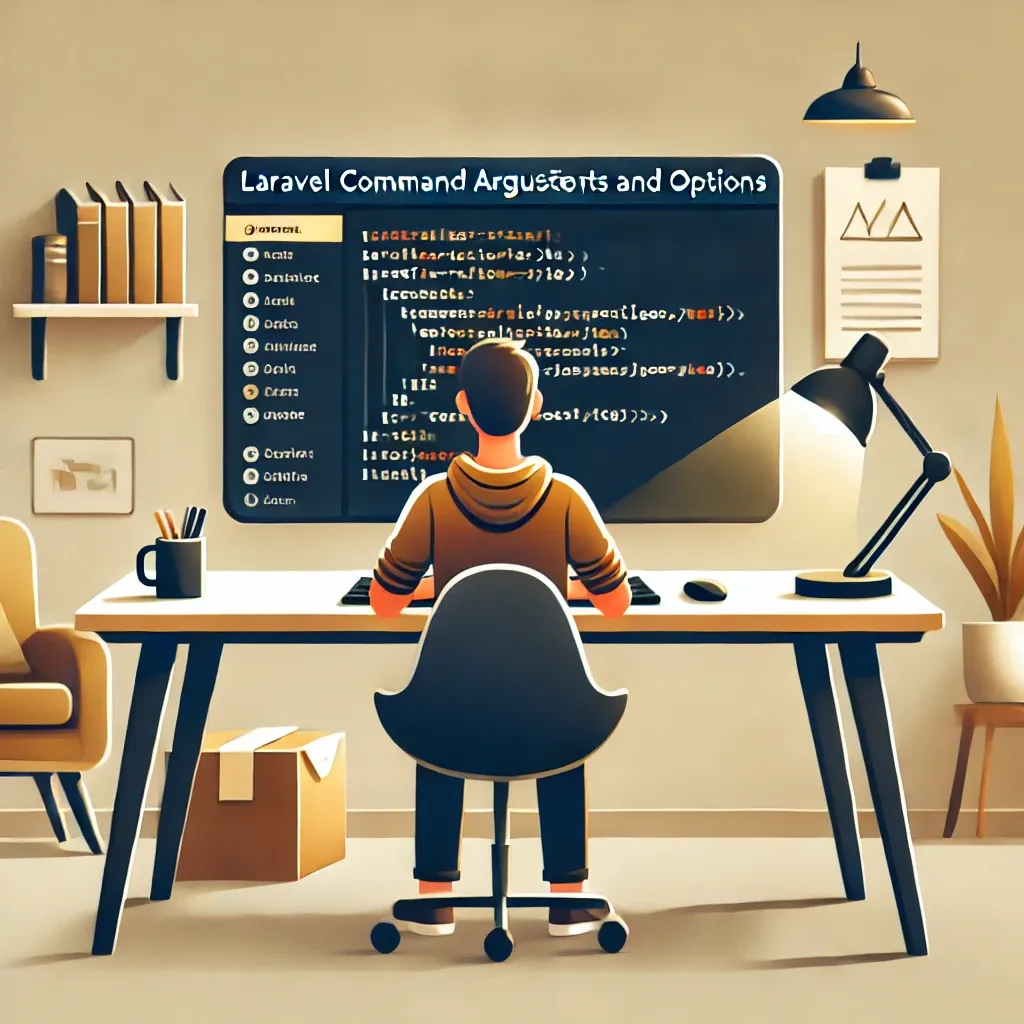
Need to work with command arguments and options in your Artisan commands? Laravel makes it super simple!
Basic Access Methods
Get single values with argument
and option
:
// Get a single argument
$userId = $this->argument('user');
// Get a single option
$queueName = $this->option('queue');
Getting All Values
Retrieve all arguments or options as arrays:
// Get all arguments
$arguments = $this->arguments();
// Get all options
$options = $this->options();
Real-World Example
Here's a practical deployment command demonstrating various ways to access inputs:
class DeployApplication extends Command
{
protected $signature = 'deploy
{environment : The deployment environment}
{--branch= : The git branch to deploy}
{--F|force : Force deployment without confirmations}
{--notify=* : Notification channels}';
public function handle()
{
// Individual access
$environment = $this->argument('environment');
$branch = $this->option('branch') ?? 'main';
$force = $this->option('force');
// Dump all inputs for debugging
if ($this->option('verbose')) {
$this->table(
['Type', 'Values'],
[
['Arguments', json_encode($this->arguments())],
['Options', json_encode($this->options())]
]
);
}
// Use the values
$this->info("Deploying to {$environment}");
$this->info("Branch: {$branch}");
if (!$force && !$this->confirm('Continue with deployment?')) {
return;
}
// Handle notifications
foreach ($this->option('notify') as $channel) {
$this->info("Sending notification via {$channel}");
}
// Deployment logic here...
}
}
Usage examples:
# Basic usage
php artisan deploy production --branch=main
# With multiple notification channels
php artisan deploy staging --branch=feature/new-api --notify=slack --notify=email
# Force deployment
php artisan deploy production -F
# Show all inputs (verbose mode)
php artisan deploy production -v
The ability to easily access command arguments and options helps you build more flexible and powerful console commands.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!