Streamlining API Responses: Converting Laravel Models to JSON
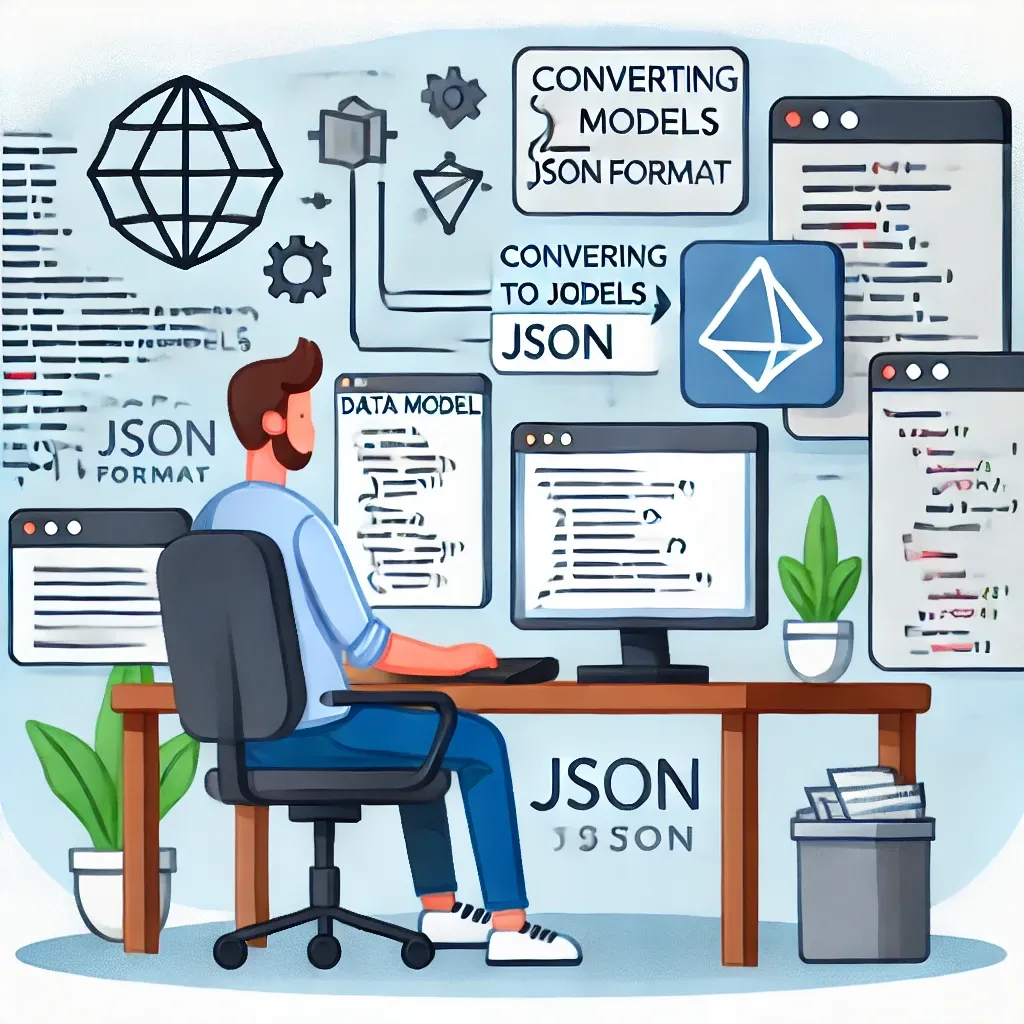
When building APIs with Laravel, converting your Eloquent models to JSON is a common task. Laravel provides several methods to accomplish this, making it easy to create consistent and flexible API responses. Let's explore how to effectively convert your models to JSON and leverage Laravel's automatic serialization features.
The toJson Method
The simplest way to convert a model to JSON is by using the toJson
method:
$user = User::find(1);
return $user->toJson();
This method converts the model to an array and then to JSON. You can also specify JSON encoding options:
return $user->toJson(JSON_PRETTY_PRINT);
Implicit Conversion
Laravel makes it even easier by automatically converting models and collections to JSON when they're returned from routes or controllers:
Route::get('/users', function () {
return User::all();
});
This route will return a JSON representation of all users without any additional code.
Customizing JSON Conversion
Sometimes you might want to customize how your model is converted to JSON. You can do this by overriding the toArray
method in your model:
class User extends Model
{
public function toArray()
{
return [
'id' => $this->id,
'name' => $this->name,
'email' => $this->email,
'is_admin' => $this->isAdmin(),
'created_at' => $this->created_at->toDateTimeString(),
];
}
}
Now, whenever this model is converted to JSON, it will use this custom array representation.
Handling Relationships
JSON conversion automatically includes loaded relationships:
$user = User::with('posts')->find(1);
return $user->toJson();
This will include the user's posts in the JSON output.
Hiding Attributes
For sensitive data, you might want to hide certain attributes when converting to JSON:
class User extends Model
{
protected $hidden = ['password', 'remember_token'];
}
These attributes will be excluded from the JSON representation.
Appending Custom Attributes
Conversely, you might want to add custom attributes to your JSON output:
class User extends Model
{
protected $appends = ['full_name'];
public function getFullNameAttribute()
{
return "{$this->first_name} {$this->last_name}";
}
}
The full_name
attribute will now be included in the JSON output.
Real-World Scenario: Building an API
Let's consider a scenario where you're building an API for a blog platform. You might have a Post
model with associated User
and Comment
models:
class Post extends Model
{
protected $appends = ['comment_count'];
public function user()
{
return $this->belongsTo(User::class);
}
public function comments()
{
return $this->hasMany(Comment::class);
}
public function getCommentCountAttribute()
{
return $this->comments()->count();
}
public function toArray()
{
return [
'id' => $this->id,
'title' => $this->title,
'content' => $this->content,
'author' => $this->user->name,
'comment_count' => $this->comment_count,
'created_at' => $this->created_at->toDateTimeString(),
'updated_at' => $this->updated_at->toDateTimeString(),
];
}
}
Now, in your controller:
class PostController extends Controller
{
public function index()
{
$posts = Post::with('user')->get();
return response()->json($posts);
}
public function show($id)
{
$post = Post::with(['user', 'comments.user'])->findOrFail($id);
return response()->json($post);
}
}
This setup allows you to easily return JSON representations of your posts, including related data, without repetitive coding in your controllers.
Laravel's built-in JSON conversion features provide a powerful and flexible way to create API responses. By leveraging these tools, you can easily transform your Eloquent models into JSON, customizing the output as needed while maintaining clean and efficient code.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!