Streamlined Data Access: Laravel's attributesToArray Method
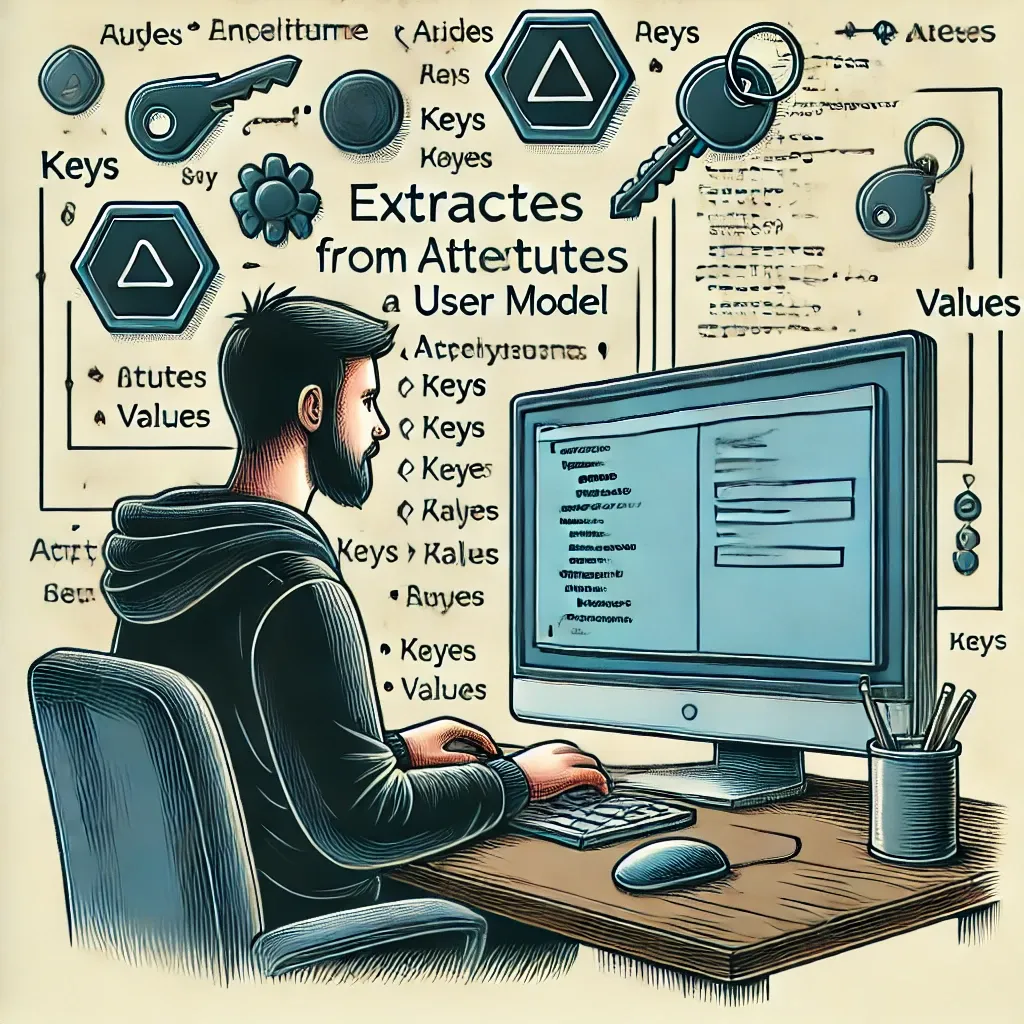
When working with Laravel's Eloquent models, there are times when you need to access the raw attribute data without the overhead of relationships or custom accessors. This is where the attributesToArray
method comes in handy. Let's explore how this method can simplify your data handling and improve performance in certain scenarios.
Understanding attributesToArray
The attributesToArray
method is a part of Laravel's Eloquent model. It converts a model's attributes to an array, excluding any loaded relationships or appended attributes. This method gives you a clean, lightweight representation of your model's core data.
Basic Usage
Here's a simple example of how to use attributesToArray
:
$user = User::first();
$attributes = $user->attributesToArray();
// Result: ['id' => 1, 'name' => 'John Doe', 'email' => 'john@example.com', ...]
This gives you an array of the model's attributes as they are stored in the database, without any additional computed properties or relationships.
Practical Applications
1. Lightweight API Responses
When you need to send a stripped-down version of your model data:
public function show($id)
{
$user = User::findOrFail($id);
return response()->json($user->attributesToArray());
}
This ensures that only the model's actual attributes are sent in the response, which can be useful for performance or security reasons.
2. Caching
When caching model data, you often want to store only the core attributes:
$cacheKey = 'user:' . $user->id;
Cache::put($cacheKey, $user->attributesToArray(), now()->addHours(24));
This approach prevents caching of volatile or computed data.
3. Comparison Operations
When you need to compare the actual stored values of two model instances:
$user1 = User::find(1);
$user2 = User::find(2);
$diff = array_diff($user1->attributesToArray(), $user2->attributesToArray());
This gives you a clear comparison of the raw attribute values.
Contrasting with toArray
It's important to understand the difference between attributesToArray
and toArray
:
attributesToArray
returns only the model's attributes.toArray
includes attributes, loaded relationships, and appended attributes.
Here's a comparison:
class User extends Model
{
protected $appends = ['full_name'];
public function posts()
{
return $this->hasMany(Post::class);
}
public function getFullNameAttribute()
{
return "{$this->first_name} {$this->last_name}";
}
}
$user = User::with('posts')->first();
$attributes = $user->attributesToArray();
$array = $user->toArray();
// $attributes will not include 'full_name' or 'posts'
// $array will include 'full_name' and 'posts'
Real-World Scenario: Audit Logging
Imagine you're building an audit system for your application. You want to log changes to models, but only for their core attributes, not computed properties or relationships. Here's how you might use attributesToArray
:
class AuditableModel extends Model
{
public static function boot()
{
parent::boot();
static::updated(function ($model) {
$original = $model->getOriginal();
$changes = array_diff($model->attributesToArray(), $original);
if (!empty($changes)) {
AuditLog::create([
'model_type' => get_class($model),
'model_id' => $model->id,
'changes' => json_encode($changes),
'user_id' => auth()->id(),
]);
}
});
}
}
class User extends AuditableModel
{
// User model implementation
}
In this example, attributesToArray
ensures that we're only logging changes to the actual database attributes, not any computed properties or relationship data.
The attributesToArray
method in Laravel provides a straightforward way to access the raw attribute data of your Eloquent models. By giving you direct access to the core data without the overhead of relationships or computed properties, it enables more efficient data handling in scenarios like API responses, caching, and auditing. Understanding when to use attributesToArray
versus toArray
can help you optimize your Laravel applications for both performance and functionality.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!