Efficient Model Creation: Recycling Models in Laravel Factories
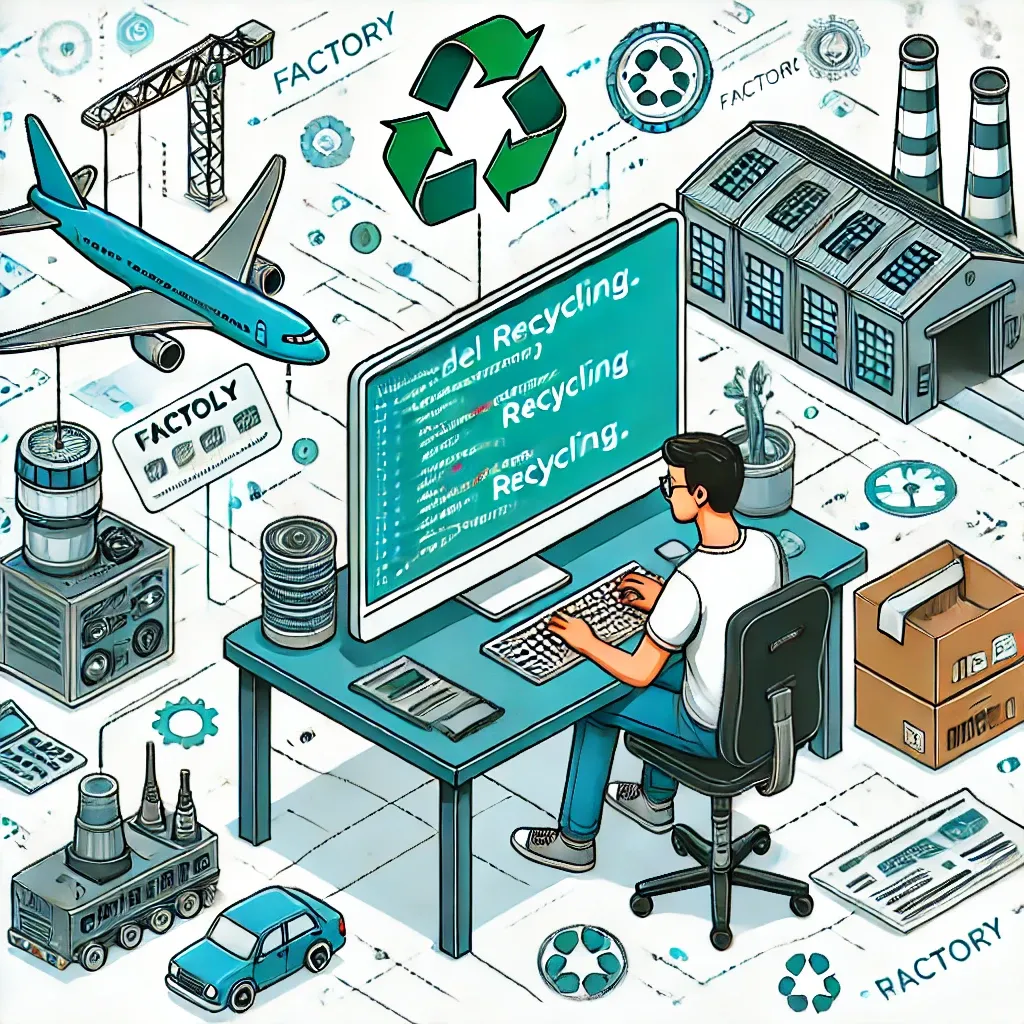
When working with Laravel factories, especially for complex data structures or relationships, you might find yourself needing to reuse certain model instances across multiple factory calls. Laravel provides a powerful feature for this exact scenario: the recycle
method. Let's dive into how this method can optimize your factory usage and improve the efficiency of your test data creation.
Understanding Model Recycling
The recycle
method allows you to reuse existing model instances across multiple factory calls. This is particularly useful when you have models that share common relationships, such as users belonging to the same company or posts belonging to the same category.
Basic Usage
Here's a simple example of how to use the recycle
method:
$airline = Airline::factory()->create();
$tickets = Ticket::factory()
->count(3)
->recycle($airline)
->create();
In this example, all three tickets will be associated with the same airline, without creating new airline instances for each ticket.
Advanced Usage
You can recycle multiple models and even collections of models:
$airline = Airline::factory()->create();
$flight = Flight::factory()->create();
$tickets = Ticket::factory()
->count(5)
->recycle([$airline, $flight])
->create();
This will reuse both the airline and flight for all created tickets.
Recycling Collections
When you have a collection of models to recycle, Laravel will randomly choose from the collection for each factory call:
$airlines = Airline::factory()->count(3)->create();
$tickets = Ticket::factory()
->count(10)
->recycle($airlines)
->create();
Each ticket will be associated with one of the three airlines, chosen randomly.
Practical Applications
1. Testing Complex Relationships
When testing scenarios with complex relationships, recycling can simplify your setup:
$company = Company::factory()->create();
$departments = Department::factory()->count(3)->recycle($company)->create();
$employees = Employee::factory()
->count(20)
->recycle($company)
->recycle($departments)
->create();
This ensures that all departments belong to the same company, and all employees belong to that company and are distributed among its departments.
2. Seeding Hierarchical Data
For seeding production or staging environments with hierarchical data:
$categories = Category::factory()->count(5)->create();
$products = Product::factory()
->count(100)
->recycle($categories)
->create();
This creates a varied but consistent product catalog structure.
3. Performance Optimization
Recycling can significantly improve performance when creating large datasets:
$users = User::factory()->count(1000)->create();
$posts = Post::factory()
->count(10000)
->recycle($users)
->create();
This approach is much more efficient than creating a new user for each post.
Real-World Scenario: E-commerce Platform
Let's consider a more complex scenario for an e-commerce platform:
// Create base data
$sellers = Seller::factory()->count(10)->create();
$categories = Category::factory()->count(20)->create();
$customers = Customer::factory()->count(1000)->create();
// Create products associated with sellers and categories
$products = Product::factory()
->count(500)
->recycle($sellers)
->recycle($categories)
->create();
// Create orders
$orders = Order::factory()
->count(2000)
->recycle($customers)
->create()
->each(function ($order) use ($products) {
// Add 1-5 products to each order
$orderProducts = $products->random(rand(1, 5));
$order->products()->attach($orderProducts, ['quantity' => rand(1, 3)]);
});
This setup creates a realistic e-commerce dataset with interconnected sellers, categories, products, customers, and orders, all while efficiently reusing model instances where appropriate.
The recycle
method in Laravel factories provides a powerful way to create complex, interconnected datasets efficiently. By allowing you to reuse model instances across multiple factory calls, it helps in creating more realistic test data, optimizing performance, and simplifying the setup of complex data structures. Whether you're writing tests, seeding databases, or generating demo data, mastering model recycling can significantly enhance your Laravel development workflow.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!